Complete-Stack Development
The process of designing, creating, and implementing a web application's client-side (or front-end) and server-side (or back-end) components is known as full-stack web development. Developers handle all aspects of this comprehensive method, including database management, server configuration, and user interface design and interaction.
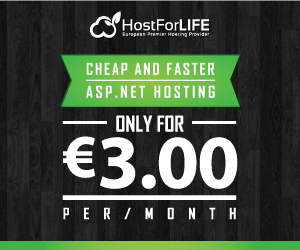
Components of a Full-Stack Web UI
Front-End Development
HTML, CSS, and JavaScript
Front-end development involves creating the visual elements and user experience of a website or web application. HTML (HyperText Markup Language), CSS (Cascading Style Sheets), and JavaScript form the core technologies used in front-end development. HTML provides the structure, CSS dictates the presentation and layout, and JavaScript adds interactivity and dynamic behavior to the user interface.
Front-End Frameworks and Libraries
To streamline development and enhance functionality, front-end developers often leverage frameworks and libraries such as React, Angular, Vue.js, and Bootstrap. These tools provide pre-built components, responsive design capabilities, and state management features, enabling developers to build interactive and visually appealing user interfaces more efficiently.
Back-End Development
Server-Side Programming Languages
Back-end development focuses on the server-side logic and functionality of a web application. Developers use programming languages like JavaScript (Node.js), Python (Django, Flask), Ruby (Ruby on Rails), Java (Spring Boot), and C# (ASP.NET Core) to implement server-side operations, data processing, and communication with databases.
Databases and Data Storage
Back-end developers work with databases to store, retrieve, and manipulate data required by the web application. Common types of databases include relational databases like MySQL, PostgreSQL, and SQL Server, as well as NoSQL databases like MongoDB and Firebase Firestore. Developers also utilize Object-Relational Mapping (ORM) libraries to simplify database interactions and manage data models.
APIs and Web Services
Application Programming Interfaces (APIs) play a crucial role in full-stack development by facilitating communication between the front-end and back-end components of a web application. Developers design and implement RESTful APIs or GraphQL endpoints to define how data is exchanged between the client and server. Web services, such as authentication services, payment gateways, and third-party integrations, are also integrated into the back-end architecture to extend the functionality of the web application.
DevOps and Deployment
Continuous Integration and Deployment (CI/CD)
DevOps practices are essential for streamlining the development, testing, and deployment processes of a full-stack web application. Developers utilize CI/CD pipelines to automate build, test, and deployment tasks, ensuring rapid and reliable delivery of updates and new features to production environments.
Cloud Platforms and Hosting Services
Full-stack developers deploy web applications on cloud platforms like Amazon Web Services (AWS), Microsoft Azure, and Google Cloud Platform (GCP), or deploy them to managed hosting services such as Heroku, Netlify, or Vercel. These platforms provide scalable infrastructure, serverless computing, and management tools that simplify deployment, monitoring, and maintenance tasks.
Introduction to ASP.NET Core 8 MVC
ASP.NET Core 8 MVC (Model-View-Controller) is a powerful framework for building modern, scalable, and feature-rich web applications. With its robust architecture, flexible programming model, and extensive tooling support, ASP.NET Core 8 MVC empowers developers to create full-stack web applications that meet the demands of today's digital landscape.
Components of ASP.NET Core 8 MVC
Model
In ASP.NET Core 8 MVC, the model represents the data and business logic of the application. Developers define model classes to encapsulate data entities, define relationships, and implement business rules. Models interact with the database through Entity Framework Core or other data access libraries, enabling seamless data manipulation and persistence.
View
Views in ASP.NET Core 8 MVC are responsible for presenting the user interface and rendering dynamic content to the client. Developers create view templates using Razor syntax, which combines HTML markup with C# code to generate dynamic web pages. Views leverage model data to display information to users and incorporate client-side scripting languages like JavaScript for enhanced interactivity.
Controller
Controllers act as intermediaries between the model and view components in ASP.NET Core 8 MVC. They handle user requests, execute business logic, and orchestrate data retrieval and manipulation operations. Controllers receive input from the user through the browser, process it, and return an appropriate response, such as rendering a view or redirecting to another page.
Full Stack Development in ASP.NET Core 8 MVC
Front-End Development
HTML, CSS, and JavaScript
Front-end development in ASP.NET Core 8 MVC involves creating responsive and visually appealing user interfaces using HTML, CSS, and JavaScript. Developers leverage HTML for structure, CSS for styling, and JavaScript for client-side interactivity and dynamic behavior.
Razor Pages and Views
ASP.NET Core 8 MVC utilizes Razor Pages and Views to generate dynamic web content. Developers use Razor syntax within HTML markup to incorporate server-side logic, access model data, and render dynamic content. Razor Pages provide a streamlined approach to building web pages, while Views offer more flexibility and customization options.
Back-End Development
C# Programming Language
Back-end development in ASP.NET Core 8 MVC revolves around the C# programming language, which serves as the primary language for implementing server-side logic, business rules, and data access operations. C# offers a robust and type-safe development environment, enabling developers to build scalable and maintainable back-end components.
Entity Framework Core
Entity Framework Core is a powerful Object-Relational Mapping (ORM) framework that simplifies data access and manipulation in ASP.NET Core 8 MVC applications. Developers use Entity Framework Core to define data models, perform database migrations, execute LINQ queries, and interact with the underlying database seamlessly.
ASP.NET Core Identity
ASP.NET Core Identity provides robust authentication and authorization features for securing ASP.NET Core 8 MVC applications. Developers leverage ASP.NET Core Identity to implement user authentication, role-based access control, password hashing, and account management functionalities, ensuring the security and integrity of web applications.
Deployment and DevOps
Continuous Integration and Deployment (CI/CD)
DevOps practices play a crucial role in the deployment and maintenance of ASP.NET Core 8 MVC applications. Developers implement CI/CD pipelines to automate build, test, and deployment processes, ensuring rapid and reliable delivery of updates to production environments. Tools like Azure DevOps, GitHub Actions, and Jenkins streamline the CI/CD workflow, enabling efficient collaboration and deployment.
Nuget Packages for this application
Microsoft.AspNetCore.Mvc
Microsoft.EntityFrameworkCore
Microsoft.AspNetCore.Identity.EntityFrameworkCore
Microsoft.Extensions.Logging
Microsoft.Extensions.Configuration
Microsoft.AspNetCore.Authentication.Cookies
Microsoft.Extensions.DependencyInjection
Newtonsoft.Json
Microsoft.AspNetCore.StaticFiles
Microsoft.AspNetCore.Diagnostics.EntityFrameworkCore
SQLite Database
We will use SQL Lite database for this application
Nuget Package for SQL Lite Database
Microsoft.EntityFrameworkCore.Sqlite
Student Model
namespace FullStackDevelopmentUsingAspnetCore8MVC.Models
{
public class Student
{
public int Id { get; set; }
public string Name { get; set; }
public string RollNo { get; set; }
public string Section { get; set; }
public string Program { get; set; }
}
}
Application Database Context
using FullStackDevelopmentUsingAspnetCore8MVC.Models;
using Microsoft.EntityFrameworkCore;
namespace FullStackDevelopmentUsingAspnetCore8MVC.ApplicationDbContext
{
public class AppDbContext : DbContext
{
public AppDbContext(DbContextOptions<AppDbContext> options) : base(options) { }
public DbSet<Student> Students { get; set; }
}
}
Application Setting File
// appsettings.json
{
"ConnectionStrings": {
"DefaultConnection": "Data Source=FullStackDevlopment.db"
},
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft": "Warning",
"Microsoft.Hosting.Lifetime": "Information"
}
},
"AllowedHosts": "*"
}
Program.cs Class
using FullStackDevelopmentUsingAspnetCore8MVC.ApplicationDbContext;
using Microsoft.EntityFrameworkCore;
var builder = WebApplication.CreateBuilder(args);
var configuration = builder.Configuration;
// Add services to the container.
builder.Services.AddDbContext<AppDbContext>(options =>
options.UseSqlite(configuration.GetConnectionString("DefaultConnection")));
builder.Services.AddRazorPages();
var app = builder.Build();
// Configure the HTTP request pipeline.
if(!app.Environment.IsDevelopment())
{
app.UseExceptionHandler("/Error");
// The default HSTS value is 30 days. You may want to change this for production scenarios, see https://aka.ms/aspnetcore-hsts.
app.UseHsts();
}
app.UseAuthorization();
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseRouting();
app.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
app.MapRazorPages();
app.Run();
Student Controller
using FullStackDevelopmentUsingAspnetCore8MVC.ApplicationDbContext;
using FullStackDevelopmentUsingAspnetCore8MVC.Models;
using Microsoft.AspNetCore.Mvc;
using Microsoft.EntityFrameworkCore;
namespace FullStackDevelopmentUsingAspnetCore8MVC.Controllers
{
public class StudentsController : Controller
{
private readonly AppDbContext _context;
public StudentsController(AppDbContext context)
{
_context = context;
}
// GET: Students
public async Task<IActionResult> Index()
{
return View(await _context.Students.ToListAsync());
}
// GET: Students/Details/5
public async Task<IActionResult> Details(int? id)
{
if(id == null)
{
return NotFound();
}
var student = await _context.Students
.FirstOrDefaultAsync(m => m.Id == id);
if(student == null)
{
return NotFound();
}
return View(student);
}
// GET: Students/Create
public IActionResult Create()
{
return View();
}
// POST: Students/Create
// To protect from overposting attacks, enable the specific properties you want to bind to.
// For more details, see http://go.microsoft.com/fwlink/?LinkId=317598.
[HttpPost]
[ValidateAntiForgeryToken]
public async Task<IActionResult> Create([Bind("Id,Name,RollNo,Section,Program")] Student student)
{
if(ModelState.IsValid)
{
_context.Add(student);
await _context.SaveChangesAsync();
return RedirectToAction(nameof(Index));
}
return View(student);
}
// GET: Students/Edit/5
public async Task<IActionResult> Edit(int? id)
{
if(id == null)
{
return NotFound();
}
var student = await _context.Students.FindAsync(id);
if(student == null)
{
return NotFound();
}
return View(student);
}
// POST: Students/Edit/5
// To protect from overposting attacks, enable the specific properties you want to bind to.
// For more details, see http://go.microsoft.com/fwlink/?LinkId=317598.
[HttpPost]
[ValidateAntiForgeryToken]
public async Task<IActionResult> Edit(int id, [Bind("Id,Name,RollNo,Section,Program")] Student student)
{
if(id != student.Id)
{
return NotFound();
}
if(ModelState.IsValid)
{
try
{
_context.Update(student);
await _context.SaveChangesAsync();
}
catch(DbUpdateConcurrencyException)
{
if(!StudentExists(student.Id))
{
return NotFound();
}
else
{
throw;
}
}
return RedirectToAction(nameof(Index));
}
return View(student);
}
// GET: Students/Delete/5
public async Task<IActionResult> Delete(int? id)
{
if(id == null)
{
return NotFound();
}
var student = await _context.Students
.FirstOrDefaultAsync(m => m.Id == id);
if(student == null)
{
return NotFound();
}
return View(student);
}
// POST: Students/Delete/5
[HttpPost, ActionName("Delete")]
[ValidateAntiForgeryToken]
public async Task<IActionResult> DeleteConfirmed(int id)
{
var student = await _context.Students.FindAsync(id);
if(student != null)
{
_context.Students.Remove(student);
}
await _context.SaveChangesAsync();
return RedirectToAction(nameof(Index));
}
private bool StudentExists(int id)
{
return _context.Students.Any(e => e.Id == id);
}
}
}
Front-End Development Using CSHTML Pages
INDEX Page For showing Details
@model IEnumerable<FullStackDevelopmentUsingAspnetCore8MVC.Models.Student>
@{
ViewData["Title"] = "Index";
Layout = "~/Pages/Shared/_Layout.cshtml";
}
<h1>Index</h1>
<p>
<a asp-action="Create">Create New</a>
</p>
<table class="table">
<thead>
<tr>
<th>
@Html.DisplayNameFor(model => model.Name)
</th>
<th>
@Html.DisplayNameFor(model => model.RollNo)
</th>
<th>
@Html.DisplayNameFor(model => model.Section)
</th>
<th>
@Html.DisplayNameFor(model => model.Program)
</th>
<th></th>
</tr>
</thead>
<tbody>
@foreach (var item in Model) {
<tr>
<td>
@Html.DisplayFor(modelItem => item.Name)
</td>
<td>
@Html.DisplayFor(modelItem => item.RollNo)
</td>
<td>
@Html.DisplayFor(modelItem => item.Section)
</td>
<td>
@Html.DisplayFor(modelItem => item.Program)
</td>
<td>
<a asp-action="Edit" asp-route-id="@item.Id">Edit</a> |
<a asp-action="Details" asp-route-id="@item.Id">Details</a> |
<a asp-action="Delete" asp-route-id="@item.Id">Delete</a>
</td>
</tr>
}
</tbody>
</table>
CREATE Page for creating student
@model FullStackDevelopmentUsingAspnetCore8MVC.Models.Student
@{
ViewData["Title"] = "Create";
Layout = "~/Pages/Shared/_Layout.cshtml";
}
<h1>Create</h1>
<h4>Student</h4>
<hr />
<div class="row">
<div class="col-md-4">
<form asp-action="Create">
<div asp-validation-summary="ModelOnly" class="text-danger"></div>
<div class="form-group">
<label asp-for="Name" class="control-label"></label>
<input asp-for="Name" class="form-control" />
<span asp-validation-for="Name" class="text-danger"></span>
</div>
<div class="form-group">
<label asp-for="RollNo" class="control-label"></label>
<input asp-for="RollNo" class="form-control" />
<span asp-validation-for="RollNo" class="text-danger"></span>
</div>
<div class="form-group">
<label asp-for="Section" class="control-label"></label>
<input asp-for="Section" class="form-control" />
<span asp-validation-for="Section" class="text-danger"></span>
</div>
<div class="form-group">
<label asp-for="Program" class="control-label"></label>
<input asp-for="Program" class="form-control" />
<span asp-validation-for="Program" class="text-danger"></span>
</div>
<div class="form-group">
<input type="submit" value="Create" class="btn btn-primary" />
</div>
</form>
</div>
</div>
<div>
<a asp-action="Index">Back to List</a>
</div>
@section Scripts {
@{await Html.RenderPartialAsync("_ValidationScriptsPartial");}
}
DELETE Page for Deleting Student
@model FullStackDevelopmentUsingAspnetCore8MVC.Models.Student
@{
ViewData["Title"] = "Delete";
Layout = "~/Pages/Shared/_Layout.cshtml";
}
<h1>Delete</h1>
<h3>Are you sure you want to delete this?</h3>
<div>
<h4>Student</h4>
<hr />
<dl class="row">
<dt class = "col-sm-2">
@Html.DisplayNameFor(model => model.Name)
</dt>
<dd class = "col-sm-10">
@Html.DisplayFor(model => model.Name)
</dd>
<dt class = "col-sm-2">
@Html.DisplayNameFor(model => model.RollNo)
</dt>
<dd class = "col-sm-10">
@Html.DisplayFor(model => model.RollNo)
</dd>
<dt class = "col-sm-2">
@Html.DisplayNameFor(model => model.Section)
</dt>
<dd class = "col-sm-10">
@Html.DisplayFor(model => model.Section)
</dd>
<dt class = "col-sm-2">
@Html.DisplayNameFor(model => model.Program)
</dt>
<dd class = "col-sm-10">
@Html.DisplayFor(model => model.Program)
</dd>
</dl>
<form asp-action="Delete">
<input type="hidden" asp-for="Id" />
<input type="submit" value="Delete" class="btn btn-danger" /> |
<a asp-action="Index">Back to List</a>
</form>
</div>
DETAILS Page for showing individual Student Details
@model FullStackDevelopmentUsingAspnetCore8MVC.Models.Student
@{
ViewData["Title"] = "Details";
Layout = "~/Pages/Shared/_Layout.cshtml";
}
<h1>Details</h1>
<div>
<h4>Student</h4>
<hr />
<dl class="row">
<dt class = "col-sm-2">
@Html.DisplayNameFor(model => model.Name)
</dt>
<dd class = "col-sm-10">
@Html.DisplayFor(model => model.Name)
</dd>
<dt class = "col-sm-2">
@Html.DisplayNameFor(model => model.RollNo)
</dt>
<dd class = "col-sm-10">
@Html.DisplayFor(model => model.RollNo)
</dd>
<dt class = "col-sm-2">
@Html.DisplayNameFor(model => model.Section)
</dt>
<dd class = "col-sm-10">
@Html.DisplayFor(model => model.Section)
</dd>
<dt class = "col-sm-2">
@Html.DisplayNameFor(model => model.Program)
</dt>
<dd class = "col-sm-10">
@Html.DisplayFor(model => model.Program)
</dd>
</dl>
</div>
<div>
<a asp-action="Edit" asp-route-id="@Model?.Id">Edit</a> |
<a asp-action="Index">Back to List</a>
</div>
Edit Page for Editing the Student Details
@model FullStackDevelopmentUsingAspnetCore8MVC.Models.Student
@{
ViewData["Title"] = "Edit";
Layout = "~/Pages/Shared/_Layout.cshtml";
}
<h1>Edit</h1>
<h4>Student</h4>
<hr />
<div class="row">
<div class="col-md-4">
<form asp-action="Edit">
<div asp-validation-summary="ModelOnly" class="text-danger"></div>
<input type="hidden" asp-for="Id" />
<div class="form-group">
<label asp-for="Name" class="control-label"></label>
<input asp-for="Name" class="form-control" />
<span asp-validation-for="Name" class="text-danger"></span>
</div>
<div class="form-group">
<label asp-for="RollNo" class="control-label"></label>
<input asp-for="RollNo" class="form-control" />
<span asp-validation-for="RollNo" class="text-danger"></span>
</div>
<div class="form-group">
<label asp-for="Section" class="control-label"></label>
<input asp-for="Section" class="form-control" />
<span asp-validation-for="Section" class="text-danger"></span>
</div>
<div class="form-group">
<label asp-for="Program" class="control-label"></label>
<input asp-for="Program" class="form-control" />
<span asp-validation-for="Program" class="text-danger"></span>
</div>
<div class="form-group">
<input type="submit" value="Save" class="btn btn-primary" />
</div>
</form>
</div>
</div>
<div>
<a asp-action="Index">Back to List</a>
</div>
@section Scripts {
@{await Html.RenderPartialAsync("_ValidationScriptsPartial");}
}
Conclusion
ASP.NET Core 8 MVC empowers developers to build full-stack web applications with unparalleled flexibility, scalability, and performance. By mastering the components of ASP.NET Core 8 MVC, including models, views, controllers, front-end and back-end development tools, and deployment practices, developers can create robust and feature-rich web applications that meet the evolving needs of users and businesses.