
March 20, 2020 12:00 by
Peter
Partial view in ASP.NET MVC is special view which renders a portion of view content. It is just like a user control of a web form application. Partial can be reusable in multiple views. It helps us to reduce code duplication. In other word a partial view enables us to render a view within the parent view.
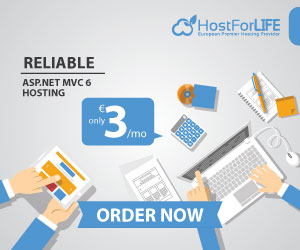
The partial view is instantiated with its own copy of a ViewDataDictionary object which is available with the parent view so that partial view can access the data of the parent view. If we made the change in this data (ViewDataDictionary object), the parent view's data is not affected. Generally the Partial rendering method of the view is used when related data that we want to render in a partial view is part of our model.
Creating Partial View
To create a partial view, right-click on view -> shared folder and select Add -> View option. In this way we can add a partial view.
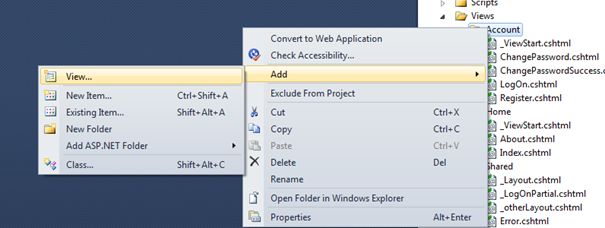
Creating Partial View
It is not mandatory to create a partial view in a shared folder but a partial view is mostly used as a reusable component, it is a good practice to put it in the "shared" folder.
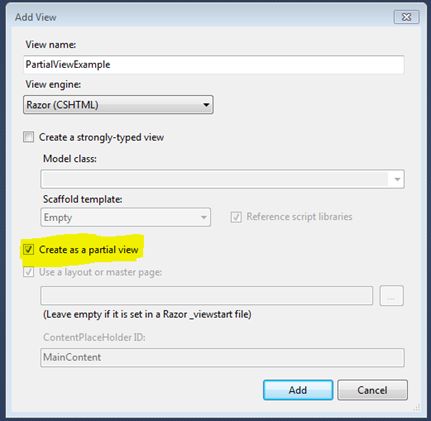
HTML helper has two methods for rendering the partial view: Partial and RenderPartial.
<div>
@Html.Partial("PartialViewExample")
</div>
<div>
@{
Html.RenderPartial("PartialViewExample");
}
</div>
@Html.RenderPartial
The result of the RenderPartial method is written directly into the HTTP response, it means that this method used the same TextWriter object as used by the current view. This method returns nothing.
@Html.Partial
This method renders the view as an HTML-encoded string. We can store the method result in a string variable.
The Html.RenderPartial method writes output directly to the HTTP response stream so it is slightly faster than the Html.Partial method.
Returning a Partial view from the Controller's Action method:
public ActionResult PartialViewExample()
{
return PartialView();
}
Render Partial View Using jQuery
Sometimes we need to load a partial view within a model popup at runtime, in this case we can render the partial view using JQuery element's load method.
<script type="text/jscript">
$('#partialView').load('/shared/PartialViewExample’);
</script>