Cross-site scripting (XSS) is a type of computer security vulnerability typically found in web applications. XSS enables attackers to inject client-side script into web pages viewed by other users. Cross-Site Scripting (XSS) attacks are a type of injection, in which malicious scripts are injected into otherwise trusted web sites. In this article, I will show you how to avoid XSS while allowing only the HTML that you want to accept. In example, only <b> and <u> tags.
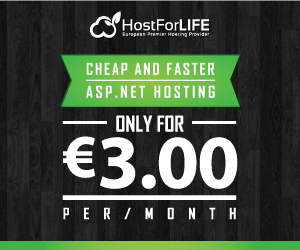
Firstly, let's filter the user input, and accept only <b></b> and <u></u> tags.
Step 1: Disables input validation
Step 2: Encodes all the input that is coming from the user
Step 3: Replace the encoded html with the HTML elements that you want to allow
Here is the full code snippet:
[HttpPost]
// Input validation is disabled, so the users can submit HTML
[ValidateInput(false)]
public ActionResult Create(Comment comment)
{
StringBuilder sbComments = new StringBuilder();
// Encode the text that is coming from comments textbox
sbComments.Append(HttpUtility.HtmlEncode(comment.Comments));
// Only decode bold and underline tags
sbComments.Replace("<b>", "<b>");
sbComments.Replace("</b>", "</b>");
sbComments.Replace("<u>", "<u>");
sbComments.Replace("</u>", "</u>");
comment.Comments = sbComments.ToString();
// HTML encode the text that is coming from name textbox
string strEncodedName = HttpUtility.HtmlEncode(comment.Name);
comment.Name = strEncodedName;
if (ModelState.IsValid)
{
db.Comments.AddObject(comment);
db.SaveChanges();
return RedirectToAction("Index");
}
return View(comment);
}
This is just one example. Only filtering the user input can't guarantee XSS elimination. XSS can happen in different ways and forms.
Hope you did it!
HostForLIFE.eu ASP.NET MVC 6 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
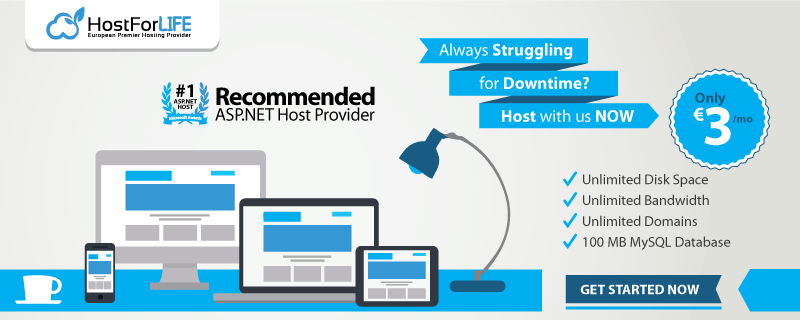