
November 9, 2018 10:58 by
Peter
Here are the steps:
Step 1: Create the basic structure of your project, View, multiple partial views View Model,
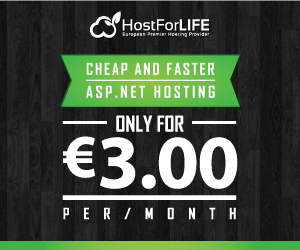
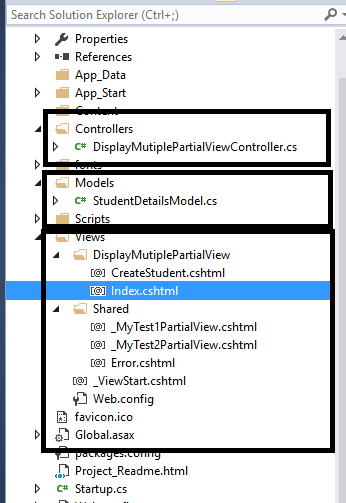
Step 2: Create your base Controller as in the following,
public class BaseController: Controller
{
protected internal virtual CustomJsonResult CustomJson(object json = null, bool allowGet = true)
{
return new CustomJsonResult(json)
{
JsonRequestBehavior = allowGet ? JsonRequestBehavior.AllowGet : JsonRequestBehavior.DenyGet
};
}
}
It is just small modifications if JSON is not provided handle it. And use this Controller as base controller.
Step 3: Add Class Controller helper which helps you to convert partial view into the string format as in the following,
public static class ControllerHelper
{
public static string RenderPartialViewToString(ControllerContext context, string viewName, object model)
{
var controller = context.Controller;
var partialView = ViewEngines.Engines.FindPartialView(controller.ControllerContext, viewName);
var stringBuilder = new StringBuilder();
using(var stringWriter = new StringWriter(stringBuilder))
{
using(var htmlWriter = new HtmlTextWriter(stringWriter))
{
controller.ViewData.Model = model;
partialView.View.Render(new ViewContext(controller.ControllerContext, partialView.View, controller.ViewData, new TempDataDictionary(), htmlWriter), htmlWriter);
}
}
return stringBuilder.ToString();
}
}
Step 4: Your action code is below. I have added the 2 partial views. Basically we are converting the partial view with objects into the string format.
public JsonResult CreatePartialView()
{
MyMultipleUpdateViewModel obj = new MyMultipleUpdateViewModel();
obj.myTest1ViewModel = new MyTest1ViewModel();
obj.myTest1ViewModel.MyTestUpdate = "Test1" + DateTime.Now.ToString();
obj.myTest2ViewModel = new MyTest2ViewModel();
obj.myTest2ViewModel.MyTestUpdate = "Test2" + DateTime.Now.ToString();
var json = new
{
Header = "Header", Footer = "Footer"
};
return CustomJson(json).AddPartialView("_MyTest1PartialView", obj).AddPartialView("_MyTest2PartialView", obj);
}
Step 5: In View display this JSON data ajax in below way . In below code PartialViewDiv1 and PartialViewDiv2 are two divs in which two partial views will be displayed. I have two partial views you may load more partial views.
<h2>Index</h2>
<script type="text/javascript" src="@Url.Content(" ~/Scripts/jquery-1.10.2.js ")"></script>
<script type="text/javascript" src="@Url.Content(" ~/Scripts/jquery.unobtrusive-ajax.min.js ")"></script>
<script>
function GetData(url, onSuccess)
{
$.ajax(
{
type: "GET",
cache: false,
url: url,
dataType: "json",
success: function(data, textStatus, jqxhr)
{
onSuccess(data.Json, data.Html);
},
error: function(data, text, error)
{
alert("Error: " + error);
}
});
return false;
}
function UpdateDiv(json, html)
{
$("#PartialViewDiv1").html(html[0]);
$("#PartialViewDiv2").html(html[1]);
}
</script>
<input type="button" onclick="GetData('/DisplayMutiplePartialView/CreatePartialView', UpdateDiv);" value="Display Multiple Partial View" />
<br>
<br>
<div id="PartialViewDiv1"></div>
<br>
<br>
<div id="PartialViewDiv2"></div>
<br>
<br>
Step 6: Run application and use URL,
It will appear as below
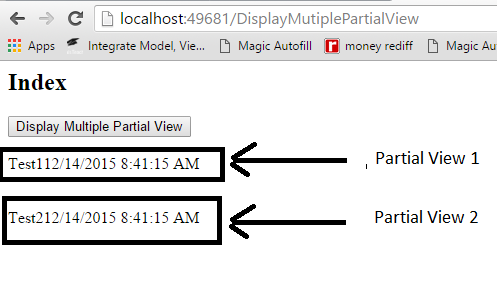
HostForLIFE.eu ASP.NET MVC 6 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
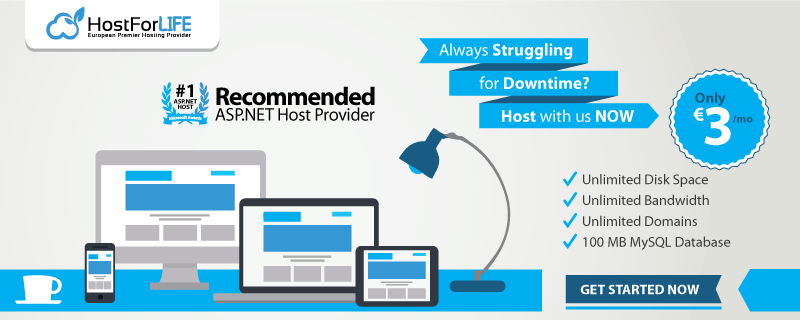