Sometimes, the controller actions can trigger a long running background process. For example, when the user clicks a link in the page, a word document is generated in the background, and the properties of the word document is shown in the subsequent page. Generation of word documents can take anywhere between 3 seconds to 30 seconds. During this time, the user needs some feedback about the progress of the operation. This post shows how you can provide progress information to the page which triggered the long running background process.
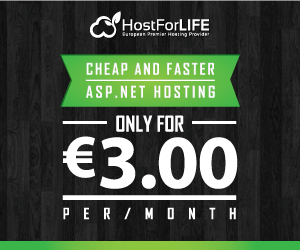
Step 1
Consider a MVC application with two pages: Index.cshtml and Generate.cshtml. The Index.cshtml has a link - Generate. When the user clicks the link, the Generate page is shown. The Generate action is a long running operation that happens in the background. To execute long running operations from a MVC controller, we derive the controller from AsyncController. The following code snippet shows the HomeController with the background operations:
public class HomeController : AsyncController
{
//
// GET: /Home/
private BackgroundWorker worker = default(BackgroundWorker);
private static int progress = default(int);
public ActionResult Index()
{
return View();
}
public void GenerateAsync()
{
worker = new BackgroundWorker();
worker.WorkerReportsProgress = true;
worker.DoWork += worker_DoWork;
worker.ProgressChanged += worker_ProgressChanged;
AsyncManager.OutstandingOperations.Increment();
worker.RunWorkerAsync();
}
void worker_ProgressChanged(object sender, ProgressChangedEventArgs e)
{
progress = e.ProgressPercentage;
}
void worker_DoWork(object sender, DoWorkEventArgs e)
{
for (int i = 0; i < 100; i++)
{
Thread.Sleep(300);
worker.ReportProgress(i+1);
}
AsyncManager.OutstandingOperations.Decrement();
}
public ActionResult GenerateCompleted()
{
return View();
}
public ActionResult GetProgress()
{
return this.Json(progress, JsonRequestBehavior.AllowGet);
}
}
The three main action methods in the above controller are - Index(), GenerateAsync(), GetProgress(). Index() displays the Index page. GenerateAsync() triggers the background operation. The background operation loops 100 times and sleeps for 300 ms in each iteration. At the end of each iteration, it reports progress as a percentage.
Step 2
The GetProgress() action gets the reported progress which is stored as a static variable. The GetProgress() is triggered when the user clicks the link. The javascript in the Index page shows how the GetProgress() action method is called:
$(document).ready(
function () {
$("#genLink").click(
function (e) {
setInterval(
function () {
$.get("/Home/GetProgress",
function (data) {
$("#progress").text(data);
});
}, 1000);
});
});
Here's the HTML
<body>
<h1>Progress</h1>
<h2 id="progress"></h2>
<div>
@Html.ActionLink("Generate", "Generate", null,
new { id = "genLink" })
</div>
</body>
On clicking the link, you have caledl the setInterval() function that is triggered every second. In the recurring function, we call the action method - GetProgress(). You have displayed the progress data in the page in the progress tag.
HostForLIFE.eu ASP.NET MVC 6 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
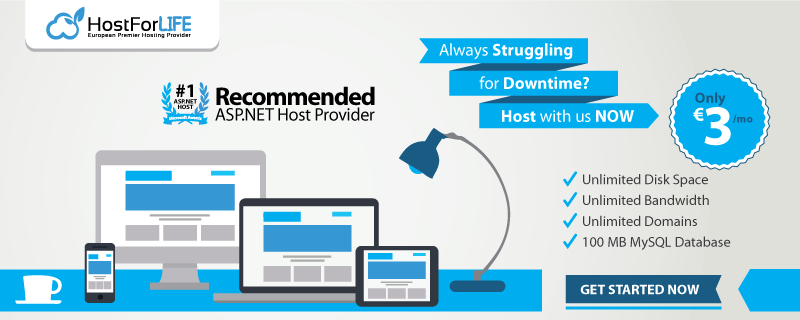