
October 27, 2020 10:19 by
Peter
In this blog post, I'll discuss a useful yet easy to implement document viewer API. Using that you can display a source file (e.g. Word, Presentation, Diagram, PSD) from your server to your browser without downloading it.
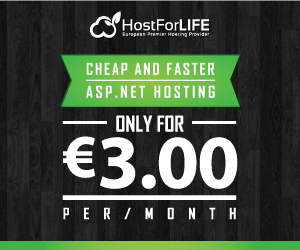
Some Use-Cases
Display a Word resume to a user in a web browser
Render a Visio Diagram on the web page
View a Presentation or a Slide online without downloading it
Implementation
Now as the use-cases are clear, we will dive into the API implementation. It'll be an ASP.NET MVC application. We'll pull data/documents from the database and save it to a stream. You have to specify the file format in order to render it correctly. The source document will be then rendered to HTML. Eventually, our controller will return this stream to the View/browser.
public ActionResult Index()
{
License lic = new License();
lic.SetLicense(@"D:/GD Licenses/Conholdate.Total.NET.lic");
MemoryStream outputStream = new MemoryStream();
//specify just the file name if you are pulling the data from database
string fileName = "sample.pdf";
FileType fileType = FileType.FromExtension(Path.GetExtension(fileName));
using (Viewer viewer = new Viewer(() => GetSourceFileStream(fileName), () => new LoadOptions(fileType)))
{
HtmlViewOptions Options = HtmlViewOptions.ForEmbeddedResources(
(pageNumber) => outputStream,
(pageNumber, pageStream) => { });
viewer.View(Options);
}
outputStream.Position = 0;
return File(outputStream, "text/html");
}
private Stream GetSourceFileStream(string fileName) =>
new MemoryStream(GetSourceFileBytesFromDb(fileName));
//TODO: If you want to pull the data from the DB
private byte[] GetSourceFileBytesFromDb(string fileName) =>
System.IO.File.ReadAllBytes(fileName);
Have a look at this image/screenshot. We displayed a PDF from Server to Browser.
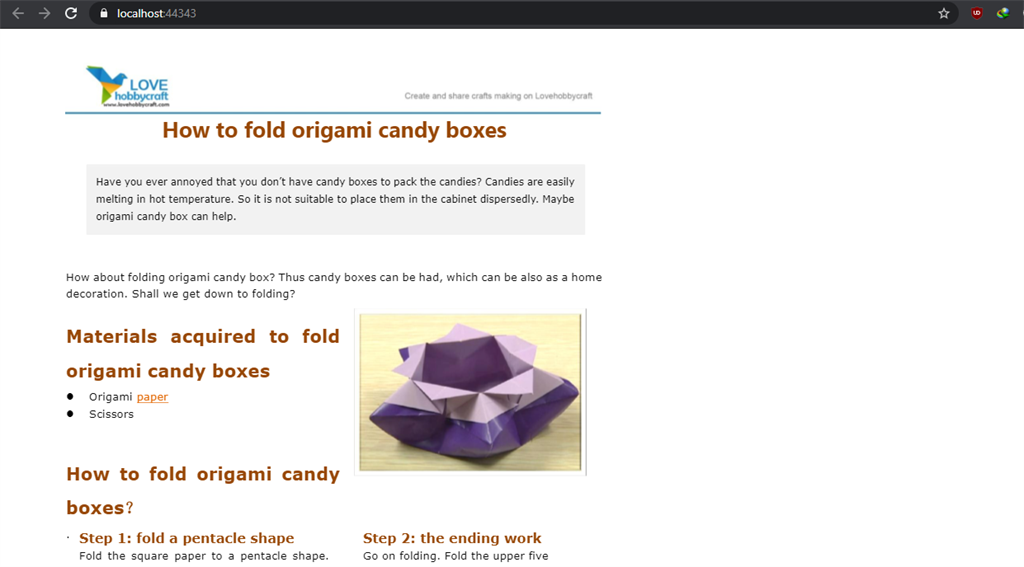