
December 15, 2023 06:00 by
Peter
CORS: What is it?
CORS is a security feature of browsers that regulates the sharing of resources, such as images or data, across websites from different domains (e.g., example.com vs. api.example.com). In order to stop unwanted cross-domain queries, it enables servers to whitelist particular domains for resource access. Consider it a data passport that guarantees secure connections between websites.
Because browsers save a ton of personal information, such as cookies, for every website, CORS issues can be a pain. An unscrupulous website has the ability to take over your browser session and maybe steal sensitive data from reliable websites if CORS limitations are not properly implemented.
Set your server to accept requests from the origin of your front end to resolve CORS difficulties in Next.js and React.
We will set up our asp.net web API to do this in this tutorial.
Step 1: Create an attribute to handle and preprocess the request.
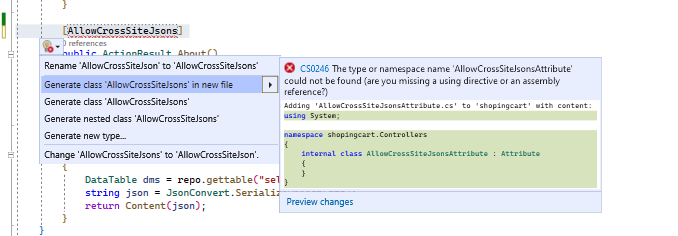
In the Generated Class File, add this code snippet.
public class AllowCrossSiteJsonAttribute : ActionFilterAttribute
{
public override void OnActionExecuting(ActionExecutingContext filterContext)
{
filterContext.RequestContext.HttpContext.Response.AddHeader("Access-Control-Allow-Origin", "*");
base.OnActionExecuting(filterContext);
}
}
Step 2. Bind with Action
[AllowCrossSiteJson]
public ActionResult About()
{
DataTable dms = repo.getpro();
string json = JsonConvert.SerializeObject(dms);
return Content(json);
}
We've tackled the notorious CORS beast in this article, explaining its complexities and giving you the tools to set up your ASP.NET MVC 5 Web API so that it works flawlessly with JavaScript frameworks like ReactJS. You've made it possible for secure, seamless communication between your dynamic client-side apps and your server-side API by putting these tips into practice.
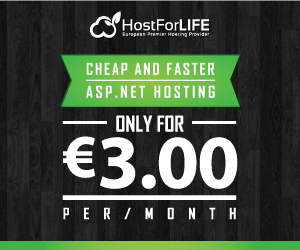
Recall the main conclusions.
- Setting the right headers to allow cross-origin requests from the server side is known as "configuring access-control headers."
Keep in mind that security is crucial. As alluring as "AllowAnyOrigin" may be, think about customizing your CORS setup to meet your unique requirements so that only allowed origins have access to your sensitive information.
We really hope that this post has helped you in your CORS journey. Please feel free to post a remark below if you have any queries or difficulties, and our community will be pleased to help!