Model-View-Controller (MVC) is a design pattern that has been widely used in software engineering for creating well-structured and maintainable applications. In the context of .NET Core, MVC provides a robust framework for building web applications, separating concerns between the data (model), the user interface (view), and the business logic (controller).
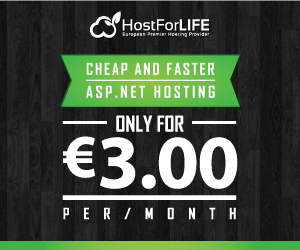
This article will delve into what MVC is, how it works in .NET Core, and how you can build a simple MVC application.
What is MVC?
MVC stands for Model-View-Controller, a pattern that divides an application into three interconnected components.
Model
- Represents the data and the business logic of the application.
- Directly manages the data, logic, and rules of the application.
- In .NET Core, the model can be an entity class that represents data in the database.
View
- Represents the presentation layer of the application.
- Responsible for displaying the data provided by the model in a user-friendly format.
- Views in .NET Core are typically Razor files (.cshtml) that generate HTML dynamically.
Controller
- Acts as an intermediary between the Model and the View.
- Handles user input, processes it using the model, and returns the appropriate view.
- In .NET Core, controllers are classes that inherit from the Controller and contain action methods.
How does MVC Work in .NET Core?
When a user interacts with an MVC application, the flow of control follows these steps.
User Request: The user sends a request, such as clicking a link or submitting a form.
Controller Action: The request is routed to a controller action method. The controller processes the request, interacts with the model if necessary, and selects a view to render.
View Rendering: The view receives the data from the controller and generates the HTML to be displayed to the user.
Response: The HTML generated by the view is sent back to the user's browser, where it is rendered.
Creating a Simple MVC Application in .NET Core
Let's walk through creating a simple MVC application in .NET Core.
Step 1. Setting Up the Project
You can start by creating a new MVC project in .NET Core using Visual Studio or the .NET CLI.
dotnet new mvc -n MvcDemoApp
This command creates a new MVC project named MvcDemoApp.
Step 2. Understanding the Project Structure
In an MVC project, you'll find three main folders.
- Models: Contains the classes that represent the application's data and business logic.
- Views: Contains the .cshtml files that define the HTML structure of the application.
- Controllers: Contains the controller classes that manage the flow of the application.
Step 3. Creating a Model
Let's create a simple model that represents a product.
namespace MvcDemoApp.Models
{
public class Product
{
public int Id { get; set; }
public string Name { get; set; }
public decimal Price { get; set; }
}
}
Step 4. Creating a ControllerNext, create a controller to handle requests related to products.
using Microsoft.AspNetCore.Mvc;
using MvcDemoApp.Models;
namespace MvcDemoApp.Controllers
{
public class ProductsController : Controller
{
public IActionResult Index()
{
var products = new List<Product>
{
new Product { Id = 1, Name = "Laptop", Price = 1200 },
new Product { Id = 2, Name = "Smartphone", Price = 800 }
};
return View(products);
}
}
}
The Index action method returns a list of products to the view.
Step 5. Creating a View
Finally, create a view to display the list of products. Add an Index.cshtml file under Views/Products.@model IEnumerable<MvcDemoApp.Models.Product>
<h2>Product List</h2>
<table class="table">
<thead>
<tr>
<th>Name</th>
<th>Price</th>
</tr>
</thead>
<tbody>
@foreach (var product in Model)
{
<tr>
<td>@product.Name</td>
<td>@product.Price</td>
</tr>
}
</tbody>
</table>
This view displays the product data in an HTML table.
Step 6. Running the ApplicationBenefits of Using MVC
- Separation of Concerns: MVC promotes a clear separation between the application's concerns, making it easier to manage and scale.
- Reusability: The separation of concerns allows for more reusable code components.
- Testability: The MVC pattern enhances the testability of your application by decoupling the components.
Conclusion
The MVC pattern in .NET Core provides a clean and organized way to develop web applications by separating the application into three core components: Model, View, and Controller. This separation makes your application more manageable, scalable, and testable. By following the steps outlined in this article, you can start building your own MVC applications in .NET Core and take advantage of the powerful features it offers.