ASP.NET MVC action methods are responsible to execute the request and generate a response to it. All the public methods of the MVC Controller are action methods. If we want the public method to be a non-action method, then we can decorate the action method by “NonAction” attribute. It will restrict the action method to render on the browser.
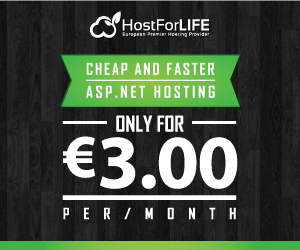
To work with action method we need to remember the following points.
- The action method is the public non-static method.
- Action method can not be the private or protected method.
- Action method can not contain ref and out parameters.
- Action method can not be an extension method.
- Action method can not be overloaded.
Example of an action method in ASP.NET MVC 5 is as below.
public class HomeController : Controller
{
// GET: Home
public ActionResult Index()
{
return View();
}
}
You can see in the above action method example that the Index() method is a public method which is inside the HomeController class. And the return type of the action method is ActionResult. It can return using the “View()” method. So, every public method inside the Controller is an action method in MVC.
Action Method can not be a private or protected method.
public class HomeController : Controller
{
// GET: Home
private ActionResult Index()
{
return "This is Index Method";
}
}
If you provide the private or protected access modifier to the action method, it will provide the error to the user, i.e., “resource can not be found” as below.
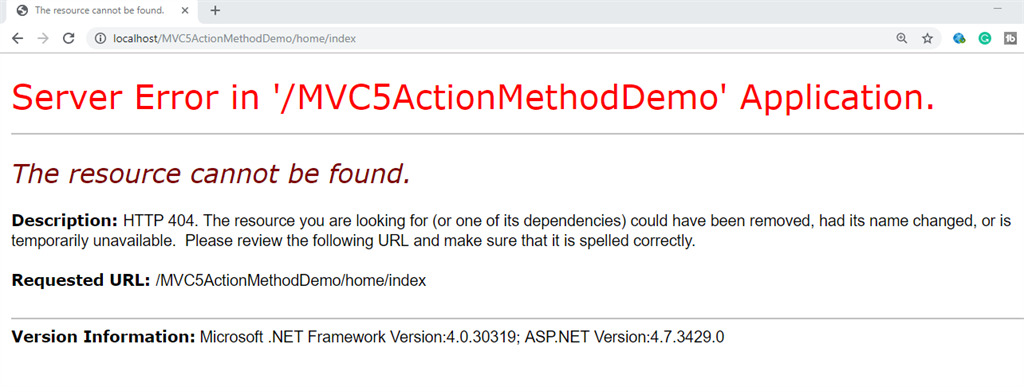
Action method can not be overloaded.
public string Index()
{
return "This is Index Method";
}
public string Index(int id)
{
return "This is Index overloaded Method";
}
If you try to overload the action method, then it will throw an ambiguous error.
An action method cannot contain ref and out parameters.
public string Index(ref int id)
{
return "This is Index Method" +id;
}
public string Index(out int id)
{
id = 0;
return "This is Index Method" +id;
}
We can not provide the ref and out parameters to the action method. If you try to provide the ref and out parameters to the action method, then it will throw an error as below.
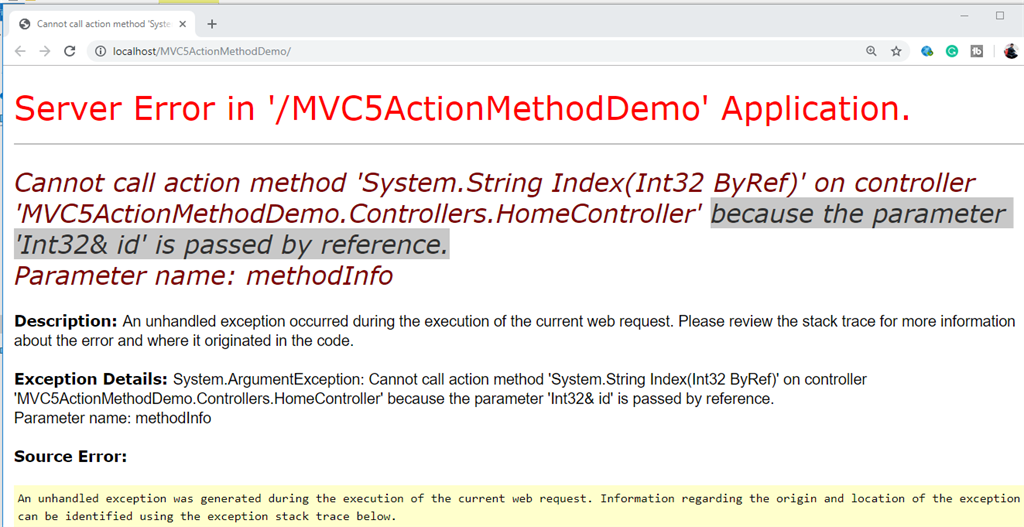
Action method can not be overloaded.
public string Index()
{
return "This is Index Method";
}
public string Index(int id)
{
return "This is Index overloaded Method";
}
If you try to overload the action method, then it will throw an ambiguous error.
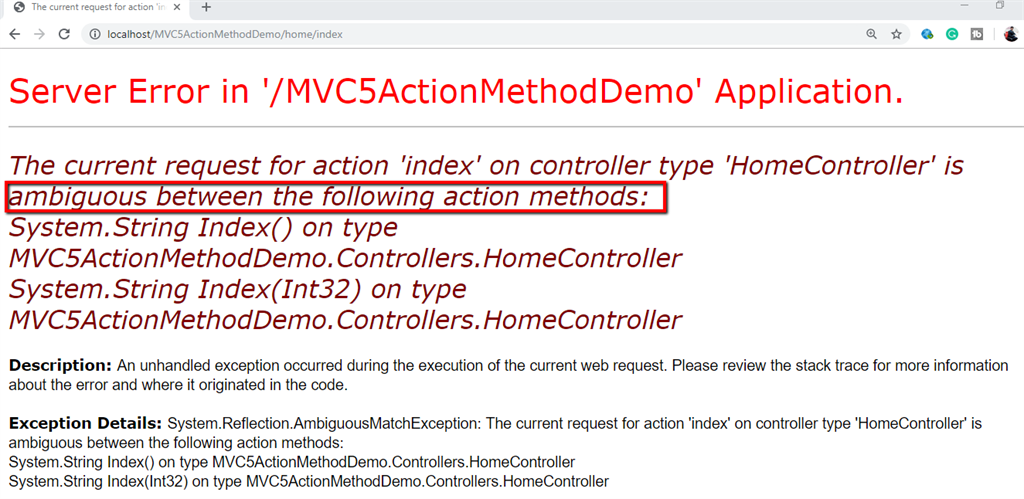
Types of Action Method.
- Action Method
- Non Action Method
How to restrict the public action method in MVC?
To restrict the public action method in MVC, we can use the “NonAction” attribute. The “NonAction” attribute exists in the “System.Web.MVC” namespace.
Example of NonAction attribute in ASP.NET MVC.
[NonAction]
public string GetFullName(string strFirstName, string strLastName)
{
return strFirstName + " " + strLastName;
}
How to call Action Method?
Syntax
YourDomainName/ControllerName/ActionMethodName
Example
localhost/Home/Index

How to change default Action Method?
The default Action method is configured in the RouteConfig.cs class. By default, the Action Method is the “Index” action method. Change the “Index” action method name as per our requirement.
public static void RegisterRoutes(RouteCollection routes)
{
routes.IgnoreRoute("{resource}.axd/{*pathInfo}");
routes.MapRoute(
name: "Default",
url: "{controller}/{action}/{id}",
defaults: new { controller = "Home", action = "Message", id = UrlParameter.Optional }
);
}
You can see in the above code that we have changed the action method name to “Message” instead of “Index” by default.
How does the default action get executed?
Default controller and action method are configured in RouteConfig.cs class. By default, Controller is the Home Controller and default Action Method is the “Index” action method.
Example of default action method
public static void RegisterRoutes(RouteCollection routes)
{
routes.IgnoreRoute("{resource}.axd/{*pathInfo}");
routes.MapRoute(
name: "Default",
url: "{controller}/{action}/{id}",
defaults: new { controller = "Home", action = "Index", id = UrlParameter.Optional }
);
}