In this article, I will integrate and setup ELMAH to asp.net MVC project. I will finish whole article in 5 different steps. ELMAH stands for Error Logging Modules and Handlers providing application wide error logging facilities. ELMAH is pluggable and easy to implement without changing single line of code. ELMAH work as interceptor of unhandled dotnet exceptions, that display over yellow screen of death. As per Author you can dynamically add ELMAH on running asp.net application without recompile or re-deploy whole application.You can download ELMAH binaries from google code or if you are using nuget then visit ELMAH nuget page.
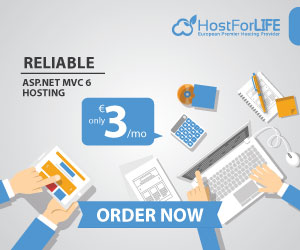
Install
The best way to install any module to Asp.net MVC project is to use Nuget package Console. You can visit ELMAH nuget page for get latest version command.
Configure
After installing ELMAH , it will automatically update Web.Config file. If it's not so you can add following code to Web.Config file.
<configuration>
<configSections>
<sectionGroup name="elmah">
<section name="security" requirePermission="false" type="Elmah.SecuritySectionHandler, Elmah" />
<section name="errorLog" requirePermission="false" type="Elmah.ErrorLogSectionHandler, Elmah" />
<section name="errorMail" requirePermission="false" type="Elmah.ErrorMailSectionHandler, Elmah" />
<section name="errorFilter" requirePermission="false" type="Elmah.ErrorFilterSectionHandler, Elmah" />
</sectionGroup>
</configSections>
<system.web>
<httpModules>
<add name="ErrorLog" type="Elmah.ErrorLogModule, Elmah" />
<add name="ErrorMail" type="Elmah.ErrorMailModule, Elmah" />
<add name="ErrorFilter" type="Elmah.ErrorFilterModule, Elmah" />
</httpModules>
</system.web>
<system.webServer>
<modules>
<add name="ErrorLog" type="Elmah.ErrorLogModule, Elmah" preCondition="managedHandler" />
<add name="ErrorMail" type="Elmah.ErrorMailModule, Elmah" preCondition="managedHandler" />
<add name="ErrorFilter" type="Elmah.ErrorFilterModule, Elmah" preCondition="managedHandler" />
</modules>
</system.webServer>
<elmah>
<security allowRemoteAccess="false" />
<errorLog type="Elmah.SqlErrorLog, Elmah" connectionStringName="YourConnectionStringName" />
</elmah>
<location path="elmah.axd" inheritInChildApplications="false">
<system.web>
<httpHandlers>
<add verb="POST,GET,HEAD" path="elmah.axd" type="Elmah.ErrorLogPageFactory, Elmah" />
</httpHandlers>
<!--
<authorization>
<allow roles="admin" />
<deny users="*" />
</authorization>
-->
</system.web>
<system.webServer>
<handlers>
<add name="ELMAH" verb="POST,GET,HEAD" path="elmah.axd" type="Elmah.ErrorLogPageFactory, Elmah" preCondition="integratedMode" />
</handlers>
</system.webServer>
</location>
</configuration>
Usage Now, It's time to use and test elmah for application. Generate exception in Home Controller
public ActionResult Index()
{
throw new Exception("This is test Exception");
return View();
}
after generating exception check your elmah like http://www.example.com/elmah.axd
Here is our output
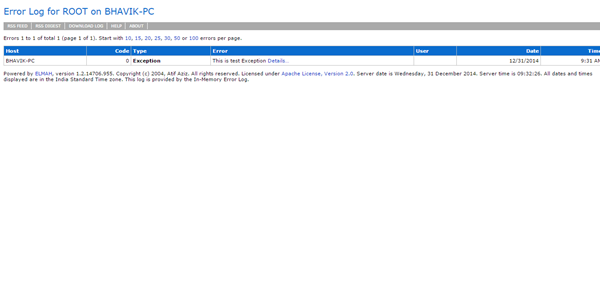
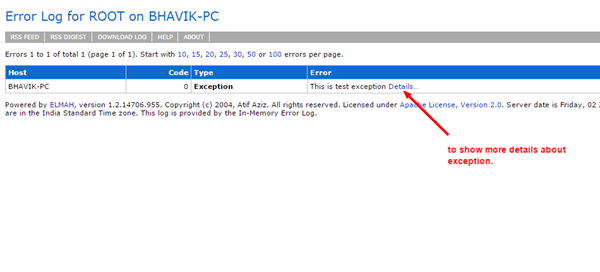
Security
In addition ELMAH provides seamless security feature to prevent unauthorized access. Please read our next article to make your elmah secure.
Filtering
ELMAH identify and store exceptions in different category, you can make or edit ELMAH error screen with different filters which we will discuss in our next ELMAH series.
Notification
You can setup ELMAH email notification when any exception occurs. To unable notification option you must include below code
Add ErrorMail module
<httpModules>
<add name="ErrorMail" type="Elmah.ErrorMailModule, Elmah"/>
</httpModules>
Add SMTP Setting
<system.net>
<mailSettings>
<smtp deliveryMethod="network">
<network host="..." port="25" userName="..." password="..." />
</smtp>
</mailSettings>
</system.net>
or
<elmah>
<errorMail from="..." to="..." async="true" smtpServer="..." smtpPort="25" userName="..." password="..." />
</elmah>
HostForLIFE.eu ASP.NET MVC Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
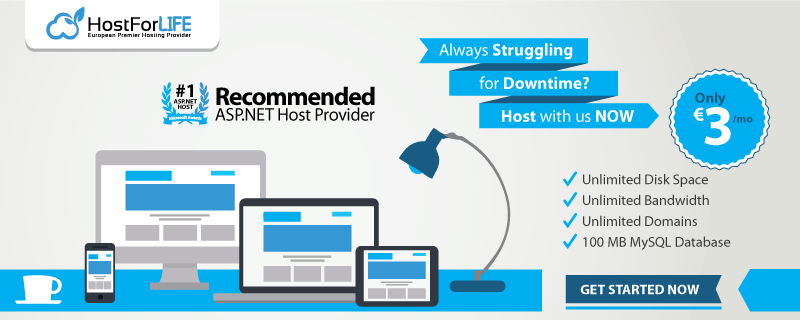