
September 26, 2019 11:14 by
Peter
What is Cross-Site Request Forgery
Cross-Site Request Forgery (CSRF) is a process in which a user first signs on to a genuine website (e.g. facebook.com) and after successful login, the user opens another website (malicious website) in the same browser.
Both websites are opened in the same browser. Malicious website will display some links to the user and asks the user to click on those links. User clicks on the links displayed on a malicious website, the malicious website sends a request using the existing session of genuine website. Web server of genuine website treats this request as a valid request and assumes that it is coming from a valid user so it executes the request and provides a proper response. A malicious website can perform harmful operations on genuine website.
In order to solve this problem, we expect the Action Method of genuine website to recognize the source of the request, whether the request is coming from genuine website or from a malicious website. This can be achieved by using the [ValidateAntiForgeryToken] attribute in ASP.Net MVC.
How to Implement CSRF Security in MVC
In order to implement CSRF security in MVC, first, we need to use HTML helper @Html.AntiForgeryToken() in view. It should be placed inside the BeginForm() method in view.
Next, we need to add [ValidateAntiForgeryToken] attribute on the action method which will accept HTTP post request. We need to do only these 2 changes and now MVC will prevent CSRF attacks.
How ValidateAntiForgeryToken works
How ValidateAntiForgeryToken prevents CSRF attacks?
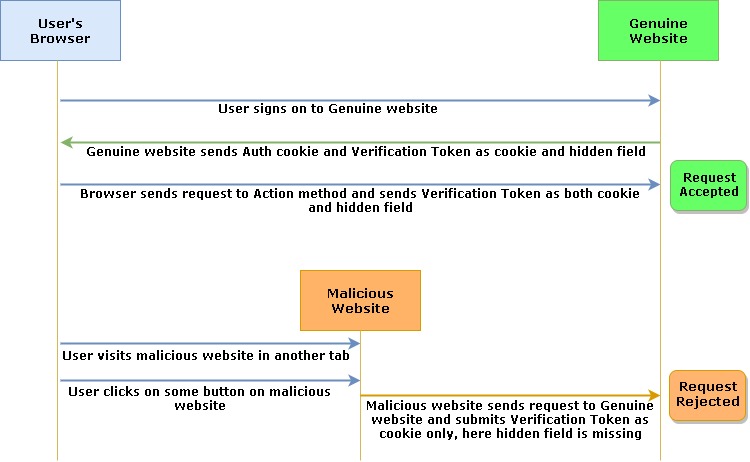
First, the user will open genuine website in the browser. User login to genuine website. After login, genuine website sends Authentication cookie and Verification token. Verification token has randomly generated alphanumeric values. The verification token is stored in the cookie as well as in hidden field on client-side. When HTML form is submitted to the server, the verification token is submitted as a cookie as well as a hidden field. On the server-side, both are checked if they are same or not? If both are same then request is valid. If they are different or one of them is missing then request is treated as invalid request and will be rejected automatically by MVC.
When a user opens a malicious website in a new tab of the same browser, the malicious website will display some links and ask the user to click on those links. This website already has a script to send the request to genuine website. When the user clicks on links, the malicious website sends a request to genuine website. Since request is being sent to genuine website, the browser automatically submits Authentication cookie to Action method of genuine website but here, the hidden field is missing. The Action method has [ValidateAntiForgeryToken] attribute so it checks whether Authentication cookie and hidden field has same value but here, the hidden field is missing so the request is treated as invalid and it is rejected by MVC.
Practical Implementation
Wherever you have a Form, use @Html.AntiForgeryToken() inside the form and action method for accepting the HTTP Post should have ValidateAntiForgeryToken attribute. These are the only change, the rest of the process will be taken care of by ASP.Net MVC.
Please see the below HTML view where I have [email protected]()
@using (Html.BeginForm("Create", "Products", FormMethod.Post, new { enctype = "multipart/form-data" }))
{
@Html.AntiForgeryToken()
<div class="form-row">
<div class="form-group col-md-6">
@Html.LabelFor(temp => temp.ProductName)
@Html.TextBoxFor(temp => temp.ProductName, new { placeholder = "Product Name", @class = "form-control" })
@Html.ValidationMessageFor(temp => temp.ProductName)
</div>
<div class="form-group col-md-6">
@Html.LabelFor(temp=>temp.Price)
@Html.TextBoxFor(temp=>temp.Price,new { @class="form-control",placeholder="Price"})
</div>
</div>
@Html.ValidationSummary()
<button type="submit" class="btn btn-success">Create</button>
<a class="btn btn-danger" href="/products/index">Cancel</a>
}
Below is the action method where I have added [ValidateAntiForgeryToken] attribute.
[HttpPost]
[ValidateAntiForgeryToken]
public ActionResult Create(Product p)
{
ProductDBContext db = new ProductDBContext();
if (ModelState.IsValid)
{
db.Products.Add(p);
db.SaveChanges();
return RedirectToAction("Index");
}
else
{
return View();
}
}
Summary
In this blog, I have explained Cross-Site Request Forgery(CSRF), its steps and what changes we need to do in MVC application to prevent CSRF attacks.
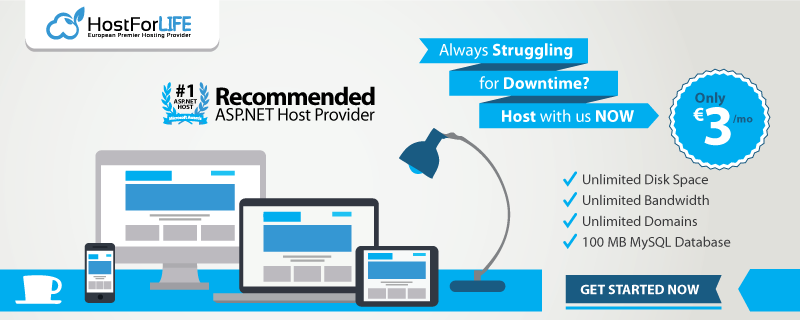

September 18, 2019 12:47 by
Peter
In this post, we will see how to render given below bootstrap Tab script in Asp.net MVC 5 view.
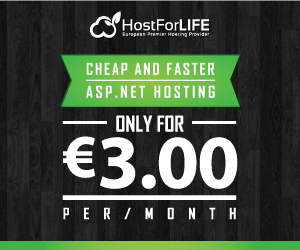
Here the number of tabs displayed in view would depend on count of members in the list.
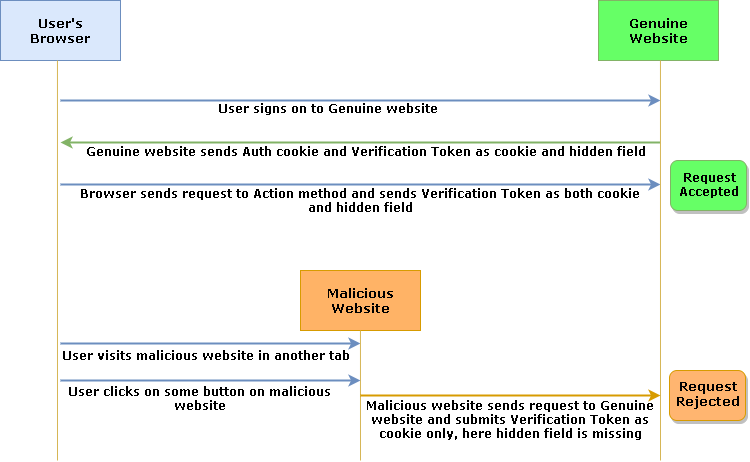
1. Create ASP.NET MVC 5 project.
2. Define Member class as follows in “Member.cs” file
public class Member
{
public int Id { get; set; }
public string Name { get; set; }
}
3. Add view to create action method and pass a list of members from controller Action method to razor view file. i.e. “create.cshtml”
public ActionResult Create()
{
List<Member> members = new List<Member>();
members = GetMemberList();
return View(members);
}
private List<Member> GetMemberList()
{
List<Member> members = new List<Member>();
members.Add(new Member{ Name = "member1" });
members.Add(new Member { Name = "member2" });
members.Add(new Member { Name = "member3" });
members.Add(new Member { Name = "member4" });
members.Add(new Member { Name = "member5" });
return members;
}
4. Using CSS classes, we can create a tabscript in our “create.cshtml” as shown below:
@model IEnumerable<Member>
<div class="container">
@if (Model.ToList().Count > 0)
{
<ul class="nav nav-tabs" role="tablist">
@{int i = 0;
foreach (var item in Model)
{
if (i == 0)
{
<li class="nav-item">
<a class="nav-link active" data-toggle="tab" href="#@item.Name">@item.Name</a>
</li>
}
else
{
<li class="nav-item">
<a class="nav-link" data-toggle="tab" href="#@item.Name">@item.Name</a>
</li>
}
i++;
}
}
</ul>
<div class="tab-content">
@foreach (var item in Model)
{
<div id="@item.Name" class="container tab-pane active">
<br>
<h3>@item.Name 's Tab Area </h3>
</div>
}
</div>
}
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.7/umd/popper.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.min.js"></script>

September 13, 2019 11:51 by
Peter
jQuery UI has an AutoComplete widget. The AutoComplete widget is quite nice and straight forward to use. In this post, I will show you how to use jQuery AutoComplete widget to consolidate AutoComplete function in ASP.NET MVC application.
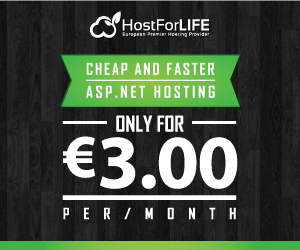
Step 1
The first step is to add the jQuery scripts and styles. With ASP.NET MVC, the following code does the work:
@Styles.Render("~/Content/themes/base/css")
@Scripts.Render("~/bundles/jquery")
@Scripts.Render("~/bundles/jqueryui")
Step 2
Using the AutoComplete widget is also simple. You will have to add a textbox and attach the AutoComplete widget to the textbox. The only parameter that is required for the widget to function is source. For this example, we will get the data for the AutoComplete functionality from a MVC action method.
$(document).ready(function () {
$('#tags').autocomplete(
{
source: '@Url.Action("TagSearch", "Home")'
});
})
In the above code, the textbox with id=tags is attached with the AutoComplete widget. The source points to the URL of TagSearch action in the HomeController: /Home/TagSearch. The HTML of the textbox is below:
<input type="text" id="tags" />
Step 3
When the user types some text in the textbox, the action method (TagSearch) is called with a parameter in the request body. The parameter name is term. So, your action method should have the following signature:
public ActionResult TagSearch(string term)
{
// Get Tags from database
string[] tags = { "ASP.NET", "WebForms",
"MVC", "jQuery", "ActionResult",
"MangoDB", "Java", "Windows" };
return this.Json(tags.Where(t => t.StartsWith(term)),
JsonRequestBehavior.AllowGet);
}
Now, the AutoComplete functionality is complete!
HostForLIFE.eu ASP.NET MVC 6 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
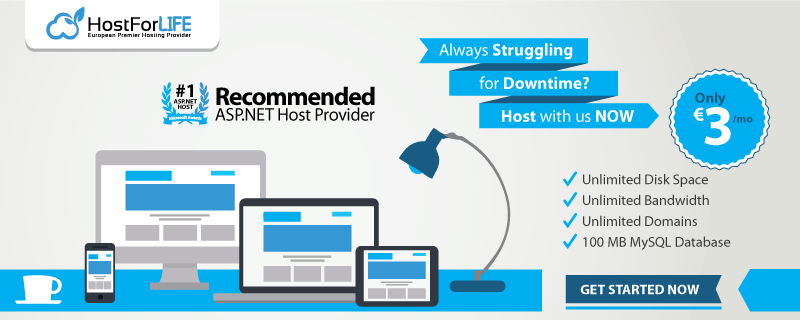

September 6, 2019 12:18 by
Peter
Basic use of session in ASP.NET MVC is as follows:
- Check user is logged in or not
- to carry authorization data of user logged in
- to carry temporary alternative data
- Check session Timeout on user action for every controller
Using asynchronous (AJAX) request it's a very common state of affairs to use jquery AJAX or unobtrusive AJAX API of ASP.NET MVC 5 to create asynchronous request in MVC 5. Each jquery AJAX and unobtrusive AJAX square measure very powerful to handle asynchronous mechanism. however in most things, we like better to use jquery AJAX to possess fine tuned control over the application. And currently suppose we wish to see the session timeout for every asynchronous call in our application. we are using JSON to grab an information on form and send it with asynchronous request. Therefore we dropped in situation where:
1. Normal direct won’t work well
RedirectToAction("LoginView", "LoginController");
2. We need to ascertain session timeout in action technique. A repetitive code in every action method therefore reduced code reusability and maintainability.
if (session.IsNewSession || Session["LoginUser"] == null) { //redirection logic
We can use base controller class or take advantage of action filters of ASP.NET MVC 5. using base controller class and preponderant OnActionExecuting methodology of Controller class:
public class MyBaseController : Controller
{
protected override void OnActionExecuting(ActionExecutingContext filterContext)
{
HttpSessionStateBase session = filterContext.HttpContext.Session;
if (session.IsNewSession || Session["LoginUser"] == null)
{
filterContext.Result = Json("Session Timeout", "text/html");
}
}
}
And inheriting Base Class:
public class MyController : BaseController
{
//your action methods…
}
Limitation of this approach is that it covers up every action method.Using action filter attribute class of ASP.NET MVC 5. Therefore we will fine tune every controller action as needed.
[AttributeUsage(AttributeTargets.Class |
AttributeTargets.Method, Inherited = true, AllowMultiple = true)]
public class SessionTimeoutFilterAttribute : ActionFilterAttribute
{
public override void OnActionExecuting(ActionExecutingContext filterContext)
{
HttpSessionStateBase session = filterContext.HttpContext.Session;
// If the browser session or authentication session has expired
if (session.IsNewSession || Session["LoginUser"] == null)
{
if (filterContext.HttpContext.Request.IsAjaxRequest())
{
JsonResult result = Json("SessionTimeout", "text/html");
filterContext.Result = result;
}
else
{
// For round-trip requests,
filterContext.Result = new RedirectToRouteResult(
new RouteValueDictionary {
{ "Controller", "Accounts" },
{ "Action", "Login" }
});
}
}
base.OnActionExecuting(filterContext);
}
}
Jquery code at client side
$.ajax({
type: "POST",
url: "controller/action",
contentType: "application/json; charset=utf-8",
dataType: "json",
data: JSON.stringify(data),
async: true,
complete: function (xhr, status) {
if (xhr.responseJSON == CONST_SESSIONTIMEOUT) {
RedirectToLogin(true);
return false;
}
if (status == 'error' || !xhr.responseText) {
alert(xhr.statusText);
}
}
});
}