Today I am going to explain how to create Google Maps Sample App with AngularJS and asp.net MVC. In one of the previous article, we have seen How to display google map in asp.net application andHow to load GMap Direction from database in ASP.NET using google map.
In order to use the Google Maps in our application, we need to add the following resources:
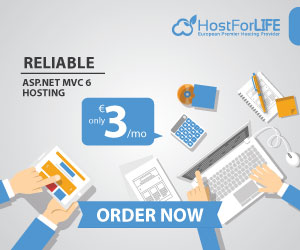
- angular.js
- lodash.js - Loadash is a dependency of angular-google-maps library.
- angular-google-maps.js - Angular Google Maps is a set of directives which integrate Google Maps in an AngularJS applications.
Just follow the following steps in order to create a sample google app with AngularJS and asp.net MVC:
Step - 1: Create New Project.
Go to File > New > Project > Select asp.net MVC4 web application > Entry Application Name > Click OK > Select Basic > Select view engine Razor > OK
Step-2: Add a Database.
Go to Solution Explorer > Right Click on App_Data folder > Add > New item > Select SQL Server Database Under Data > Enter Database name > Add. Here I have added a database for store some location information in our database for show in the google map.
Step-3: Create a table.
Here I will create 1 table (as below) for store location information. Open Database > Right Click on Table > Add New Table > Add Columns > Save > Enter table name > Ok.
Step-4: Add Entity Data Model.
Go to Solution Explorer > Right Click on Project name form Solution Explorer > Add > New item > Select ADO.net Entity Data Model under data > Enter model name > Add. A popup window will come (Entity Data Model Wizard) > Select Generate from database > Next > Chose your data connection > select your database > next > Select tables > enter Model Namespace > Finish.
Step-5: Create a Controller.
Go to Solution Explorer > Right Click on Controllers folder form Solution Explorer > Add > Controller > Enter Controller name > Select Templete "empty MVC Controller"> Add. Here I have created a controller "HomeController"
Step-6: Add new action into the controller to get the view where we will show google map
Here I have added "Index" Action into "Home" Controller. Please write this following code
public ActionResult Index()
{
return View();
}
Step-7: Add another action (here "GetAllLocation") for fetch all the location from the database.
public JsonResult GetAllLocation()
{
using (MyDatabaseEntities dc = new MyDatabaseEntities())
{
var v = dc.Locations.OrderBy(a => a.Title).ToList();
return new JsonResult { Data = v, JsonRequestBehavior = JsonRequestBehavior.AllowGet };
}
}
Step-8: Add 1 more action (here "GetMarkerInfo") for getting google marker information from the database to show in the map.
public JsonResult GetMarkerInfo(int locationID)
{ using (MyDatabaseEntities dc = new MyDatabaseEntities())
{ Location l = null;
l = dc.Locations.Where(a => a.LocationID.Equals(locationID)).FirstOrDefault();
return new JsonResult { Data = l, JsonRequestBehavior = JsonRequestBehavior.AllowGet };
}
}
Step-9: Add a new javascript file, will contain all the necessary logic to implement our Google Map.
Right Click on Action Method (here right click on Index action) > Add View... > Enter View Name > Select View Engine (Razor) > Add.
var app = angular.module('myApp', ['uiGmapgoogle-maps']);
app.controller('mapController', function ($scope, $http) {
//this is for default map focus when load first time
$scope.map = { center: { latitude: 22.590406, longitude: 88.366034 }, zoom: 16 }
$scope.markers = [];
$scope.locations = [];
//Populate all location
$http.get('/home/GetAllLocation').then(function (data) {
$scope.locations = data.data;
}, function () {
alert('Error');
});
//get marker info
$scope.ShowLocation = function (locationID) {
$http.get('/home/GetMarkerInfo', {
params: {
locationID: locationID
}
}).then(function (data) {
//clear markers
$scope.markers = [];
$scope.markers.push({
id: data.data.LocationID,
coords: { latitude: data.data.Lat, longitude: data.data.Long },
title: data.data.title,
address: data.data.Address,
image : data.data.ImagePath
});
//set map focus to center
$scope.map.center.latitude = data.data.Lat;
$scope.map.center.longitude = data.data.Long;
}, function () {
alert('Error');
});
}
//Show / Hide marker on map
$scope.windowOptions = {
show: true
};
});
Step-10: Add view for the action (here "Index") & design.
Right Click on Action Method (here right click on Index action) > Add View... > Enter View Name > Select View Engine (Razor) > Add. HTML Code
@{ ViewBag.Title = "Index";
}
<h2>Index</h2>
<div ng-app="myApp" ng-controller="mapController">
<div class="locations">
<ul>
<li ng-repeat="l in locations" ng-click="ShowLocation(l.LocationID)">{{l.Title}}</li>
</ul>
</div>
<div class="maps">
<!-- Add directive code (gmap directive) for show map and markers-->
<ui-gmap-google-map center="map.center" zoom="map.zoom">
<ui-gmap-marker ng-repeat="marker in markers" coords="marker.coords" options="marker.options" events="marker.events" idkey="marker.id">
<ui-gmap-window options="windowOptions" show="windowOptions.show">
<div style="max-width:200px">
<div class="header"><strong>{{marker.title}}</strong></div>
<div id="mapcontent">
<p>
<img ng-src="{{marker.image}}" style="width:200px; height:100px" />
<div>{{marker.address}}</div>
</p>
</div>
</div>
</ui-gmap-window>
</ui-gmap-marker>
</ui-gmap-google-map>
</div>
</div>
@* Now here we need to some css and js *@
<style>
.angular-google-map-container {
height:300px;
}
.angular-google-map {
width:80%;
height:100%;
margin:auto 0px;
}
.locations {
width: 200px;
float: left;
}
.locations ul {
padding: 0px;
margin: 0px;
margin-right: 20px;
}
.locations ul li {
list-style-type: none;
padding: 5px;
border-bottom: 1px solid #f3f3f3;
cursor: pointer;
}
</style>
@section Scripts{
@* AngularJS library *@
<script src="//cdnjs.cloudflare.com/ajax/libs/angular.js/1.4.6/angular.js"></script>
@* google map directive js *@
<script src="//cdnjs.cloudflare.com/ajax/libs/lodash.js/2.4.1/lodash.js"></script>
<script src="//rawgit.com/angular-ui/angular-google-maps/2.0.X/dist/angular-google-maps.js"></script>
@* here We will add our created js file *@
<script src="~/Scripts/ngMap.js"></script>
}
Step-11: Run Application.
HostForLIFE.eu ASP.NET MVC 6 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
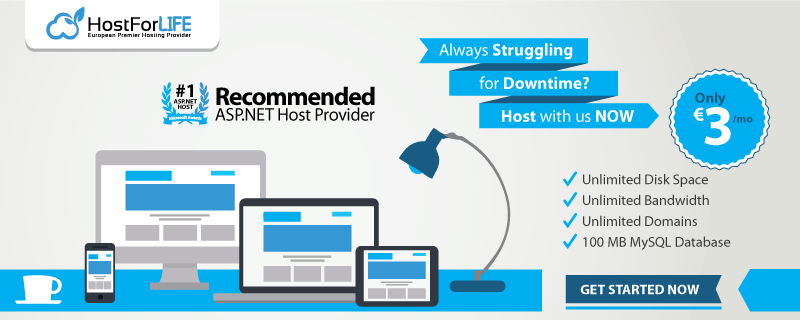