If you have worked on ASP.NET MVC, you must be familiar with how when you type in an URL, the appropriate controller is chosen, and the action fired. Today we will dig a little deeper within the MVC request life cycle. Before we start discussing its life cycle, let's briefly understand the concept of HttpHandlers and HttpModules.
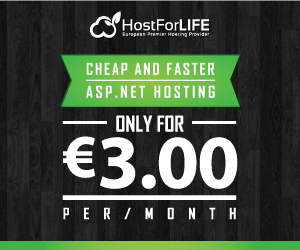
Handlers are responsible for generating the actual response in MVC. They implement the IHttpHandler class and only one handler will execute per request. On the other hand, HttpModules are created in response to life cycle events. Modules can, for example, be used for populating HttpContext objects. A request can use many modules. These classes derive from IHttpModule. We are now ready to learn about the MVC Request Life Cycle. The MVC life cycle can be briefly demonstrated as below,
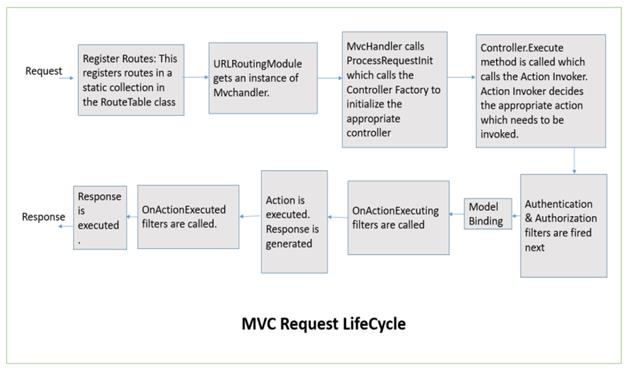
When a request is fired for the first time, the Application_Start method in the Global.asax file is called. This method calls the RegisterRoutes method as below,
public class MvcApplication : System.Web.HttpApplication
{
protected void Application_Start()
{
AreaRegistration.RegisterAllAreas();
FilterConfig.RegisterGlobalFilters(GlobalFilters.Filters);
RouteConfig.RegisterRoutes(RouteTable.Routes);
BundleConfig.RegisterBundles(BundleTable.Bundles);
}
}
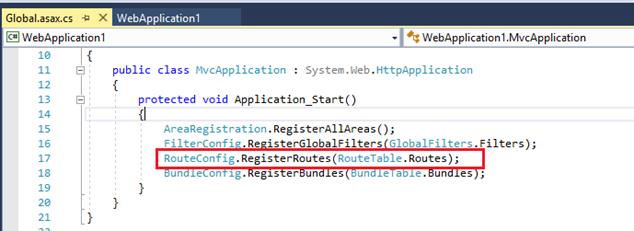
RegisterRoutes method stores the routes defined in that method, in a static collection in the Routetable class.
Each route has an HttpHandler defined for it. In our case above, the MapRoute method defines the HttpHandler.
Next, the URLRoutingModule is called. It matches the request route with the routes defined in the route table. It calls the GetHttpHandler method which returns an instance of an MVCHandler.
The MVCHandler calls the ProcessRequest method. The controller execution and initialization happens inside this method. ProcessRequest calls ProcessRequestInit, which uses ControllerFactory to select an appropriate controller based on the supplied route. The ControllerFactory calls the Controller Activator which uses the dependency resolver to create an instance of the controller class.
Once the controller is created its Execute method is called.
Now comes the point where the action must be executed. The execute method in the controller calls the ExecuteCore method which calls the InvokeAction method of ActionInvoker. Action Invoker determines which action must be selected based on certain conditions, depending upon the methods available, their names and the action selectors used for them.
Once the action is selected, Authentication & Authorization filters are fired next.
Once the action passes through the authentication and authorization filter checks, the model binding takes place. The information needed for the action to execute is gathered in this step.
OnActionExecuting action filters are fired next. Once the OnActionExecuting filters are executed a response for the action is generated. The thing to note here is that the response is generated at this stage, but not executed.
Next, the OnActionExecuted filters are executed. Once all the filters have finished executing, the response is finally executed in the ExecuteResult method which is called from the InvokeActionResult by the ActionInvoker. If the response is a view or a partial view, the ViewEngine will render it, else it will be handled appropriately. The ExecuteResult will find the appropriate view using FindView or FindPartialView method. This method will search for the view in specific locations and then render it. This is the final step in generating the response.
If you would like to further dig into the MVC request life cycle, I would highly recommend Alex Wolf’s pluralsight course by the same name.
HostForLIFE.eu ASP.NET MVC 6 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
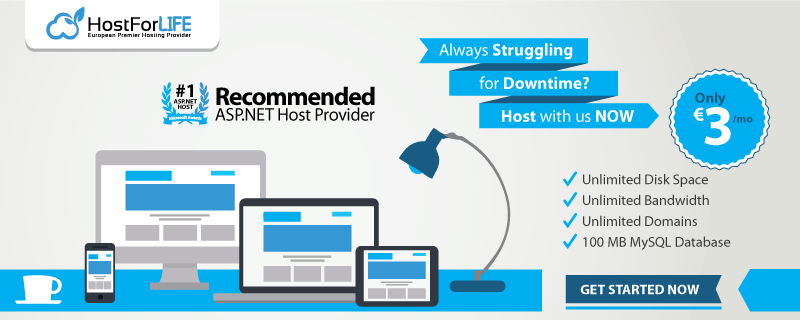