ASP.NET MVC 6 Hosting is the technology that brought me to Microsoft and the west-coast and it’s been fun getting to grips with it these last few weeks. Last week I needed to expose RSS feeds and checked out some examples online but was very disappointed. If you find yourself contemplating writing code to solve technical problems rather than the specific business domain you work in you owe it to your employer and fellow developers to see what exists before churning out code to solve it.
The primary excuse (and I admit to using it myself) is “X is too bloated, I only need a subset. I can write that quicker than learn their solution.” but a quick reality check:
1. Time – code always takes longer than you think
2. Bloat – indicates the problem is more complex than you realize
3. Growth – todays requirements will grow tomorrow
4. Maintenance – fixing code outside your business domain
5. Isolation – nobody coming in will know your home-grown solution
The RSS examples I found had their own ‘feed’ and ‘items’ classes and implemented flaky XML rendering by themselves or as MVC view pages.
If these people had spent a little time doing some research they would have discovered .NET’s built in SyndicatedFeed and SyndicatedItem class for content and two classes (Rss20FeedFormatter and Atom10FeedFormatter ) to handle XML generation with correct encoding, formatting and optional fields. All that is actually required is a small class to wire up these built-in classes to MVC.
using System;
using System.ServiceModel.Syndication;
using System.Text;
using System.Web;
using System.Web.Mvc;
using System.Xml;
namespace MyApplication.Something
{
public class FeedResult : ActionResult
{
public Encoding ContentEncoding { get; set; }
public string ContentType { get; set; }
private readonly SyndicationFeedFormatter feed;
public SyndicationFeedFormatter Feed{
get { return feed; }
}
public FeedResult(SyndicationFeedFormatter feed) {
this.feed = feed;
}
public override void ExecuteResult(ControllerContext context) {
if (context == null)
throw new ArgumentNullException("context");
HttpResponseBase response = context.HttpContext.Response;
response.ContentType = !string.IsNullOrEmpty(ContentType) ? ContentType : "application/rss+xml";
if (ContentEncoding != null)
response.ContentEncoding = ContentEncoding;
if (feed != null)
using (var xmlWriter = new XmlTextWriter(response.Output)) {
xmlWriter.Formatting = Formatting.Indented;
feed.WriteTo(xmlWriter);
}
}
}
}
In a controller that supplies RSS feed simply project your data onto SyndicationItems and create a SyndicationFeed then return a FeedResult with the FeedFormatter of your choice.
public ActionResult NewPosts() {
var blog = data.Blogs.SingleOrDefault();
var postItems = data.Posts.Where(p => p.Blog = blog).OrderBy(p => p.PublishedDate).Take(25)
.Select(p => new SyndicationItem(p.Title, p.Content, new Uri(p.Url)));
var feed = new SyndicationFeed(blog.Title, blog.Description, new Uri(blog.Url) , postItems) {
Copyright = blog.Copyright,
Language = "en-US"
};
return new FeedResult(new Rss20FeedFormatter(feed));
}
This also has a few additional advantages:
1. Unit tests can ensure the ActionResult is a FeedResult
2. Unit tests can examine the Feed property to examine results without parsing XML
3. Switching to Atom format involved just changing the new Rss20FeedFormatter to Atom10FeedFormatter.
HostForLIFE.eu ASP.NET MVC 6 Hosting
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
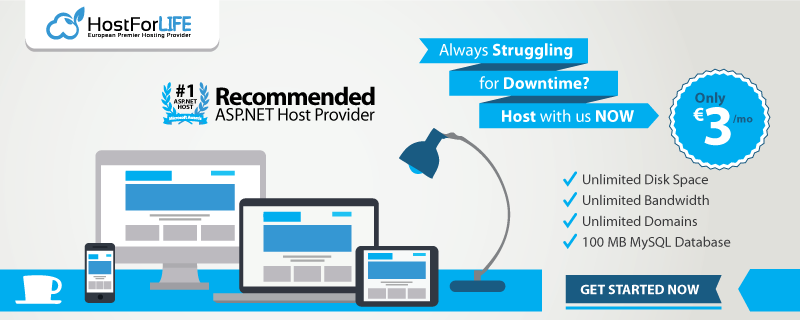