In this tutorial, I will show you how to make simple application in ASP.NET MVC using Select2. The infinite scrolling feature has also been implemented with server side options population. Select2 is very useful for dropdown lists with large datasets.
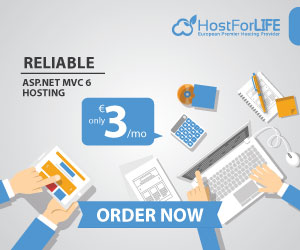
Why Select2 :
- Using this jQuery plugin for dropdown lists, you can implement features such as option grouping, searching, infinite scrolling, tagging, remote data sets and other highly used features.
- To use select2 in web projects, you just have to include JavaScript and CSS files of Select2 in your website.
- Current version of select2 is 4.0.0. You can easily include these files by installation of NuGet package ‘Select2.js’ from NuGet package manager.
Steps of Implementation:
1. Create a blank ASP.NET MVC project, and install NuGet packages Select2.js, jQuery and jQuery Unobtrusive.
2. Add one controller with the name ‘HomeController’ and add view for default method ‘Index’.
3. Create new class in Models folder ‘IndexViewModel.cs as shown below:
public class IndexViewModel
{
[Required(ErrorMessage="Please select any option")]
public string OptionId { get; set; }
}
4. Bind ‘Index.cshtml’ view with IndexViewModelClass as model, by adding the following line in Index view:
@model Select2InMvcProject.Models.IndexViewModel
5. In ‘Index.cshtml’, include the css and js files below:
<link href="~/Content/css/select2.css" rel="stylesheet" />
<script src="~/Scripts/jquery-2.1.4.js"></script>
<script src="~/Scripts/select2.js"></script>
<script src="~/Scripts/jquery.validate.js"></script>
<script src="~/Scripts/jquery.validate.unobtrusive.js"></script>
6. Write the following code in the Index view for creation of select list:
@using (Html.BeginForm())
{
<br /><br />
@Html.DropDownListFor(n => n.OptionId, Enumerable.Empty<SelectListItem>(), new { @id = "txtOptionId", @style = "width:300px;" })
//Created selectlist with empty enumerable of SelectListItem and given //id as “txtOptionId”
@Html.ValidationMessageFor(n => n.OptionId)
//Adds span of validation error message
<br /><br />
<button type="submit">Submit</button>
<br /><br />
}
7. For applying Select2 to the dropdown list created above, fetching data from server side and for infinite scroll, use the jQuery code below in Index view:
<script type="text/javascript">
$(document).ready(function () {
var pageSize = 20;
var optionListUrl = '@Url.Action("GetOptionList", "Home")';
//Method which is to be called for populating options in dropdown //dynamically
$('#txtOptionId').select2(
{
ajax: {
delay: 150,
url: optionListUrl,
dataType: 'json',
data: function (params) {
params.page = params.page || 1;
return {
searchTerm: params.term,
pageSize: pageSize,
pageNumber: params.page
};
},
processResults: function (data, params) {
params.page = params.page || 1;
return {
results: data.Results,
pagination: {
more: (params.page * pageSize) < data.Total
}
};
}
},
placeholder: "-- Select --",
minimumInputLength: 0,
allowClear: true,
});
});
</script>
8. Create new class in Models folder with name ‘Select2OptionModel’ and add the two classes below:
public class Select2OptionModel
{
public string id { get; set; }
public string text { get; set; }
}
public class Select2PagedResult
{
public int Total { get; set; }
public List<Select2OptionModel> Results { get; set; }
}
9. Create one new folder with name ‘Repository’ in the solution, and add new class in that folder with name ‘Select2Repository. The functions in this class are mentioned below:
public class Select2Repository
{
IQueryable<Select2OptionModel> AllOptionsList;
public Select2Repository()
{
AllOptionsList = GetSelect2Options();
}
IQueryable<Select2OptionModel> GetSelect2Options()
{
string cacheKey = "Select2Options";
//check cache
if (HttpContext.Current.Cache[cacheKey] != null)
{
return (IQueryable<Select2OptionModel>)HttpContext.Current.Cache[cacheKey];
}
var optionList = new List<Select2OptionModel>();
var optionText = "Option Number ";
for (int i = 1; i < 1000; i++)
{
optionList.Add(new Select2OptionModel
{
id = i.ToString(),
text = optionText + i
});
}
var result = optionList.AsQueryable();
//cache results
HttpContext.Current.Cache[cacheKey] = result;
return result;}
List<Select2OptionModel> GetPagedListOptions(string searchTerm, int pageSize, int pageNumber, out int totalSearchRecords)
{
var allSearchedResults = GetAllSearchResults(searchTerm);
totalSearchRecords = allSearchedResults.Count;
return allSearchedResults.Skip((pageNumber - 1) * pageSize).Take(pageSize).ToList();
}
List<Select2OptionModel> GetAllSearchResults(string searchTerm)
{
var resultList = new List<Select2OptionModel>();
if (!string.IsNullOrEmpty(searchTerm))
resultList = AllOptionsList.Where(n => n.text.ToLower().Contains(searchTerm.ToLower())).ToList();
else
resultList = AllOptionsList.ToList();
return resultList;
}
public Select2PagedResult GetSelect2PagedResult(string searchTerm, int pageSize, int pageNumber)
{
var select2pagedResult = new Select2PagedResult();
var totalResults = 0;
select2pagedResult.Results = GetPagedListOptions(searchTerm,
pageSize, pageNumber, out totalResults);
select2pagedResult.Total = totalResults;
return select2pagedResult;
}
}
10. In HomeController class, create new method as shown below:
public JsonResult GetOptionList(string searchTerm, int pageSize, int pageNumber)
{
var select2Repository = new Select2Repository();
var result = select2Repository.GetSelect2PagedResult(searchTerm, pageSize, pageNumber);
return Json(result, JsonRequestBehavior.AllowGet);
}
11. Once you are done with coding, you can build and run the project. The output will be shown as below:
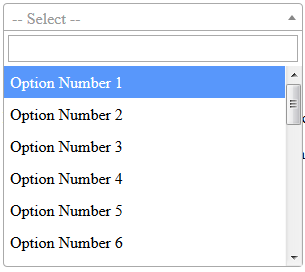
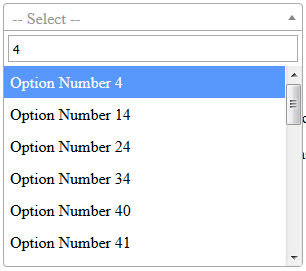
HostForLIFE.eu ASP.NET MVC Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
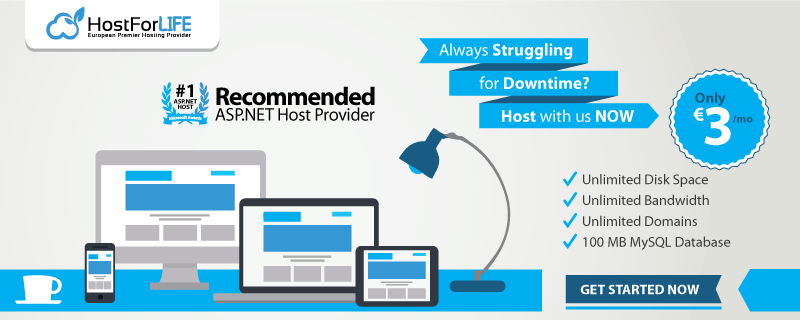