Single Page Applications (SPA)?
Normally a web application is a collection of web pages, each doing a specific task. For example, consider a web application that does CRUD operations (Create, Read, Update and Delete) on data. A common practice is to create different web pages for operations such as showing a list of existing records, adding a new record, updating an existing record and deleting a record. A trend becoming increasingly popular is to have a single web page perform all of these operations. Such an application is called Single Page Application or SPA. So, in this example instead of developing four separate web pages you develop just one web page. At runtime, depending on the operation selected by a user, the web page renders an appropriate user interface. Such an application heavily relies on client side JavaScript libraries.
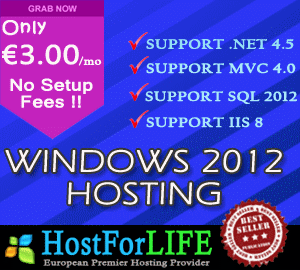
It should be noted that SPA is a general concept and ASP.NET MVC 4 has decided to offer some basic infrastructure to the developers to put this concept into practice. ASP.NET MVC 4 provides a project template that creates a basic yet functional SPA application. You can then customize the application to add more functionality. In the discussion that follows you will learn SPA with respect to ASP.NET MVC 4.
Parts of SPA
A Single Page Application consists of several pieces that fit together to provide the overall functionality of the application. A typical SPA consists of the following pieces:
- Data Model : This is a server side piece that represents your data (often mapping database tables as .NET objects).
- Data Service : Data service provides operations for database access (typically CRUD operations). This is also a service side piece and uses Entity Framework Code First approach.
- ViewModel : View Model refers to your data and UI level operations that you wish to perform on the data. You can think of View Model as a wrapper over your model data that adds UI level operations to it.
- Views : Views display your data to the user and also contain associated JavaScript. The default SPA project template uses Razor views.
- Database: SPA uses Entity Framework Code First approach for database operations. The default project template creates a database in the local installation of SQL Server Express.
The following sections discuss all these parts from the default SPA project template in detail.
Creating a New Project Based on SPA Template
Installing ASP.NET MVC 4 adds a new project template in Visual Studio 2010. To create a new SPA you should create an ASP.NET MVC4 Web Application project based on this template.
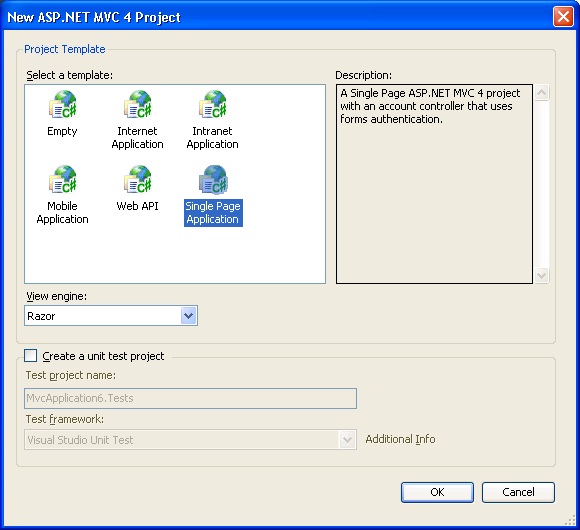
The default project created using the SPA project template contains data models, views and client script files for performing CRUD operations of a sample "To Do" application. SPA extensively uses two JavaScript libraries, namely Knockout and Upshot. The following figure shows these libraries added in the Solution Explorer.
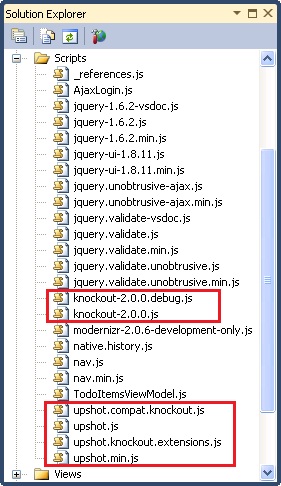
Data Model
The sample application created by the default SPA project template deals with "To Do" items, i.e. tasks. A task is represented by a data model class - TodoItem. The TodoItem class resides in the Models folder and looks like this:
public class TodoItem
{
public int TodoItemId { get; set; }
[Required]
public string Title { get; set; }
public bool IsDone { get; set; }
}
As you can see the TodoItem is a simple class with three properties, viz. TodoItemId, Title and IsDone. The Title property is a required property as indicated by Data Annotation Attribute [Required].
To deal with the application data you need to create a DbContext and a DbDataController. This is done for you when you add a new controller to the project specifying the SPA controller template. Right click on the Controllers folder and select Add > Controller. In the Add Controller dialog specify details as shown below:
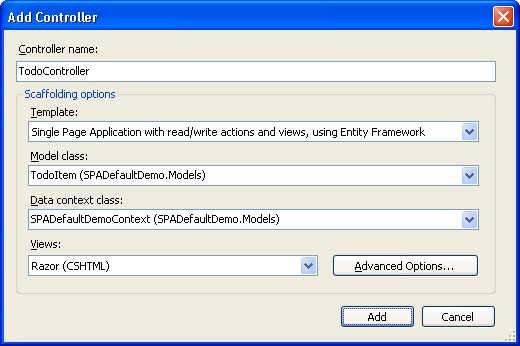
Specify the controller name as TodoController. Select scaffolding template of "Single Page Application with read/write actions and views, using Entity Framework". In the Model class drop-down select TodoItem class. In the Data context class drop-down click "New data context" and specify a name for the DbContext class. Once you click on the Add button the following files will be created for you:
- TodoController.cs (Controllers folder)
- SPADefaultDemoController.cs (Controllers folder)
- SPADefaultDemoController.TodoItem.cs (Controllers folder)
- SPADefaultDemoContext.cs (Models folder)
- Index.cshtml and associated partial views (Views folder)
Out of these classes the DbContext class (SPADefaultDemoContext) looks like this:
public class SPADefaultDemoContext : DbContext
{
public DbSet<TodoItem> TodoItems { get; set; }
}
As you can see the SPADefaultDemoContext class inherits from DbContext base class and contains a DbSet of TodoItem.
Data Service
The job of performing CRUD operations is handled by the SPADefaultDemoController (the data service) class. This class is shown below:
public partial class SPADefaultDemoController :
DbDataController<SPADefaultDemo.Models.SPADefaultDemoContext>
{
public IQueryable<SPADefaultDemo.Models.TodoItem> GetTodoItems() {
return DbContext.TodoItems.OrderBy(t => t.TodoItemId);
}
public void InsertTodoItem(SPADefaultDemo.Models.TodoItem entity) {
InsertEntity(entity);
}
public void UpdateTodoItem(SPADefaultDemo.Models.TodoItem entity) {
UpdateEntity(entity);
}
public void DeleteTodoItem(SPADefaultDemo.Models.TodoItem entity) {
DeleteEntity(entity);
}
}
As you can see the SPADefaultDemoController class inherits from DbDataController base class and includes methods for selecting, inserting, updating and deleting TodoItem records to the database. The data service is called from the client side JavaScript code as you will see later.
ViewModel
The ViewModel class for the TodoItem data model is created automatically for you and is placed in the Scripts folder.
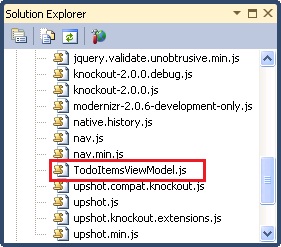
As you can see TodoItemsViewModel.js file is placed in the Scripts folder. This ViewModel is developed using Knockout and a part of it is shown below:
// TodoItem class
var entityType = "TodoItem:#SPADefaultDemo.Models";
MyApp.TodoItem = function (data) {
var self = this;
// Underlying data
self.TodoItemId = ko.observable(data.TodoItemId);
self.Title = ko.observable(data.Title);
self.IsDone = ko.observable(data.IsDone);
upshot.addEntityProperties(self, entityType);
}
...
As you can see the TodoItem ViewModel class contains the same properties as the server side data model. These properties are observable properties as indicated by ko.observable() syntax. Knockout synchronizes the data between views and ViewModel. The communication between the ViewModel and the server side data happens through Upshot.js.
View
The Index.cshtml file represents the main view of the application. Three partial views are also created viz. _Grid, _Editor and _Paging that provide the user interface for list, insert/update and paging respectively. Depending on the operation selected by the user the appropriate partial view is rendered. The following markup shows a fragment from the Index.cshtml.
@{
ViewBag.Title = "TodoItems";
Layout = "~/Views/Shared/_SpaLayout.cshtml";
}
<div data-bind="visible: editingTodoItem">
@Html.Partial("_Editor")
</div>
<div data-bind="visible: !editingTodoItem()">
@Html.Partial("_Grid")
</div>
<div class="message-info message-success" data-bind="flash: { text: successMessage, duration: 5000
}"></div>
<div class="message-info message-error" data-bind="flash: { text: errorMessage, duration: 20000 }"></div>
<script type="text/javascript" src="@Url.Content("~/Scripts/TodoItemsViewModel.js")"></script>
<script type="text/javascript">
$(function () {
upshot.metadata(@(Html.Metadata<SPADefaultDemo.Controllers.SPADefaultDemoController>()));
var viewModel = new MyApp.TodoItemsViewModel({
serviceUrl: "@Url.Content("~/api/SPADefaultDemo")"
});
ko.applyBindings(viewModel);
});
</script>
Notice that data service URL is specified as ~/api/SPADefaultDemo. The Html.Metadata() method provides the metadata of the types to the Upshot. The binding between View and ViewModel is provided by the applyBindings() method of Knockout.
If you run the application and navigate to http://localhost:1275/todo (change the port no. as per your setup) you will see something similar to the following figure.
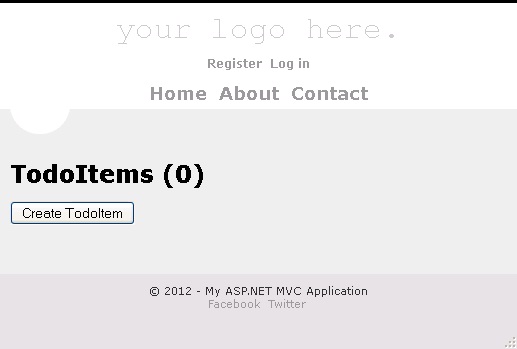
You can click on the "Create TodoItem" button to add a few records. You can then modify or delete them. The following figure shows the view in edit mode.
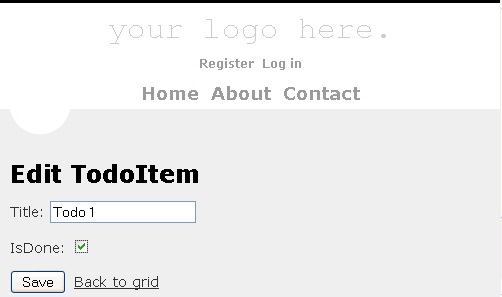
Database
At this stage the sample "To do" application is able to store and retrieve the data but you might be wondering where the actual data is. Since SPA uses Code First approach to database operations, the database is automatically created for you when you run the application for the first time. The subsequent runs use the previously created database. Have a look at the following figure that shows a sample database generated under local installation of SQL Express.
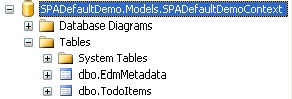
As you can see, by default the database name is the same as the fully qualified name of the DbContext class. Inside there is a TodoItems table that stores the application data.