
April 9, 2015 08:11 by
Scott
In this article I will disclose about How to show Multiple Models in a Single View utilizing dynamically created object in ASP.NET MVC. Assume I have two models, Course and Student, and I have to show a list of courses and students within a single view. By what means would we be able to do this? And here is the code that I used:
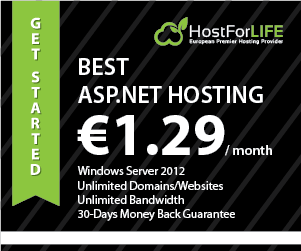
Model(Student.cs)
using System;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace MVCMultipleModelsinView.Models
{
public class Student
{
public int studentID { get; set; }
public string studentName { get; set; }
public string EnrollmentNo { get; set; }
public string courseName { get; set; }
public List<Student> GetStudents()
{
List<Student> students = new List<Student>();
students.Add(new Student { studentID = 1, studentName = "Peter", EnrollmentNo = "K0001", courseName ="ASP.NET"});
students.Add(new Student { studentID = 2, studentName = "Scott", EnrollmentNo = "K0002", courseName = ".NET MVC" });
students.Add(new Student { studentID = 3, studentName = "Rebecca", EnrollmentNo = "K0003", courseName = "SQL Server" });
return students;
}
}
}
Model(Course.cs):
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace MVCMultipleModelsinView.Models
{
public class Course
{
public int courseID { get; set; }
public string courseCode { get; set; }
public string courseName { get; set; }
public List<Course> GetCourses()
{
List<Course> Courses = new List<Course>();
Courses.Add(new Course { courseID = 1, courseCode = "CNET", courseName = "ASP.NET" });
Courses.Add(new Course { courseID = 2, courseCode = "CMVC", courseName = ".NET MVC" });
Courses.Add(new Course { courseID = 3, courseCode = "CSVR", courseName = "SQL Server" });
return Courses;
}
}
}
Controller (CourseStudentController.cs):
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using System.Dynamic;
using MVCMultipleModelsinView.Models;
namespace MVCMultipleModelsinView.Controllers
{
public class CourseStudentController : Controller
{
public ActionResult Index()
{
ViewBag.Message = "Welcome!";
dynamic model = new ExpandoObject();
Student stu = new Student();
model.students = stu.GetStudents();
Course crs = new Course();
model.courses = crs.GetCourses();
return View(model);
}
}
}
View(Index.cshtml):
@using MVCMultipleModelsinView.Models;
@{
ViewBag.Title = "Index";
}
<h2>@ViewBag.Message</h2>
<style type="text/css">
table
{
margin: 4px;
border-collapse: collapse;
width: 500px;
font-family:Tahoma;
}
th
{
background-color: #990000;
font-weight: bold;
color: White !important;
}
table th a
{
color: White;
text-decoration: none;
}
table th, table td
{
border: 1px solid black;
padding: 5px;
}
</style>
<p>
<b>Student List</b></p>
<table>
<tr>
<th>
Student Id
</th>
<th>
Student Name
</th>
<th>
Course Name
</th>
<th>
Enrollment No
</th>
</tr>
@foreach (Student stu in Model.students)
{
<tr>
<td>@stu.studentID
</td>
<td>@stu.studentName
</td>
<td>@stu.courseName
</td>
<td>@stu.EnrollmentNo
</td>
</tr>
}
</table>
<p>
<b>Course List</b></p>
<table>
<tr>
<th>
Course Id
</th>
<th>
Course Code
</th>
<th>
Course Name
</th>
</tr>
@foreach (Course crs in Model.courses)
{
<tr>
<td>@crs.courseID
</td>
<td>@crs.courseCode
</td>
<td>@crs.courseName
</tr>
}
</table>
HostForLIFE.eu ASP.NET MVC 6.0 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
