
September 26, 2019 11:14 by
Peter
What is Cross-Site Request Forgery
Cross-Site Request Forgery (CSRF) is a process in which a user first signs on to a genuine website (e.g. facebook.com) and after successful login, the user opens another website (malicious website) in the same browser.
Both websites are opened in the same browser. Malicious website will display some links to the user and asks the user to click on those links. User clicks on the links displayed on a malicious website, the malicious website sends a request using the existing session of genuine website. Web server of genuine website treats this request as a valid request and assumes that it is coming from a valid user so it executes the request and provides a proper response. A malicious website can perform harmful operations on genuine website.
In order to solve this problem, we expect the Action Method of genuine website to recognize the source of the request, whether the request is coming from genuine website or from a malicious website. This can be achieved by using the [ValidateAntiForgeryToken] attribute in ASP.Net MVC.
How to Implement CSRF Security in MVC
In order to implement CSRF security in MVC, first, we need to use HTML helper @Html.AntiForgeryToken() in view. It should be placed inside the BeginForm() method in view.
Next, we need to add [ValidateAntiForgeryToken] attribute on the action method which will accept HTTP post request. We need to do only these 2 changes and now MVC will prevent CSRF attacks.
How ValidateAntiForgeryToken works
How ValidateAntiForgeryToken prevents CSRF attacks?
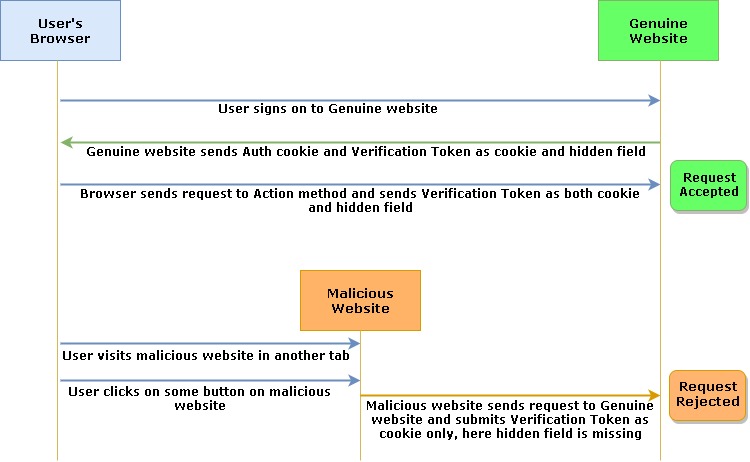
First, the user will open genuine website in the browser. User login to genuine website. After login, genuine website sends Authentication cookie and Verification token. Verification token has randomly generated alphanumeric values. The verification token is stored in the cookie as well as in hidden field on client-side. When HTML form is submitted to the server, the verification token is submitted as a cookie as well as a hidden field. On the server-side, both are checked if they are same or not? If both are same then request is valid. If they are different or one of them is missing then request is treated as invalid request and will be rejected automatically by MVC.
When a user opens a malicious website in a new tab of the same browser, the malicious website will display some links and ask the user to click on those links. This website already has a script to send the request to genuine website. When the user clicks on links, the malicious website sends a request to genuine website. Since request is being sent to genuine website, the browser automatically submits Authentication cookie to Action method of genuine website but here, the hidden field is missing. The Action method has [ValidateAntiForgeryToken] attribute so it checks whether Authentication cookie and hidden field has same value but here, the hidden field is missing so the request is treated as invalid and it is rejected by MVC.
Practical Implementation
Wherever you have a Form, use @Html.AntiForgeryToken() inside the form and action method for accepting the HTTP Post should have ValidateAntiForgeryToken attribute. These are the only change, the rest of the process will be taken care of by ASP.Net MVC.
Please see the below HTML view where I have [email protected]()
@using (Html.BeginForm("Create", "Products", FormMethod.Post, new { enctype = "multipart/form-data" }))
{
@Html.AntiForgeryToken()
<div class="form-row">
<div class="form-group col-md-6">
@Html.LabelFor(temp => temp.ProductName)
@Html.TextBoxFor(temp => temp.ProductName, new { placeholder = "Product Name", @class = "form-control" })
@Html.ValidationMessageFor(temp => temp.ProductName)
</div>
<div class="form-group col-md-6">
@Html.LabelFor(temp=>temp.Price)
@Html.TextBoxFor(temp=>temp.Price,new { @class="form-control",placeholder="Price"})
</div>
</div>
@Html.ValidationSummary()
<button type="submit" class="btn btn-success">Create</button>
<a class="btn btn-danger" href="/products/index">Cancel</a>
}
Below is the action method where I have added [ValidateAntiForgeryToken] attribute.
[HttpPost]
[ValidateAntiForgeryToken]
public ActionResult Create(Product p)
{
ProductDBContext db = new ProductDBContext();
if (ModelState.IsValid)
{
db.Products.Add(p);
db.SaveChanges();
return RedirectToAction("Index");
}
else
{
return View();
}
}
Summary
In this blog, I have explained Cross-Site Request Forgery(CSRF), its steps and what changes we need to do in MVC application to prevent CSRF attacks.
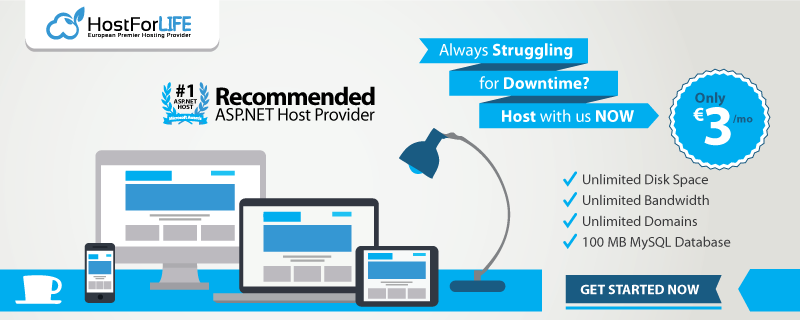