Assume you are creating a system for booking travel, and when a user books a car, two emails are sent.
- Service email: Sent with information about the reservation to the firm.
- Customer email: Submitted to the client as a reservation confirmation.
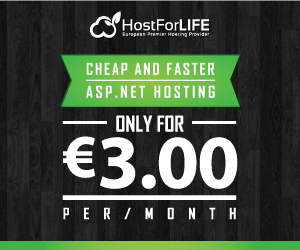
The use of the MailMessage and SmtpClient classes to do this is demonstrated in the C# code that follows.
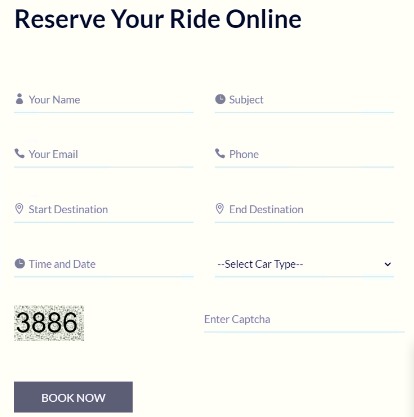
This article explains how to combine the HTML form that is provided, the C# backend mail-sending code, and AJAX capability that uses jQuery to transfer form data to the server. I've included the necessary cshtml code that includes validation, Ajax integration, and captcha features.
Code Breakdown
CSHTML with AJAX Integration
<div class="booking_content">
<h2>Reserve Your Ride Online</h2>
<form action="" method="post" id="booking_form" novalidate="novalidate" class="row booking_form">
<div class="col-md-6">
<div class="form-group">
<input type="text" class="form-control" name="name" placeholder=" Your Name">
<label class="border_line"></label>
</div>
</div>
<div class="col-md-6">
<div class="form-group">
<input type="text" class="form-control" name="subject" placeholder=" Subject">
<label class="border_line"></label>
</div>
</div>
<div class="col-md-6">
<div class="form-group">
<input type="text" class="form-control" name="email" placeholder=" Your Email">
<label class="border_line"></label>
</div>
</div>
<div class="col-md-6">
<div class="form-group">
<input type="text" class="form-control" name="mobile" placeholder=" Phone">
<label class="border_line"></label>
</div>
</div>
<div class="col-md-6">
<div class="form-group">
<input type="text" class="form-control" name="stratdestination" placeholder=" Start Destination">
<label class="border_line"></label>
</div>
</div>
<div class="col-md-6">
<div class="form-group">
<input type="text" class="form-control" name="endDestination" placeholder=" End Destination">
<label class="border_line"></label>
</div>
</div>
<div class="col-md-6">
<div class="form-group">
<input type="text" class="form-control date-input-css" name="timedate" placeholder=" Time and Date">
<label class="border_line"></label>
</div>
</div>
<div class="col-md-6">
<div class="form-group">
<select class="form-control" name="cartype">
<option>--Select Car Type--</option>
<option value="A/C Tata Indigo">A/C Tata Indigo</option>
<!-- Add other car types -->
</select>
<label class="border_line"></label>
</div>
</div>
<div class="col-sm-6 col-md-6">
<div class="form-group row">
<label class="col-sm-4 col-md-3 control-label">
<div id="divGenerateRandomValues"></div>
</label>
</div>
</div>
<div class="col-sm-6 col-md-6">
<div class="form-group row">
<input type="text" id="textInput" class="form-control" placeholder="Enter Captcha" />
<span class="errCap text-danger"></span>
</div>
</div>
<div class="col-lg-12">
<div class="form-group">
<button type="submit" value="submit" class="btn slider_btn dark_hover btnBookNow" id="btnSubmit">Book Now</button>
</div>
</div>
</form>
</div>
<!-- Modal -->
<div class="modal fade sccmodl" id="sccmodl" tabindex="1" role="dialog" aria-labelledby="exampleModalLabel" aria-hidden="true" data-backdrop="static" data-keyboard="false">
<div class="modal-dialog modal-dialog-centered" role="document">
<div class="modal-content">
<div class="modal-header">
<h5>Booking Information</h5>
<button type="button" class="close No" data-dismiss="modal" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
</div>
<div class="modal-body">
<h6 class="indxmsg"></h6>
</div>
<div class="modal-footer">
<input type="button" class="btn btn-info btn-sm btnOk" value="Ok" />
</div>
</div>
</div>
</div>
@section Scripts {
<script type="text/javascript">
var iNumber = Math.floor(1000 + Math.random() * 9000);
$(document).ready(function () {
$("#btnSubmit").prop("disabled", true);
$("#divGenerateRandomValues").html("<input id='txtNewInput' value='" + iNumber + "' disabled/>");
// Validate Captcha
$("#btnSubmit").click(function (e) {
e.preventDefault();
if ($("#textInput").val() != iNumber) {
$('.errCap').text('Invalid Captcha !');
} else {
$('.errCap').text('');
submitForm();
}
});
var wrongInput = function () {
return $("#textInput").val() != iNumber;
};
$("#textInput").bind('input', function () {
$("#btnSubmit").prop('disabled', wrongInput);
});
});
function submitForm() {
$('#booking_form').validate({
rules: {
name: { required: true, minlength: 2 },
subject: { required: true, minlength: 4 },
email: { required: true, email: true },
mobile: { required: true, minlength: 10, maxlength: 10, number: true },
stratdestination: { required: true, minlength: 2 },
endDestination: { required: true, minlength: 2 },
timedate: { required: true },
cartype: { required: true }
},
messages: {
name: { required: "Enter your name", minlength: "At least 2 characters" },
subject: { required: "Enter a subject", minlength: "At least 4 characters" },
email: { required: "Enter a valid email" },
mobile: { required: "Enter a valid phone number", minlength: "10 characters" },
stratdestination: { required: "Enter a start destination" },
endDestination: { required: "Enter an end destination" },
timedate: { required: "Select a date" },
cartype: { required: "Select a car type" }
},
submitHandler: function (form) {
$.ajax({
type: "POST",
url: "/Home/SendMail",
data: $(form).serialize(),
success: function (response) {
$('#sccmodl').modal('show');
$('.indxmsg').html(response);
},
error: function () {
alert("Error in sending the form.");
}
});
}
});
}
</script>
}
1. SendMail Action
Here’s the main controller action that handles the sending of the emails.
[HttpPost]
public ActionResult SendMail(string name, string email, string mobile, string subject, string timedate, string stratdestination, string endDestination, string cartype)
{
try
{
// Create mail messages
MailMessage mail = new MailMessage();
MailMessage forCustomer = new MailMessage();
SmtpClient smtpClient = new SmtpClient("mail.prakash.in", 587);
// Configure SMTP client
smtpClient.UseDefaultCredentials = false;
smtpClient.Credentials = new NetworkCredential("[email protected]", "Bmbn0Dn26g6i~kybW");
smtpClient.EnableSsl = false;
// Configure mail for service
mail.To.Add("[email protected]");
mail.Bcc.Add("[email protected]");
mail.From = new MailAddress("[email protected]", "Company Name");
mail.Subject = subject;
mail.Body = GenerateServiceMailBody(name, email, mobile, subject, timedate, stratdestination, endDestination, cartype);
mail.IsBodyHtml = true;
// Configure mail for customer
forCustomer.To.Add(email);
forCustomer.From = new MailAddress("[email protected]", "Company Name");
forCustomer.Subject = subject;
forCustomer.Body = GenerateCustomerMailBody(name);
forCustomer.IsBodyHtml = true;
// Send emails
smtpClient.Send(mail);
smtpClient.Send(forCustomer);
return Json("Mail sent successfully! Please check your mail.");
}
catch (SmtpException smtpEx)
{
// Log detailed SMTP exception
return Json($"SMTP Error: {smtpEx.Message}");
}
catch (Exception ex)
{
// Log general exception
return Json($"Error: {ex.Message}");
}
}
Explanation
SMTP Configuration: The SMTP client is configured using the SmtpClient class. In this case, it uses the mail.prakash.in the SMTP server on port 587, and the credentials for the email account are provided.
smtpClient.UseDefaultCredentials = false;
smtpClient.Credentials = new NetworkCredential("[email protected]", "Bmb~kybW");
smtpClient.EnableSsl = false;
Sending Emails to Multiple Recipients
Two separate emails are created.
- The first email is sent to the company (service email) with the customer's booking details.
- The second email is a confirmation sent to the customer.
Both emails are sent using smtpClient.Send(mail).
2. HTML Email Templates
HTML-formatted emails are used to send user-friendly content. Here, we generate two different types of email bodies: one for the service and one for the customer.
Service Email Template
private string GenerateServiceMailBody(string name, string email, string mobile, string subject, string timedate, string stratdestination, string endDestination, string cartype)
{
return "<table cellspacing='0'>" +
"<tr><td colspan='2'>Your contact person details:<br/></td></tr>" +
"<tr><td colspan='2'><b><br/> Name: " + name + " <br/> Email: " + email + " <br/> Mobile: " + mobile + " <br/> Subject: " + subject + " <br/> Booking Date: " + timedate + " <br/> Start Destination: " + stratdestination + " <br/> End Destination: " + endDestination + " <br/> Booking Car: " + cartype + " </b></td></tr>" +
"<tr><td colspan='2'><br/>Please contact above person as soon as possible.<br />Thanks!</td></tr>" +
"</table>";
}
Customer Email Template
private string GenerateCustomerMailBody(string name)
{
return "<table cellspacing='0'>" +
"<tr><td colspan='2'>Hello ,<br/></td></tr>" +
"<tr><td colspan='2'><b><br/> " + name + " </b></td></tr>" +
"<tr><td colspan='2'><br/>Thanks for your email. </td></tr>" +
"<tr><td colspan='2'><br/>Please connect with us. </td></tr>" +
"</table>";
}
The email sent to the customer confirms that their booking has been received and includes a personalized greeting.
3. Error Handling
Error handling is an essential aspect when dealing with external services like SMTP. Two exception blocks are used:
- SmtpException: Catches issues related to SMTP (e.g., wrong credentials, SMTP server down).
- Exception: A general catch block for any other issues that may arise.
catch (SmtpException smtpEx)
{
return Json($"SMTP Error: {smtpEx.Message}");
}
catch (Exception ex)
{
return Json($"Error: {ex.Message}");
}
Conclusion
This approach enables sending structured HTML emails from an ASP.NET MVC application using MailMessage and SmtpClient. You can easily customize the email templates, manage multiple recipients, and handle errors effectively.
When working with sensitive information like email credentials, it’s essential to avoid hardcoding them in the source code. Instead, store these values securely in a configuration file, like web.config, or use environment variables for improved security.
By following this structure, you can seamlessly add email functionality to your MVC applications and provide a smooth experience for both service providers and customers.