
October 27, 2020 10:19 by
Peter
In this blog post, I'll discuss a useful yet easy to implement document viewer API. Using that you can display a source file (e.g. Word, Presentation, Diagram, PSD) from your server to your browser without downloading it.
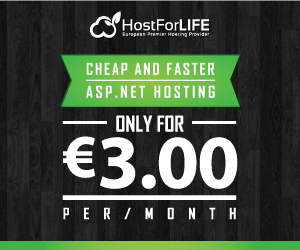
Some Use-Cases
Display a Word resume to a user in a web browser
Render a Visio Diagram on the web page
View a Presentation or a Slide online without downloading it
Implementation
Now as the use-cases are clear, we will dive into the API implementation. It'll be an ASP.NET MVC application. We'll pull data/documents from the database and save it to a stream. You have to specify the file format in order to render it correctly. The source document will be then rendered to HTML. Eventually, our controller will return this stream to the View/browser.
public ActionResult Index()
{
License lic = new License();
lic.SetLicense(@"D:/GD Licenses/Conholdate.Total.NET.lic");
MemoryStream outputStream = new MemoryStream();
//specify just the file name if you are pulling the data from database
string fileName = "sample.pdf";
FileType fileType = FileType.FromExtension(Path.GetExtension(fileName));
using (Viewer viewer = new Viewer(() => GetSourceFileStream(fileName), () => new LoadOptions(fileType)))
{
HtmlViewOptions Options = HtmlViewOptions.ForEmbeddedResources(
(pageNumber) => outputStream,
(pageNumber, pageStream) => { });
viewer.View(Options);
}
outputStream.Position = 0;
return File(outputStream, "text/html");
}
private Stream GetSourceFileStream(string fileName) =>
new MemoryStream(GetSourceFileBytesFromDb(fileName));
//TODO: If you want to pull the data from the DB
private byte[] GetSourceFileBytesFromDb(string fileName) =>
System.IO.File.ReadAllBytes(fileName);
Have a look at this image/screenshot. We displayed a PDF from Server to Browser.
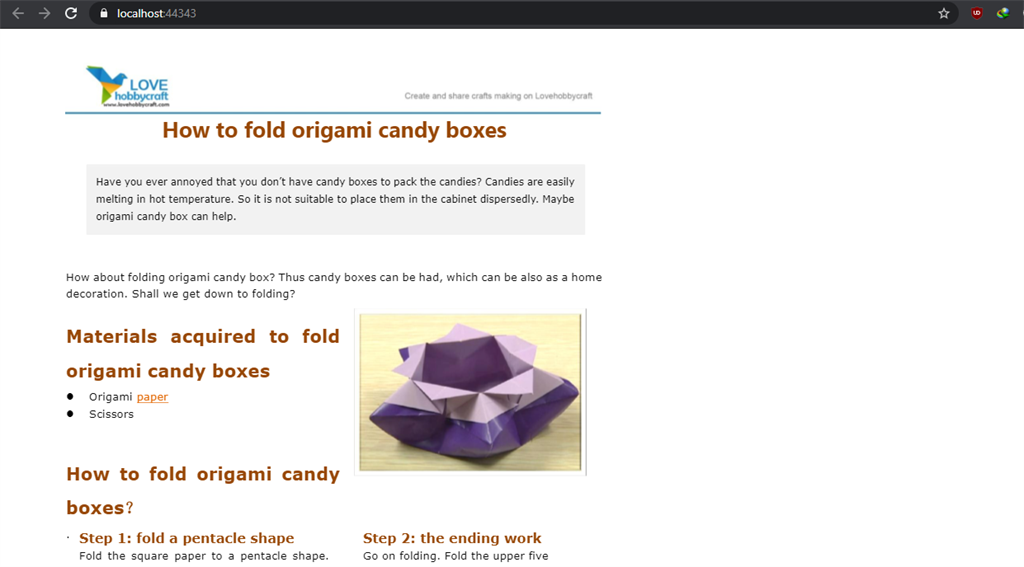

October 23, 2020 09:44 by
Peter
ViewBag, ViewData, and TempData all are objects in ASP.NET MVC and these are used to pass the data in various scenarios.
The following are the scenarios where we can use these objects.
Pass the data from Controller to View.
Pass the data from one action to another action in the same Controller.
Pass the data in between Controllers.
Pass the data between consecutive requests.
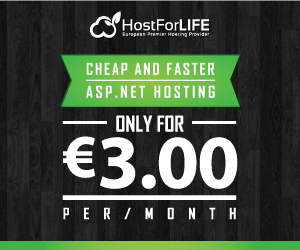
ViewBag
ViewBag is a dynamic object to pass the data from Controller to View. And, this will pass the data as a property of object ViewBag. And we have no need to typecast to read the data or for null checking. The scope of ViewBag is permitted to the current request and the value of ViewBag will become null while redirecting.
Ex Controller
Public ActionResult Index()
{
ViewBag.Title = “Welcome”;
return View();
}
View
<h2>@ViewBag.Title</h2>
ViewData
ViewData is a dictionary object to pass the data from Controller to View where data is passed in the form of key-value pair. And typecasting is required to read the data in View if the data is complex and we need to ensure null check to avoid null exceptions. The scope of ViewData is similar to ViewBag and it is restricted to the current request and the value of ViewData will become null while redirecting.
Ex
Controller:
Public ActionResult Index()
{
ViewData[”Title”] = “Welcome”;
return View();
}
View
<h2>@ViewData[“Title”]</h2>
TempData
TempData is a dictionary object to pass the data from one action to other action in the same Controller or different Controllers. Usually, TempData object will be stored in a session object. Tempdata is also required to typecast and for null checking before reading data from it. TempData scope is limited to the next request and if we want Tempdata to be available even further, we should use Keep and peek.
Ex - Controller
Public ActionResult Index()
{
TempData[”Data”] = “I am from Index action”;
return View();
}
Public string Get()
{
return TempData[”Data”] ;
}
To summarize, ViewBag and ViewData are used to pass the data from Controller action to View and TempData is used to pass the data from action to another action or one Controller to another Controller.
Hope you have understood the concept of ViewBag, ViewData, and TempData.