In this article we will tell you How to Create Header Checkbox in web Gridview with ASP.NET MVC 6. You need to Simply Change in this Example is that in Index.cshtml document.
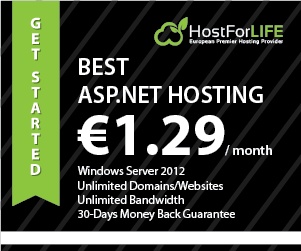
I used the following code to show the Header Checkbox:
columns: grid.Columns(
grid.Column(header: "{CheckBoxHeading}", format:
@<text><input class="box" type="checkbox" /></text>),
grid.Column("ID", canSort: true, style: "Id"),
grid.Column("Name", canSort: true, style: "Name"),
grid.Column("Question", canSort: true, style: "Question"),
grid.Column("", header: "Actions", format: @<text>
@Html.ActionLink("Edit", "Edit", new { id=item.Id} )
@Html.ActionLink("Delete", "Delete", new { id=item.Id} )
</text>
)
).ToString().Replace("{CheckBoxHeading}", "<input type='checkbox' id='allBox'/>")
In which we additionally give Facility to user to choose multiple record at once focused around checkbox selection. When client click on Header checkbox then it will automatically check all the checkbox means select All row or record.
if the GridView contain large number of Record at that point user wish to delete all record then user have needed more time to pick each {and every|and every} record by click on each row checkbox, thus for that reason we offer a facility to user simply click on one Header CheckBox and choose the all row or Record in Gridview.
If you have previous Example, then simply copy this code and paste it in Index.CSHtml file:
Index.CSHtml File
@model IEnumerable<finalpaging.Models.poll>
@{
ViewBag.Title = "Index";
}
<!DOCTYPE html>
<html>
<head>
<title>WebgridSample</title>
<script src="../../Scripts/jquery-1.7.1.min.js" type="text/javascript"></script>
<script type="text/javascript">
//Select or Deselect all checkboxs in the grid
$(document).ready(function () {
$("#allBox").click(function () {
$(".box").attr("checked", $(this).attr("checked") ? true : false);
});
});
</script>
<style type="text/css">
.webGrid { margin: 4px; border-collapse: collapse; width: 500px; background-color:#FCFCFC;} .header { background-color: #C1D4E6; font-weight: bold; color: #FFF; }
.webGrid th, .webGrid td { border: 1px solid #C0C0C0; padding: 5px; }
.alt { background-color: #E4E9F5; color: #000; }
.gridHead a:hover {text-decoration:underline;}
.description { width:auto}
.select{background-color: #389DF5}
</style>
</head>
<body>
<p>
@Html.ActionLink("Create New", "Create")
</p>
@{
finalpaging.Models.poll product = new finalpaging.Models.poll();
}
@{
var grid = new WebGrid(Model, canPage: true, rowsPerPage: 10, selectionFieldName: "selectedRow",ajaxUpdateContainerId: "gridContent");
grid.Pager(WebGridPagerModes.NextPrevious);}
<div id="gridContent">
MvcHtmlString.Create(grid.GetHtml(tableStyle: "webGrid",
headerStyle: "header",
alternatingRowStyle: "alt",
selectedRowStyle: "select",
fillEmptyRows: false,
footerStyle: "footer",
mode: WebGridPagerModes.All,
firstText: "<< First",
previousText: "< Prev",
nextText: "Next >",
lastText: "Last >>",
columns: grid.Columns(
grid.Column(header: "{CheckBoxHeading}", format:
@<text><input class="box" type="checkbox" /></text>),
grid.Column("ID", canSort: true, style: "Id"),
grid.Column("Name", canSort: true, style: "Name"),
grid.Column("Question", canSort: true, style: "Question"),
grid.Column("", header: "Actions", format: @<text>
@Html.ActionLink("Edit", "Edit", new { id=item.Id} )
@Html.ActionLink("Delete", "Delete", new { id=item.Id} )
</text>
)
)).ToString().Replace("{CheckBoxHeading}", "<input type='checkbox' id='allBox'/>")
),
</div>
</body>
</html>
I hope this tutorial works for you!
HostForLIFE.eu ASP.NET MVC 6 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
