
February 11, 2016 20:21 by
Peter
HTTP methods are not often thought about once writing ASP.NET webforms applications. Links are GETs, buttons are POSTs and it all happens automatically. With Asp.NET MVC, and other MVC frameworks like Rails, the http method used is more obvious and developers are begining to care about which they use.
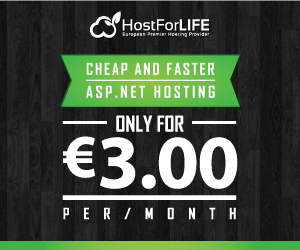
The problem is that GET requests tell visitors to your site, together with search engines, client-side web optimizers and other automatic tools, that it's safe to make the request. Which is a problem if your checkout button causes a GET. To quote Dave Thomas, paraphrasing Tim Berners-Lee, "Use GET requests to retrieve info from the server, and use POST requests to request a change of state on the server".
To help me correctly control which HTTP methods are used to access my controller actions I created an ActionFilterAttribute. ActionFilters provide a declarative way to access the executing context immediately prior to, and immediately following, the execution of an action. they're an excellent way to introduce aspect oriented programming to an asp.net mvc application. To use my action filter you attribute a controller action like this:
AllowedHttpMethods(AllowedMethods= new HttpMethods[] {HttpMethods.POST})]
public void Save()
{ ... }
The code for the Action Filter inherits from ActionFilterAttribute and overrides the OnActionExecuting event.
public class AllowedHttpMethodsAttribute : ActionFilterAttribute
{
public HttpMethods[] AllowedMethods { get; set; }
public override void OnActionExecuting(FilterExecutingContext filterContext)
{
int count = AllowedMethods.Count(m => m.ToString().Equals(filterContext.HttpContext.Request.HttpMethod));
if (count == 0) throw new Exception("Invalid http method: " + filterContext.HttpContext.Request.HttpMethod);
}
}
public enum HttpMethods
{
GET,POST
}
By adding the AllowedHttpMethods attribute to all of my controller actions I can assure that http methods are used correctly.
HostForLIFE.eu ASP.NET MVC 6 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
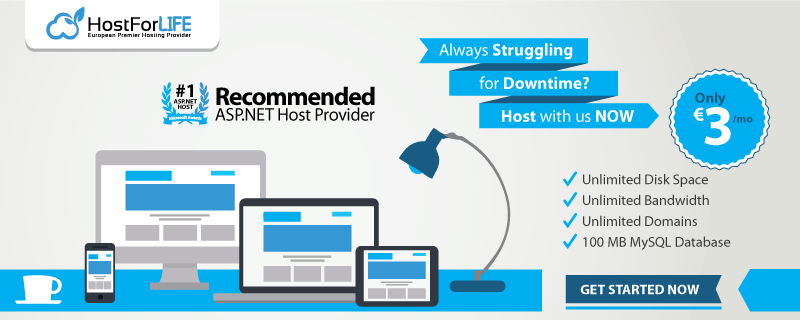