
April 9, 2020 05:10 by
Peter
What is an Html Helper?
Html helper is a method that is used to render HTML content in a view. Html helpers are implemented using an extension method. If you want to create an input text box with
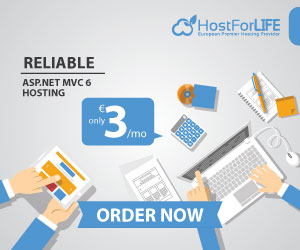
id=email and name in email:
<input type=text id=email name=email value=’’/>
This is all the Html we need to write -- by using the helper method it becomes so easy:
@Html.TextBox(‘email’)
It will generate a textbox control whose name is the email.
If we want to assign the value of the textbox with some initial value then use the below method:
@Html.TextBox(‘email’,’[email protected]’)
If I want to set an initial style for textbox we can achieve this by using the below way:
@Html.TextBox(‘email’,’[email protected]’,new {style=’your style here’ , title=’your title here’});
Here the style we pass is an anonymous type.
If we have a reserved keyword like class readonly and we want to use this as an attribute we will do this using the below method, which means append with @ symbol with the reserved word.
@Html.TextBox(‘email’,’[email protected]’,new {@class=’class name’, @readonly=true});
If we want to generate label:
@Html.Label(‘firstname’,’sagar’)
For password use the below Html helper method to create password box:
@Html.Password(“password”)
If I want to generate a textarea then for this also we have a method:
@Html.TextArea(“comments”,”,4,12,null)
In the above code 4 is the number of rows and 12 is the number of columns.
To generate a hidden box:
@Html.Hidden(“EmpID”)
Hidden textboxes are not displayed on the web page but used for storing data and when we need to pass data to action method then we can use that.
Is it possible to create our Html helpers in asp.net MVC?
Yes, we can create our Html helpers in MVC.
Is it mandatory to use Html helpers?
No, we can use plain Html for that but Html helpers reduce a significant amount of Html code to write that view.
Also, your code is simple and maintainable and if you require some complicated logic to generate view then this is also possible.