
December 30, 2020 12:17 by
Peter
As part of this article, we will learn about ASP.Net MVC filters, their different types and we will create a custom log Action Filter using Visual Studio. So without wasting any time let's start.
What are ASP.Net MVC filters
In an ASP.Net MVC Web application, we have action methods defined in controllers that call from different views. For example, when the user clicks on some button on the view, a request goes to the designated controller and then the corresponding action method. Within this flow, sometimes we want to perform logic either before an action method is called or after an action method runs.
To fulfill the above condition ASP.Net MVC provides us filters. Filters are the custom classes that provide us with an option to add pre-action and post-action behavior to controller action methods.
ASP.NET MVC framework supports four different types of filters:
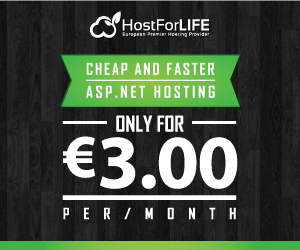
- Authorization filters
- Action filters
- Result filters
- Exception filters
Note
They are executed in the order listed above.
Authorization filters
In ASP.NET MVC web application by default, all the action methods of all the controllers can be accessible for all the users (for both authenticated and anonymous users both). For this, if we want to restrict some action methods from anonymous users or we want to allow the action methods only to an authenticated user, then you need to use the Authorization Filter in MVC.
The Authorization Filter provides two built-in attributes such as Authorize and AllowAnonymous. We can decorate our action methods with the Authorize attribute which allows access to the only authenticated user and we can use AllowAnonymous attribute to allow some action method to all users.
We can also create custom Authorization filters for this we have to implement IAuthenticationFilter interface and have overrides its OnAuthentication() and OnAuthenticationChallenge(). We are going deep into it.
Action filters
In the ASP.NET MVC web application, action filters are used to perform some logic before and after action methods. The output cache is one of the built-in action filters which we can use in our action methods.
[OutputCache(Duration=100)]
public ActionResult Index()
{
return View();
}
We can also create a custom action filter either by implementing IActionFilter interface and FilterAttribute class or by deriving the ActionFilterAttribute abstract class. As part of this article, I am covering creating a custom action filter using the ActionFilterAttribute abstract class.
ActionFilterAttribute includes the following method to override it:
void OnActionExecuted(ActionExecutedContext filterContext)
void OnActionExecuting(ActionExecutingContext filterContext)
void OnResultExecuted(ResultExecutedContext filterContext)
void OnResultExecuting(ResultExecutingContext filterContext)
We will see this in detail below.
Result filters
In an ASP.NET MVC web application, result filters are used to perform some logic before and after a view result is executed. For example, we might want to modify a view result right before the view is rendered.
Exception filters
In the ASP.NET MVC web application, we can use an exception filter to handle errors raised by either our controller actions or controller action results. We also can use exception filters to log errors.
Creating a Log Action Filter
Open Visual Studio and create a new ASP.NET Web Application
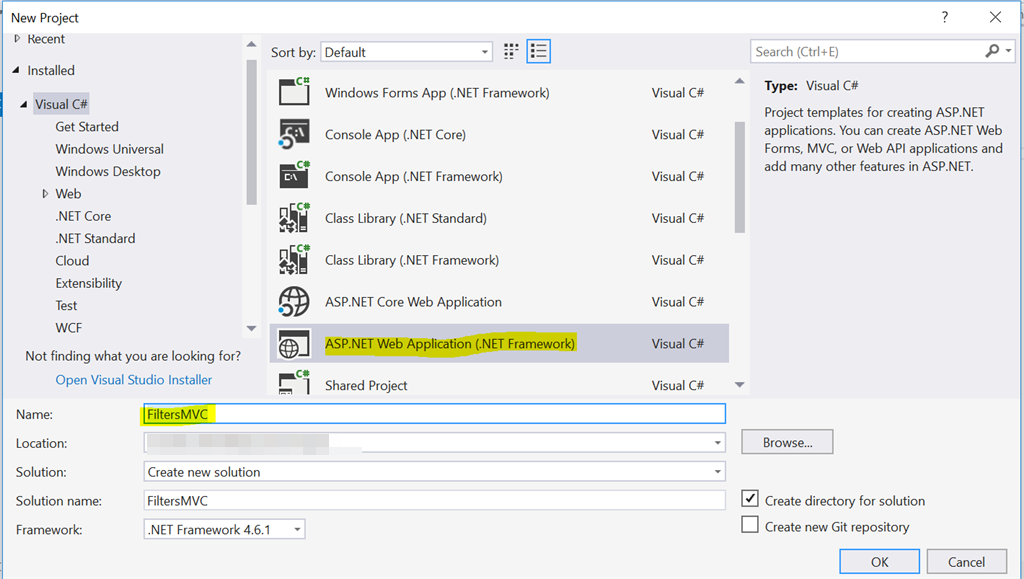
Choose Empty, select “MVC” and then click the OK button.
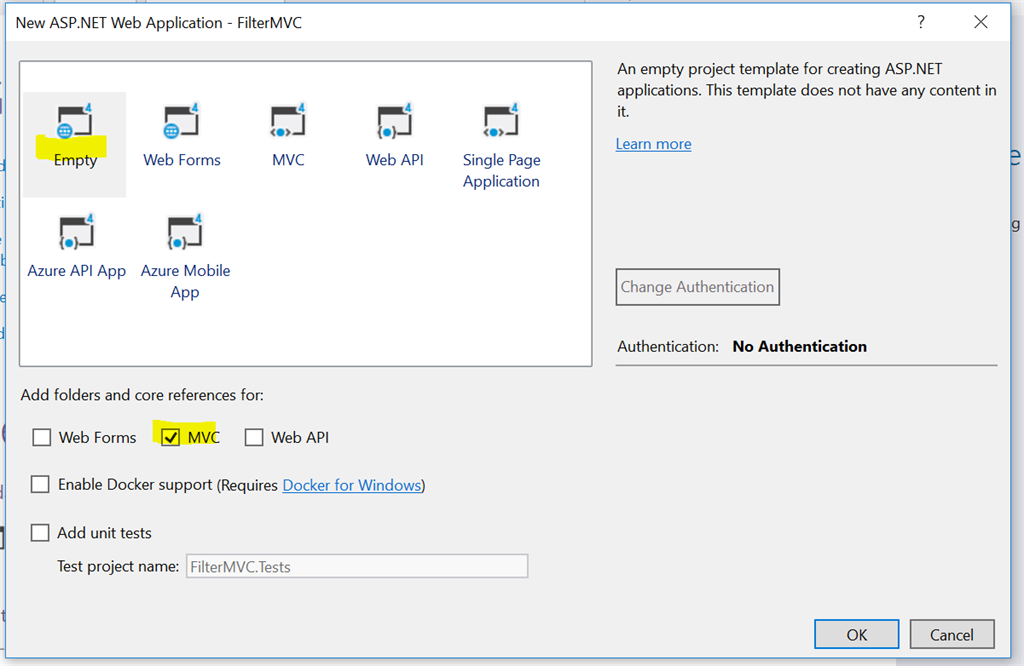
Add a ‘Filters’ folder and create a new class LogActionFilter.cs under it:
using System.Diagnostics;
using System.Web.Mvc;
using System.Web.Routing;
namespace FiltersMVC.Filters
{
public class LogActionFilter : ActionFilterAttribute
{
public override void OnActionExecuting(ActionExecutingContext filterContext)
{
Log("OnActionExecuting", filterContext.RouteData);
}
public override void OnActionExecuted(ActionExecutedContext filterContext)
{
Log("OnActionExecuted", filterContext.RouteData);
}
public override void OnResultExecuting(ResultExecutingContext filterContext)
{
Log("OnResultExecuting", filterContext.RouteData);
}
public override void OnResultExecuted(ResultExecutedContext filterContext)
{
Log("OnResultExecuted", filterContext.RouteData);
}
private void Log(string methodName, RouteData routeData)
{
var controllerName = routeData.Values["controller"];
var actionName = routeData.Values["action"];
var message = $"{methodName} controller:{controllerName} action:{actionName}";
Debug.WriteLine(message, "Action Filter Log");
}
}
}
We overrides OnActionExecuting(), OnActionExecuted(), OnResultExecuting(), and OnResultExecuted() methods all are calling the Log() method. The name of the method and the current route data is passed to the Log() method. The Log() method writes a message to the Visual Studio Output window.
Now, add HomeController.cs under the Controllers folder.
using FiltersMVC.Filters;
using System.Web.Mvc;
namespace FiltersMVC.Controllers
{
[LogActionFilter]
public class HomeController : Controller
{
// GET: Home
public ActionResult Index()
{
return View();
}
}
}
After adding the above files our folder structure will look like this:
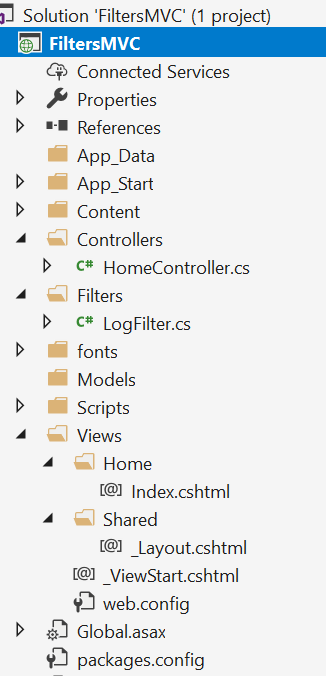
Now run the application, we can see the results in the “Output” window.
