This article demonstrates how to create charts in ASP.NET MVC using Chart.js and C#. This article starts with how to use Chart.js in your MVC project. After that, it demonstrates how to add charts to a View.
Using Chart.js in your ASP.NET MVC project (C#)
Chart.js is a JavaScript charting tool for web developers. The latest version can be downloaded from GitHub or can use CDN.
In this article, Chart.js CDN (v2.6.0) is used for demonstration purposes.
<script src="https://cdnjs.cloudflare.com/ajax/libs/Chart.js/2.6.0/Chart.min.js" type="text/javascript"></script>
In the View (*.cshtml), add the Chart.js CDN along with jQuery CDN (recommended) in the head section if you haven’t mentioned those in layout pages.
@section head
{
<script src="https://cdnjs.cloudflare.com/ajax/libs/Chart.js/2.6.0/Chart.min.js" type="text/javascript"></script>
<script src="https://code.jquery.com/jquery-1.11.3.min.js"></script>
}
Adding chart to a View
In the following example, the <canvas> tag is used to hold the chart in View’s <body> section.
<div Style="font-family: Corbel; font-size: small ;text-align:center " class="row">
<div style="width:100%;height:100%">
<canvas id="myChart" style="padding: 0;margin: auto;display: block; "> </canvas>
</div>
</div>
Now, in the Controller class, let’s add a method to return the data for the chart that we added in the View. In this example, we are using JSON format for the source data.
[HttpPost]
public JsonResult NewChart()
{
List<object> iData = new List<object>();
//Creating sample data
DataTable dt = new DataTable() ;
dt.Columns.Add("Employee",System.Type.GetType("System.String"));
dt.Columns.Add("Credit",System.Type.GetType("System.Int32"));
DataRow dr = dt.NewRow();
dr["Employee"] = "Sam";
dr["Credit"] = 123;
dt.Rows.Add(dr);
dr = dt.NewRow();
dr["Employee"] = "Alex";
dr["Credit"] = 456;
dt.Rows.Add(dr);
dr = dt.NewRow();
dr["Employee"] = "Michael";
dr["Credit"] = 587;
dt.Rows.Add(dr);
//Looping and extracting each DataColumn to List<Object>
foreach (DataColumn dc in dt.Columns)
{
List<object> x = new List<object>();
x = (from DataRow drr in dt.Rows select drr[dc.ColumnName]).ToList();
iData.Add(x);
}
//Source data returned as JSON
return Json(iData, JsonRequestBehavior.AllowGet);
}
The data from the source table is processed in such a way that each column in the result table is made to separate list. The first column is expected to have the X-axis data of the chart, whereas the consequent columns hold the data for Y-axis. (Chart.js expects the Axis labels in separate list. Please check the AJAX call section.)
The data for axises is combined to a single List<Object>, and returned from the method as JSON.
AJAX calls are used in the <script> section of View to call the method in Controller to get the chart data.
<script>
$.ajax({
type: "POST",
url: "/Chart/NewChart",
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function (chData) {
var aData = chData;
var aLabels = aData[0];
var aDatasets1 = aData[1];
var dataT = {
labels: aLabels,
datasets: [{
label: "Test Data",
data: aDatasets1,
fill: false,
backgroundColor: ["rgba(54, 162, 235, 0.2)", "rgba(255, 99, 132, 0.2)", "rgba(255, 159, 64, 0.2)", "rgba(255, 205, 86, 0.2)", "rgba(75, 192, 192, 0.2)", "rgba(153, 102, 255, 0.2)", "rgba(201, 203, 207, 0.2)"],
borderColor: ["rgb(54, 162, 235)", "rgb(255, 99, 132)", "rgb(255, 159, 64)", "rgb(255, 205, 86)", "rgb(75, 192, 192)", "rgb(153, 102, 255)", "rgb(201, 203, 207)"],
borderWidth: 1
}]
};
var ctx = $("#myChart").get(0).getContext("2d");
var myNewChart = new Chart(ctx, {
type: 'bar',
data: dataT,
options: {
responsive: true,
title: { display: true, text: 'CHART.JS DEMO CHART' },
legend: { position: 'bottom' },
scales: {
xAxes: [{ gridLines: { display: false }, display: true, scaleLabel: { display: false, labelString: '' } }],
yAxes: [{ gridLines: { display: false }, display: true, scaleLabel: { display: false, labelString: '' }, ticks: { stepSize: 50, beginAtZero: true } }]
},
}
});
}
});
</script>
aData[0] has the data for X-Axis labels and aData[1] has the data for Y-Axis correspondingly.
As in the code, the AJAX call is made to the Controller method ’/Chart/NewChart’ where ‘Chart’ is the name of the Controller class and ‘NewChart’ is the method which returns the source data for the chart in JSON format.
AJAX call, when returned successfully, processes the returned JSON data.
The JSON data is processed to extract the labels and axis data for the chart preparation. The 2D context of the canvas ‘myChart’ is created using ‘getContext("2d")’ method, and then the context is used to create the chart object in ‘new Chart()’ method inside the script.
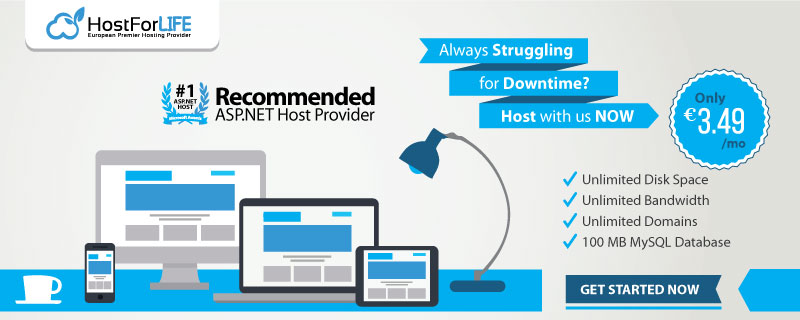