Sometimes, we need to create a PDF file that opens only when the users put in a password when prompted. Let us see how to create a password-protected PDF file in MVC.
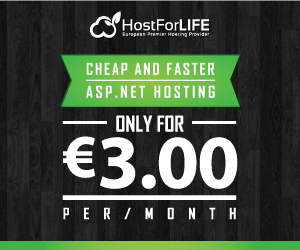
First, let's open Visual Studio and create a new project. We need to select the ASP.NET Web application type.
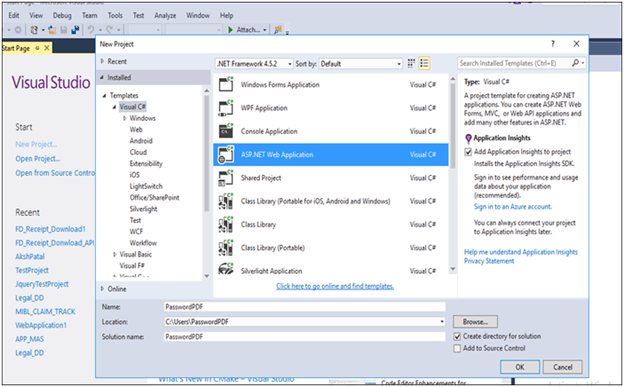
Select Web API as the template and in the "Add folders and core references" section, we need to select MVC and Web API. Click on "Change Authentication" on the right side pane and select "No Authentication".
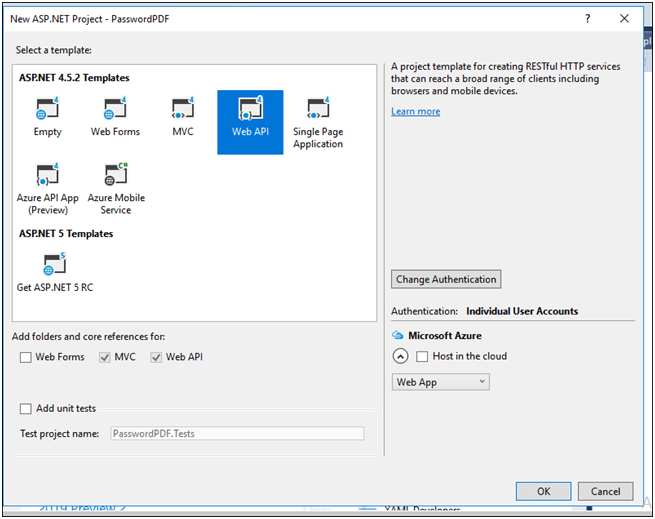
In the web.config file, let us define one key named Filepath and use it in our code. The PDF file must be present there.
It is good to change the key's value when it's placed in web.config.
<appSettings>
<add key="FilePath" value="Anil\PDF\LDEPRD9.pdf"/>
</appSettings>
Add the below code to the Home Controller.
string FilePath = ConfigurationManager.AppSettings["FilePath"].ToString();
public ActionResult DownloadFile()
{
try
{
byte[] bytes = System.IO.File.ReadAllBytes(FilePath);
using (MemoryStream inputData = new MemoryStream(bytes))
{
using (MemoryStream outputData = new MemoryStream())
{
string PDFFilepassword = "123456";
PdfReader reader = new PdfReader(inputData);
PdfReader.unethicalreading = true;
PdfEncryptor.Encrypt(reader, outputData, true, PDFFilepassword, PDFFilepassword, PdfWriter.ALLOW_SCREENREADERS);
bytes = outputData.ToArray();
Response.AddHeader("content-length", bytes.Length.ToString());
Response.BinaryWrite(bytes);
return File(bytes, "application/pdf");
}
}
}
catch (Exception ex)
{
throw ex;
}
}
string PDFFilepassword = "123456";
PdfReader reader = new PdfReader(inputData);
PdfReader.unethicalreading = true;
PdfEncryptor.Encrypt(reader, outputData, true, PDFFilepassword, PDFFilepassword, PdfWriter.ALLOW_SCREENREADERS);
In the PDFFilepassword variable, you can set anything as password - the file name, PAN card number, or you can validate the entered value against the values stored in the database.
In Route.config, we can define the default route with the Controller And ActionName.
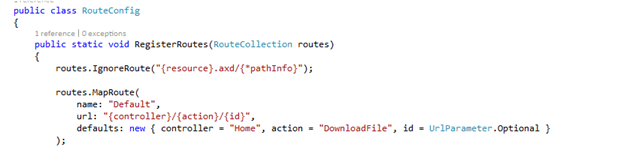
Run the website and enter http://localhost:49744/Home/DownloadFile.
Here, Home is the controller name and DownloadFile is the action name.
It shows the following Password prompt.
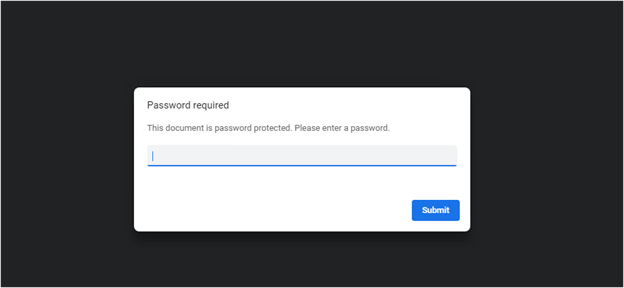
After entering the right password and successful authentication, the PDF file will get opened.
HostForLIFE.eu ASP.NET MVC 6 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
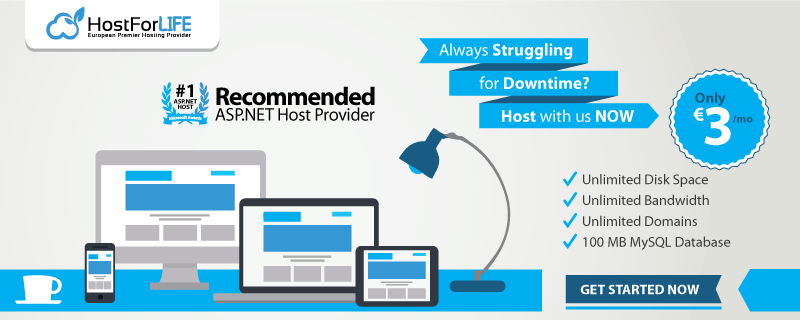