One of the items I continuously forgot to add to my web applications is the Robots.txt file that Search Engines use to see what they should index. This file and site maps help make your site easier to navigate by the bots and allow them to know what's legal and what you would rather not have the published in their engines. I usually add any administrative pages or account pages despite the fact that they're protected by security, no need for the login page to be index if they sniff the link.
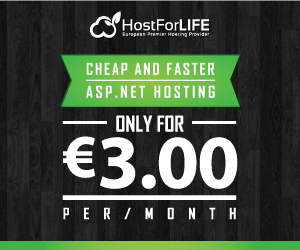
So how do you add Robots.txt to your MVC three application? Glad you asked, here may be a very little code to get you started.
Code
1. Choose the controller you'd wish to use for the robots.txt output. I selected the HomeController in my application as i use it for many “top level” generic links like about us, contact us, index, etc.
2. Create a method called Robots to handle the request.
#region -- Robots() Method –
public ActionResult Robots()
{
Response.ContentType = "text/plain";
return View();
}
#endregion
Add the Robots.cshtml view to your Controller’s View directory. Here is the code I have in my view, yours will vary.
@{
Layout = null;
}
# robots.txt for @this.Request.Url.Host
User-agent: *
Disallow: /Administration/
Disallow: /Account/
Load up the class you are using to control your routes, if you are in an Area, this could your AreaRegistration class. If you are at the top like I am and using the standard MVC template, this is probably the Global.asax.cs file. Add your route to this file, mine looks like this.
routes.MapRoute("Robots.txt",
"robots.txt",
new { controller = "Home", action = "Robots" });
Now, Compile and test.
If you have an internet facing site, the chances are you will have a bot find you're request this page. you might as well offer them the advantage of the doubt and allow them to know where you want them to travel. additionally you may save yourself some error log once this page is requested and no controller is found.
Just like something in ASP.NET, there are some ways to solve this riddle, if you employ a special approach, please feel free to share it within the comments.
HostForLIFE.eu ASP.NET MVC 6 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
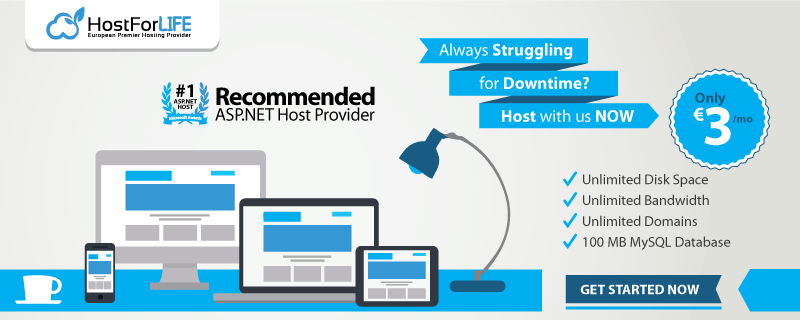