
June 24, 2024 07:09 by
Peter
Your web application's functionality can be substantially increased by integrating third-party APIs, which give it access to and usage of outside resources. We will look at how to use AJAX to incorporate a third-party API into an ASP.NET Core Razor Pages application in this article. Using a sample in which we retrieve public holidays from an API and present them in a Bootstrap table, we will illustrate this procedure.
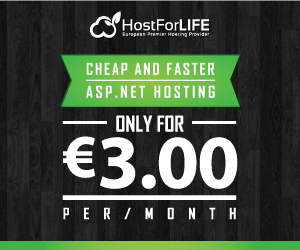
Step 1. Setting up the ASP.NET Core Razor Pages project
First, create a new ASP.NET Core Razor Pages project in Visual Studio:
- Open Visual Studio and create a new project.
- Select ASP.NET Core Web Application and click Next.
- Name your project and click Create.
- Choose NET Core" and ASP.NET Core 5.0 or later.
- Select Web Application (Model-View-Controller) and click Create.
Step 2. Creating the Razor Page
Next, add a new Razor Page to your project:
- Right-click on the Pages folder and select Add > New Item
- Choose Razor Page and name it Index.
Step 3. Designing the Razor Page
Edit the Index.cshtml file to include a form for user input and a Bootstrap table to display the fetched data.
@page
@model IndexModel
@{
ViewData["Title"] = "Home page";
}
<div class="text-center">
<h1 class="display-4">Welcome</h1>
<form id="inputForm">
<input type="text" id="yearValue" name="yearValue" placeholder="Enter Year value">
<input type="text" id="countryCode" name="countryCode" placeholder="Enter countryCode value">
<button type="submit">Submit</button>
</form>
</div>
<div class="container mt-4">
<div class="row">
<div class="col-md-12">
<table id="holidayTable" class="table table-striped">
<thead>
<tr>
<th>Date</th>
<th>Local Name</th>
<th>Name</th>
<th>Country Code</th>
<th>Fixed</th>
<th>Global</th>
<th>Types</th>
</tr>
</thead>
<tbody id="tableBody"></tbody>
</table>
</div>
</div>
</div>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script type="text/javascript">
$(document).ready(function () {
$("#inputForm").submit(function (e) {
debugger;
e.preventDefault();
var yearValue = $("#yearValue").val();
var countryCode = $("#countryCode").val();
$.ajax({
type: "GET",
url: "https://date.nager.at/api/v3/publicholidays/" + yearValue + "/" + countryCode,
success: function (data) {
console.log(data);
populateTable(data);
},
error: function (error) {
console.log(error);
}
});
});
});
function populateTable(data) {
debugger;
var tableBody = $("#tableBody");
tableBody.empty();
data.forEach(function (holiday) {
var row = $("<tr>");
row.append($("<td>").text(holiday.date));
row.append($("<td>").text(holiday.localName));
row.append($("<td>").text(holiday.name));
row.append($("<td>").text(holiday.countryCode));
row.append($("<td>").text(holiday.fixed));
row.append($("<td>").text(holiday.global));
row.append($("<td>").text(holiday.types.join(', ')));
tableBody.append(row);
});
}
</script>
Step 4. Adding jQuery and AJAX Script
In the Index.cshtml file, include jQuery, and write the AJAX call to fetch data from the third-party API. Make sure to include the security token if required.
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script type="text/javascript">
$(document).ready(function () {
$("#inputForm").submit(function (e) {
debugger;
e.preventDefault();
var yearValue = $("#yearValue").val();
var countryCode = $("#countryCode").val();
$.ajax({
type: "GET",
url: "https://date.nager.at/api/v3/publicholidays/" + yearValue + "/" + countryCode,
success: function (data) {
console.log(data);
populateTable(data);
},
error: function (error) {
console.log(error);
}
});
});
});
function populateTable(data) {
debugger;
var tableBody = $("#tableBody");
tableBody.empty();
data.forEach(function (holiday) {
var row = $("<tr>");
row.append($("<td>").text(holiday.date));
row.append($("<td>").text(holiday.localName));
row.append($("<td>").text(holiday.name));
row.append($("<td>").text(holiday.countryCode));
row.append($("<td>").text(holiday.fixed));
row.append($("<td>").text(holiday.global));
row.append($("<td>").text(holiday.types.join(', ')));
tableBody.append(row);
});
}
</script>
In this script
- The form submission is handled by preventing the default action and getting the input values.
- An AJAX GET request is made to the third-party API with the year and country code values.
- The security token is included in the request headers.
- On a successful response, the populateTable function dynamically creates rows and cells in the table to display the fetched data.
Step 5. Running the Application
- Build and run the application.
- Navigate to the /Index page.
- Enter the year and country code values and submit the form.
- The public holidays data will be fetched from the API and displayed in the Bootstrap table.
Output
\
Conclusion
Setting up the form and table on the Razor page, performing the AJAX request with the required arguments and headers, and dynamically updating the table with the retrieved data are all part of integrating a third-party API using AJAX in an ASP.NET Core Razor Pages application. Without refreshing the website, this method enables a smooth and interactive user experience.