Here in this tutorial, I will explain about how to use Google reCAPTCHA in ASP.NET MVC.
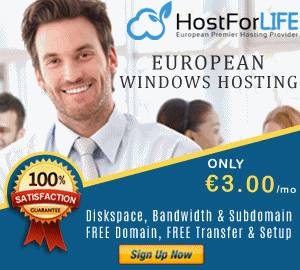
What is reCAPTCHA? reCAPTCHA protects the websites you love from spam and abuse. Google has updated thier reCAPTCHA API to 2.0 . Now, users can now attest they are human without having to solve a CAPTCHA. Instead with just a single click they’ll confirm they are not a robot and it is called as “No CAPTCHA reCAPTCHA“. This is how new reCAPTCHA look as follows:
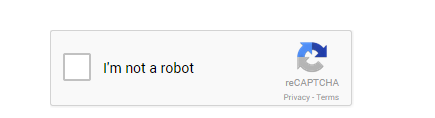
Getting Google ReCAPTCHA
Now, lets create an API key pair for your site at https://www.google.com/recaptcha/intro/index.html and click on Get reCAPTCHA at top of the page and follow the below steps to create an application.
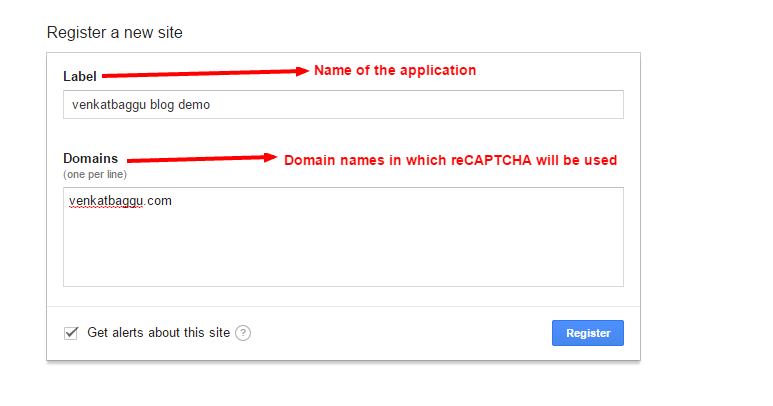
Once you have done with registration, the following keys will be generated:

- Site key : is used to display the widget in your page or code.
- Secret key: can be used as communication between your site and Google to verify the user response whether the reCAPTCHA is valid or not.
The next is to display the reCAPTCHA widget in your site.
Displaying Widget
We can render the Google reCAPTCHA widget in two ways as:
- Automatically render the widget
- Explicitly render the widget
For this example we will use Automatically render the widget in your page.
First load the script reference:
<script src="https://www.google.com/recaptcha/api.js" async defer></script>
Now, you need generate a DIV element with class name as g-recaptcha and your site key in the data-site key attribute in your webpage to generate reCAPTCHA.
<div class="g-recaptcha" data-sitekey="your_site_key"></div>
Now, implement above code in Home/Index.cshtml view page.
<div class="row">
<div class="col-md-12">
<form action="/home/validatecaptcha" method="POST">
<div class="g-recaptcha" data-sitekey="6LfiS_8SAAAAABvF6ixcyP5MtsevE5RZ9dTorUWr"></div>
<br />
<input type="submit" value="Submit">
</form>
</div>
</div>
@section scripts{
<script src="https://www.google.com/recaptcha/api.js" async defer></script>
}
Verifying User Response
Once reCAPTCHA is generated and solved by a end user, a field with g-recaptcha-response will be populated in the html. When ever user submit the form on your site, you can POST the parameter g-recaptcha-response to verify the user response. The following API url is used to verify the user response.
https://www.google.com/recaptcha/api/siteverify?secret=your_secret&response=response_string&remoteip=user_ip_address
In above API url the secret and response parameters are required and where as remoteip is optional. Here secret represents the Secret Key that was generated in the key pair and the repsonse is the g-recaptcha-response that was submitted during the form post. The following is the API JSON response object that we get once the response is submitted.
{
"success": true|false,
"error-codes": [...] // optional
}
Next, you will create an response class to verify the user response:
public class CaptchaResponse
{
[JsonProperty("success")]
public bool Success { get; set; }
[JsonProperty("error-codes")]
public List<string> ErrorCodes { get; set; }
}
Then, you can create a POST method in Index action in the Home controller to verify the user response.
[HttpPost]
public ActionResult ValidateCaptcha()
{
var response = Request["g-recaptcha-response"];
//secret that was generated in key value pair
const string secret = "YOUR KEY VALUE PAIR";
var client = new WebClient();
var reply =
client.DownloadString(
string.Format("https://www.google.com/recaptcha/api/siteverify?secret={0}&response={1}", secret, response));
var captchaResponse = JsonConvert.DeserializeObject<CaptchaResponse>(reply);
//when response is false check for the error message
if (!captchaResponse.Success)
{
if (captchaResponse.ErrorCodes.Count <= 0) return View();
var error = captchaResponse.ErrorCodes[0].ToLower();
switch (error)
{
case ("missing-input-secret"):
ViewBag.Message = "The secret parameter is missing.";
break;
case ("invalid-input-secret"):
ViewBag.Message = "The secret parameter is invalid or malformed.";
break;
case ("missing-input-response"):
ViewBag.Message = "The response parameter is missing.";
break;
case ("invalid-input-response"):
ViewBag.Message = "The response parameter is invalid or malformed.";
break;
default:
ViewBag.Message = "Error occured. Please try again";
break;
}
}
else
{
ViewBag.Message = "Valid";
}
return View();
}
That's all! Hope it works for you!
HostForLIFE.eu ASP.NET MVC 6 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
