In this tutorial, you’re going to learn how to add video using Entity Framework. We will be building a form for adding a new video to the database.Step 1: Creating a Page to Add a Video
Before we get started, let’s take a closer look at routing.
Earlier I told you that the routing engine determines the name of the controller and the action from the URL of the request. The default rule (or the default convention) targets a URL with the pattern /controller/action/id. Here both action and id are optional. If action is not specified, Index is assumed as the action name.

For the purpose of this section, we need a new page for the user to add a new video. To do this, we’re going to create a new action that responds to a URL like /videos/new. Inside this action, we’ll return a view which will include a data entry form for adding a video.
First, go to VideosController and create a new action like this:
public ActionResult New()
{
return View();
}
All we do here is simply return a view. Let’s create the corresponding view. In Solution Explorer, expand the Views folder, right-click the Videos folder, and go to Add > View…. Set the name of the view to New and make sure _Layout.cshtml is selected as the layout (similar to the last section).

Next, set the model behind this view on the top:
@model Beaver.Models.Video
We set the model to the Video because we’re going to capture a Video object in this form.
@using (Html.BeginForm("Add", "Videos", FormMethod.Post, new { @class = "form" }))
{
}
Here we are using Razor syntax (note the @) to write C# code. Html.BeginForm is a helper method, which we use to render an HTML form element. It returns an instance of MvcForm, which is a disposable object. By wrapping it inside a using block, we can ensure that it will be properly disposed.
The first and second arguments specify the name of the action and controller that will be called when we post this form. In this case, we expect an action named Add in our VideosController to be called. We haven’t created this action yet, but we’ll do so soon.
The third argument specifies the form method. In HTML forms, we have two methods: GET and POST. When sending data for modification to the server, we should always use the POST method.
The last argument is an anonymous object ( new {} ) that specifies any additional attributes to add to the HTML markup. So, when this code is executed, the view engine will render something like this:
<form action=”/videos/add” method=”post” class=”form”>
</form>
We use the form CSS class to give a nice, modern look to our forms. This CSS class is defined in Bootstrap, which is a CSS framework for building modern and responsive (mobile- and tablet-friendly) web applications. When you create an ASP.NET MVC project in Visual Studio, Bootstrap is automatically added for you.
We added the form. Now, we need to add three input fields in this form: Title, Description and Genre. Write this code inside the using block:Now, write the following code in the view to create an HTML form element:
<div class="form-group">
@Html.LabelFor(m => m.Title)
@Html.TextBoxFor(m => m.Title, new { @class = "form-control" })
</div>
<div class="form-group">
@Html.LabelFor(m => m.Description)
@Html.TextAreaFor(m => m.Description, new { @class = "form-control", rows = 4 })
</div>
<div class="form-group">
@Html.LabelFor(m => m.Genre)
@Html.EnumDropDownListFor(m => m.Genre, new { @class = "form-control" })
</div>
Here we have three sections, each wrapped with a div with a form-group class. Again, this is one of the classes that is defined in Bootstrap. If you follow Botostrap’s markup, you always get a nice, clean form that renders well both on the desktop and on mobile devices.
Inside each form-group, we have a label and an input field. Look at the first form-group.
<div class="form-group">
@Html.LabelFor(m => m.Title)
@Html.TextBoxFor(m => m.Title, new { @class = "form-control" })
</div>
We’re using Html.LabelFor to render a label for the Title property of the Video class.
@Html.LabelFor(m => m.Title)
The expression m => m.Title is a lambda expression that we use to access a property in the model of this view. If you’re not familiar with lambda expressions, check out my C# Advanced course on Udemy. When this line is executed by the view engine, we’ll get an HTML markup like this:
<label for=”Title”>Title</label>
Next, we use Html.TextBoxFor helper method to render a text box.
@Html.TextBoxFor(m => m.Title, new { @class = "form-control" })
Again, we use a lambda expression to specify the target property. Also, note that the second argument to this method is an anonymous object that specifies any additional attributes to add to the markup, in this case form-control CSS class. This is another Bootstrap class that gives our text boxes a bit of padding, round corners and a nice effect when the input is in focus. The result will be HTML markup like this:
<input type=”text” id=”Title” name=”Title” class=”form-control”></input>
Now you read the second form-group.
<div class="form-group">
@Html.LabelFor(m => m.Description)
@Html.TextAreaFor(m => m.Description, new { @class = "form-control", rows = 4 })
</div>
It’s very similar to the first one, except that here we use the Html.TextAreaFor helper method to render a textarea input element instead of a text box. This allows the user to write a description that is more than one line.
And finally, in the third form-group, we use a different helper method (Html.EnumDropDownListFor) to render a drop-down list for the Genre property, which is an enum:
<div class="form-group">
@Html.LabelFor(m => m.Genre)
@Html.EnumDropDownListFor(m => m.Genre, new { @class = "form-control" })
</div>
For the last step, we need a button to submit the form. Add this markup inside the using block, after all form groups:
<input type="submit" class="btn btn-primary" value="Save" />
Here, btn and btn-primary are both Bootstrap classes that make our buttons look cool and modern.
Your entire using block should look like this:
@using (Html.BeginForm("Add", "Videos", FormMethod.Post, new { @class = "form" }))
{
<div class="form-group">
@Html.LabelFor(m => m.Title)
@Html.TextBoxFor(m => m.Title, new { @class = "form-control" })
</div>
<div class="form-group">
@Html.LabelFor(m => m.Description)
@Html.TextAreaFor(m => m.Description, new { @class = "form-control", rows = 4 })
</div>
<div class="form-group">
@Html.LabelFor(m => m.Genre)
@Html.EnumDropDownListFor(m => m.Genre, new { @class = "form-control" })
</div>
<input type="submit" class="btn btn-primary" value="Save" />
}
Now it’s time to review what we have done. Run the application with Ctrl+F5 and look at the form we’ve built:

Note how the input fields and the button look. This is all because of the beautiful styles defined in Bootstrap.
In this step, we added a new action to our controller that returned a view. We created a view with a form to add a video.
HostForLIFE.eu ASP.NET MVC 5 Hosting
HostForLIFE.eu revolutionized hosting with Plesk Control Panel, a Web-based interface that provides customers with 24x7 access to their server and site configuration tools. Plesk completes requests in seconds. It is included free with each hosting account. Renowned for its comprehensive functionality - beyond other hosting control panels - and ease of use, Plesk Control Panel is available only to HostForLIFE's customers. They offer a highly redundant, carrier-class architecture, designed around the needs of shared hosting customers.
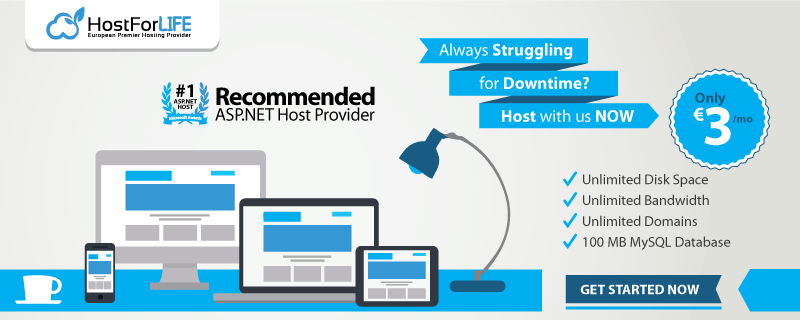