For small MVC sample application I’m fiddling with on my spare time, I wanted to be able to split my form up in smaller parts, much like the ASP.NET Wizard Control that is available to you when you are using Web Forms.
Basically I wanted a pretty simple Wizard, where I break up the input fields in a form in two or more steps, and display a summary at the end. I wanted the users to be able to step through the wizard without filling in required fields (just so they can get a grasp of the amount of info they would need to fill in), but of course they should be stopped when trying to submit it if anything is missing. I also wanted to avoid going to the server to retrieve a partial view for the summary.
The model I will use is pretty straight forward. It contains some fields for the user to fill inn, that I will split up in “Personal Details”, “Address” and “Contact Details”:
public class SimpleWizardFormModel : IValidatableObject
{
[Required]
[Display(Name = "First Name")]
public string FirstName { get; set; }
[Required]
[Display(Name = "Last Name")]
public string LastName { get; set; }
[Display(Name = "Street Address")]
public string Address { get; set; }
[Required]
[Display(Name = "Postal Code")]
public string PostalCode { get; set; }
[Required]
[Display(Name = "City")]
public string City { get; set; }
[Display(Name = "Home Phone")]
public string Phone { get; set; }
[Display(Name = "Mobile Phone")]
public string Mobile { get; set; }
[Display(Name = "I'm at least 18 years old?")]
public bool HasTurned18 { get; set; }
public IEnumerable<ValidationResult> Validate(ValidationContext validationContext)
{
if (!HasTurned18)
yield return new ValidationResult("You must be 18 or older.", new[] { "HasTurned18" });
}
}
The view isn’t very complicated either:
@model SimpleWizardFormModel
@section head
{
<style type="text/css">
.wizard-step { display: none; }
.wizard-confirmation { display: none; }
.wizard-nav { }
.wizard-nav input[type="button"] { width: 100px; }
</style>
}
@section script
{
<script type="text/javascript">
//*SNIP*
</script>
}
<h2>Simple Wizard Form</h2>
@using (Html.BeginForm())
{
<fieldset>
<legend></legend>
<div class="wizard-step">
<h4>Personal Details</h4>
<ol>
<li>
@Html.LabelFor(m => m.FirstName)
@Html.TextBoxFor(m => m.FirstName)
@Html.ValidationMessageFor(m => m.FirstName)
</li>
<li>
@Html.LabelFor(m => m.LastName)
@Html.TextBoxFor(m => m.LastName)
@Html.ValidationMessageFor(m => m.LastName)
</li>
<li>
@Html.LabelFor(m => m.HasTurned18)
@Html.CheckBoxFor(m => m.HasTurned18)
@Html.ValidationMessageFor(m => m.HasTurned18)
</li>
</ol>
</div>
<div class="wizard-step">
@**SNIP**@
</div>
<div class="wizard-step wizard-confirmation">
<h4>Confirm</h4>
<div id="field-summary"></div>
<div id="validation-summary">
<span class="message-error">Please correct the following errors;</span>
@Html.ValidationSummary(true)
</div>
</div>
<div class="wizard-nav">
<input type="button" id="wizard-prev" value="<< Previous" />
<input type="button" id="wizard-next" value="Next >>" />
<input type="button" id="wizard-submit" value="Submit" />
</div>
</fieldset>
}
I’ve cut out the javascript as I will get back to that later, as well as a couple of the wizard steps since they are look just like step 1 (just with other input fields). Inside my Layout.cshtml-file I’m importing jquery, jquery.validate, jquery.validate.unobtrusive, and rendering the “head”-section (in the head-tag) and “script”-section (just before body-close-tag) seen above.
The most important “feature” of the view are the divs which have been given the “wizard-step”-class. These contains the various input fields and will become the (as the class name suggests) steps in the wizard that is presented to the user. Initially all these divs are hidden from the user (note the display –> none in the css styles), and the javascript will take care of showing the div that represents the current wizard step to the user.
And now the stuff which actually performs some work, the javascript:
function DisplayStep() {
var selectedStep = null;
var firstInputError = $("input.input-validation-error:first"); // check for any invalid input fields
if (firstInputError.length) {
selectedStep = $(".wizard-confirmation");
if (selectedStep && selectedStep.length) { // the confirmation step should be initialized and selected if it exists present
UpdateConfirmation();
}
else {
selectedStep = firstInputError.closest(".wizard-step"); // the first step with invalid fields should be displayed
}
}
if (!selectedStep || !selectedStep.length) {
selectedStep = $(".wizard-step:first"); // display first step if no step has invalid fields
}
$(".wizard-step:visible").hide(); // hide the step that currently is visible
selectedStep.fadeIn(); // fade in the step that should become visible
// enable/disable the prev/next/submit buttons
if (selectedStep.prev().hasClass("wizard-step")) {
$("#wizard-prev").show();
}
else {
$("#wizard-prev").hide();
}
if (selectedStep.next().hasClass("wizard-step")) {
$("#wizard-submit").hide();
$("#wizard-next").show();
}
else {
$("#wizard-next").hide();
$("#wizard-submit").show();
}
}
The first method in my javascript, called “DisplayStep”, takes care of displaying the correct wizard step (typically this means the first step) when the view is loaded. if the view is loaded after submitting it to the server and server validation errors are found however, it will show the confirmation step if there is one, and if not it will show the first step which contains erroneous input. Once the correct step to show is found, it will decide where this step is located in relation to the other steps and show or hide the “previous”, “next” and “submit” buttons.
function PrevStep() {
var currentStep = $(".wizard-step:visible"); // get current step
if (currentStep.prev().hasClass("wizard-step")) { // is there a previous
step?
currentStep.hide().prev().fadeIn(); // hide current step and display previous step
$("#wizard-submit").hide(); // disable wizard-submit button
$("#wizard-next").show(); // enable wizard-next button
if (!currentStep.prev().prev().hasClass("wizard-step")) { // disable wizard-prev button?
$("#wizard-prev").hide();
}
}
}
The “PrevStep” method is pretty straight forward. It just finds the current step, hides it, shows the previous one, and shows/hides the buttons. No validation is performed before navigation to the previous step, but if desired, this could be done just like in the “NextStep” shown below.
function NextStep() {
var currentStep = $(".wizard-step:visible"); // get current step
var validator = $("form").validate(); // get validator
var valid = true;
currentStep.find("input:not(:blank)").each(function () { // ignore empty fields, i.e. allow the user to step through without filling in required fields
if (!validator.element(this)) { // validate every input element inside this step
valid = false;
}
});
if (!valid)
return; // exit if invalid
if (currentStep.next().hasClass("wizard-step")) { // is there a next step?
if (currentStep.next().hasClass("wizard-confirmation")) { // is the next step the confirmation?
UpdateConfirmation();
}
currentStep.hide().next().fadeIn(); // hide current step and display next step
$("#wizard-prev").show(); // enable wizard-prev button
if (!currentStep.next().next().hasClass("wizard-step")) { // disable wizard-next button and enable wizard-submit?
$("#wizard-next").hide();
$("#wizard-submit").show();
}
}
}
The “NextStep” is a little more complicated. In addition to performing pretty much the same tasks as the “PrevStep” (only the opposite), it validates all input fields in the current step, and if there are any errors, you won’t be allowed to go to the next step. It only validates none empty fields however, i.e. the required rule if applicable for a given field isn’t evaluated. This is done because I wanted the user to be able to step through the entire form to see how much needs to be filled in (you can easily change this by changing the part of the script where the input fields are found). If the next step has been given the “wizard-confirmation”-class a call is also made to setup/update the confirmation (the specifics of this function will be explained further down).
function Submit() {
if ($("form").valid()) { // validate all fields, including blank required
fields
$("form").submit();
}
else {
DisplayStep(); // validation failed, redisplay correct step
}
}
The last function related to navigation is “Submit”. This function validates the entire form (including required fields), and submits the form if all is good, or calls “DisplayStep” to show the confirmation step (if there is one), or the first step with errors on it (in cases where there are no confirmation step).
function UpdateConfirmation() {
UpdateValidationSummary();
var fieldList = $("<ol/>");
$(".wizard-step:not(.wizard-confirmation)").find("input").each(function () {
var input = this;
var value;
switch (input.type) {
case "hidden":
return;
case "checkbox":
value = input.checked;
break;
default:
value = input.value;
}
var name = $('label[for="' + input.name + '"]').text();
fieldList.append("<li><label>" + name + "</label><span>" + value + " </span></li>");
});
$("#field-summary").children().remove();
$("#field-summary").append(fieldList);
}
function UpdateValidationSummary() {
var validationSummary = $("#validation-summary");
if (!validationSummary.find(".validation-summary-errors").length) { // check if validation errors container already exists, and if not create it
$('<div class="validation-summary-errors"><ul></ul></div>').appendTo(validationSummary);
}
var errorList = $(".validation-summary-errors ul");
errorList.find("li.field-error").remove(); // remove any field errors that might have been added earlier, leaving any server errors intact
$('.field-validation-error').each(function () {
var element = this;
$('<li class="field-error">' + element.innerText + '</li>').appendTo(errorList); // add the current field errors
});
if (errorList.find("li").length) {
$("#validation-summary span").show();
}
else {
$("#validation-summary span").hide();
}
}
The “UpdateConfirmation” function (and the “UpdateValidationSummary”-function called by this function) takes care of setting up / displaying the confirmation step. The “UpdateValidationSummary” function finds all input errors (if any) and adds them to the server validation error list (creating this list if it doesn’t already exist). The “UpdateConfirmation” function, in addition to calling “UpdateValidationSummary”, finds all input fields and associated labels and created a list with them that is displayed to the user.
$(function () {
// attach click handlers to the nav buttons
$("#wizard-prev").click(function () { PrevStep(); });
$("#wizard-next").click(function () { NextStep(); });
$("#wizard-submit").click(function () { Submit(); });
// display the first step (or the confirmation if returned from server with errors)
DisplayStep();
});
Last part of the javascript is where we hook up handlers for the navigation buttons and calls the function to display the first (or correct) step when the view is first loaded.
That was all the code needed, not to bad if I say so myself.
A couple of screens to show how it looks in action (first picture shows one of the steps, while the second picture shows the confirmation step):
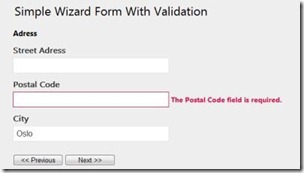
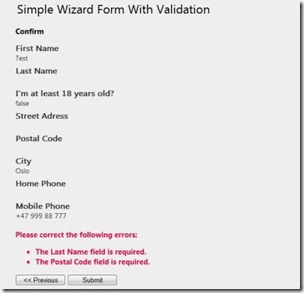
As I said in the beginning, this wizard is pretty basic, but it works pretty good.