
January 22, 2020 10:19 by
Peter
This article will solve a problem. Display modes in ASP.NET MVC 5 provide a way of separating page content from the way it is rendered on various devices, like web, mobile, iPhone, iPod and Windows Phones. All you need to do is to define a display mode for each device, or class of devices.
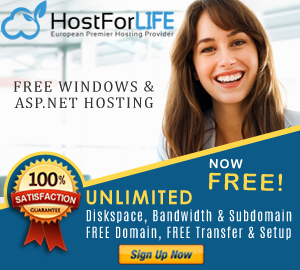
First you create a model and context class. We create an Employee class that has the following properties like.
public class Employee
{
public Guid ID { get; set; }
[Display(Name="First Name")]
public string FirstName { get; set; }
[Display(Name = "Last Name")]
public string LastName { get; set; }
[Display(Name = "Department")]
public string Department { get; set; }
[Display(Name = "Salary")]
public double Salary { get; set; }
[Display(Name = "Address")]
public string Address { get; set; }
}
And second is the context class like this that inherits the DbContext class.
public class DBConnectionContext:DbContext
{
public DBConnectionContext(): base("name=dbContext")
{
Database.SetInitializer(new DropCreateDatabaseIfModelChanges
<DBConnectionContext>());
}
public DbSet<Employee> Employees { get; set; }
}
If you want to recreate data every time the model changes, add these lines of code.
Database.SetInitializer(new DropCreateDatabaseIfModelChanges<DbConnectionContext>());
You also have a web config file. We configure connectionStrings in the Web.Config file.
<connectionStrings>
<add name="dbContext" connectionString="Data Source=localhost; Initial Catalog=CommonDataBase; Integrated Security=true"
providerName="System.Data.SqlClient" />
</connectionStrings>
Then you create a controller class in a controller folder and edit the name as HomeController. Add a Scaffold to select a MVC 5 Controller with Views, using Entity Framework.
DisplayModes
DisplayModes give you another level of flexibility on top of the default capabilities we saw in the last section. DisplayModes can also be used along with the previous feature so we will simply build off of the site we just created. Let's say we wanted to show an alternate view for the Windows Phone 8, iPhone, iPod or Android.
Windows Phone 8 DisplayMode
Now that you have made the override files, you can use a DisplayMode to show them for the appropriate phones.
The best time to set this up is when the application starts. Here is our global.asax.cs, with the DisplayMode setup.
DisplayModeProvider.Instance.Modes.Insert(1, new DefaultDisplayMode("WP")
{
ContextCondition = (ctx => ctx.GetOverriddenUserAgent()
.IndexOf("Windows Phone OS", StringComparison.OrdinalIgnoreCase) > 0)
});
DisplayModeProvider.Instance.Modes.Insert(1, new DefaultDisplayMode("iPhone")
{
ContextCondition = (ctx => ctx.GetOverriddenUserAgent()
.IndexOf("iPhone", StringComparison.OrdinalIgnoreCase) > 0)
});
DisplayModeProvider.Instance.Modes.Insert(1, new DefaultDisplayMode("Android")
{
ContextCondition = (ctx => ctx.GetOverriddenUserAgent()
.IndexOf("Android", StringComparison.OrdinalIgnoreCase) > 0)
});
The DisplayModeProvider Class
DisplayModeProvider holds a list of DefaultDisplayMode obejects, each representing display mode. And the provider holds the two display modes, default and mobile. The default display mode in an empty string and the second holds the mobile string.
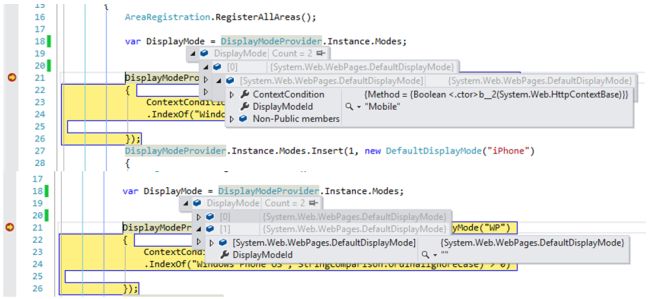
We just create multiple View with [View].Android.cshtml, [View].iPhone.cshtml and so on for every device such as:
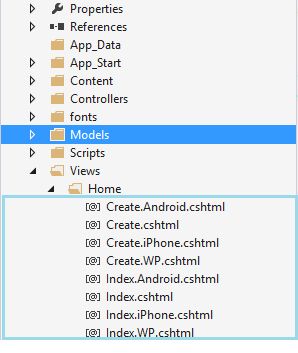
We create an index for iPhone and create a new employee in iPhone Index.iPhone.cshtml and Create.iPhone.cshtml.
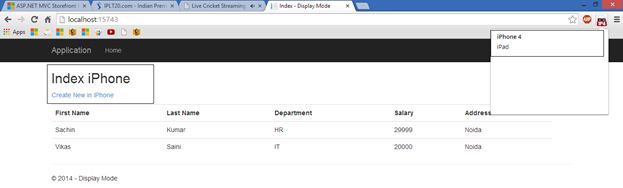
We create an index for Windows Phone and create a new employee in the Windows Phone Index.WP.cshtml Create.WP.cshtml.
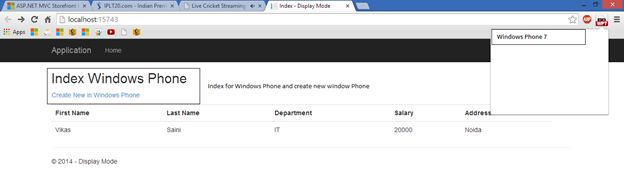
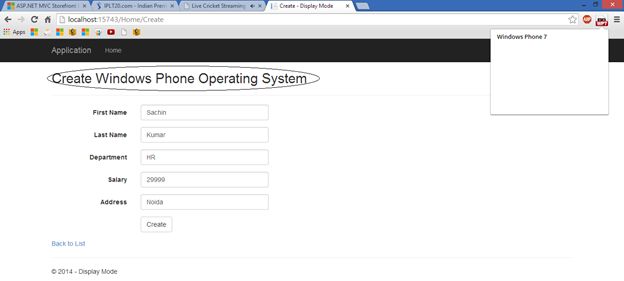
We create an index for Android and create a new employee in Windows Phone Index.Android.cshtml Create.Android.cshtml.
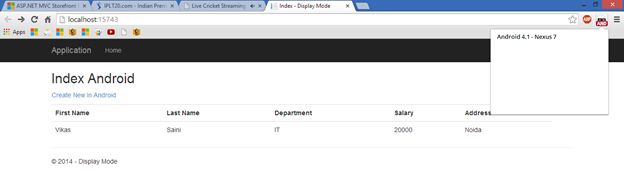
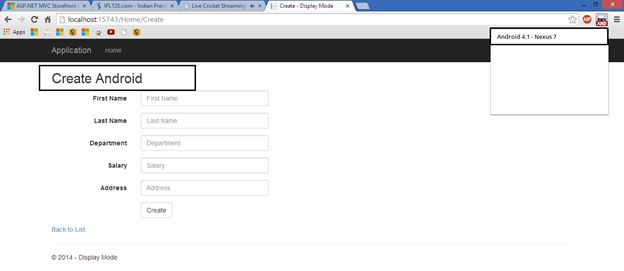