
May 13, 2020 09:18 by
Peter
This article is an overview of FileResult in ASP.Net Core MVC. The FileResult actions are used to read and write files. FileResult is the parent of all file-related action results. There is a method on ControllerBase class called File. This method accepts a set of parameters based on the type of file and its location, which maps directly to the more specific return types. I’ll discuss how to use all the FileResult actions available in ASP.Net Core MVC.
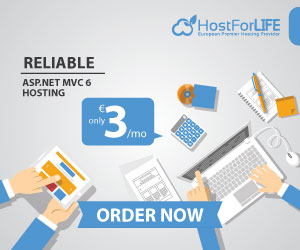
There are different type of file results in core MVC.
FileResult
FileContentResult
FileStreamResult
VirtualFileResult
PhysicalFileResult
FileResult
FileResult is the parent of all file-related action results. It is a base class that is used to send binary file content to the response. It represents an ActionResult that when executed will write a file as the response.
public FileResult DownloadFile()
{
return File("/Files/File Result.pdf", "text/plain", "File Result.pdf");
}
FileContentResult
FileContentResult is an ActionResult that when executed will write a binary file to the response.
public FileContentResult DownloadContent()
{
var myfile = System.IO.File.ReadAllBytes("wwwroot/Files/FileContentResult.pdf");
return new FileContentResult(myfile, "application/pdf");
}
FileStreamResult
FileStreamResult Sends binary content to the response by using a Stream instance when we want to return the file as a FileStream.
public FileStreamResult CreateFile()
{
var stream = new MemoryStream(Encoding.ASCII.GetBytes("Hello World"));
return new FileStreamResult(stream, new MediaTypeHeaderValue("text/plain"))
{
FileDownloadName = "test.txt"
};
}
VirtualFileResult
A FileResult that on execution writes the file specified using a virtual path to the response using mechanisms provided by the host. You can use VirtualFileResult if you want to read a file from a virtual address and return it.
public VirtualFileResult VirtualFileResult()
{
return new VirtualFileResult("/Files/PhysicalFileResult.pdf", "application/pdf");
}
PhysicalFileResult
A FileResult on execution will write a file from disk to the response using mechanisms provided by the host.You can use PhysicalFileResult to read a file from a physical address and return it, as shown in PhysicalFileResult method.
public PhysicalFileResult PhysicalFileResult()
{
return new PhysicalFileResult(_environment.ContentRootPath + "/wwwroot/Files/PhysicalFileResult.pdf", "application/pdf");
}
Step 1
Open Visual Studio 2019 and select the ASP.NET Core Web Application template and click Next.
Step 2
Name the project FileResultActionsCoreMvc_Demo and click Create.
Step 3
Select Web Application (Model-View-Controller), and then select Create. Visual Studio used the default template for the MVC project you just created.
Step 4
In Solution Explorer, right-click the wwwroot folder. Select Add > New Folder. Name the folder Files. Add some files to work with them.
Complete controller code
using Microsoft.AspNetCore.Hosting;
using Microsoft.AspNetCore.Mvc;
using Microsoft.Net.Http.Headers;
using System.IO;
using System.Text;
namespace FileResultActionsCoreMvc_Demo.Controllers
{
public class HomeController : Controller
{
private readonly IWebHostEnvironment _environment;
public HomeController(IWebHostEnvironment environment)
{
_environment = environment;
}
public IActionResult Index()
{
return View();
}
public FileResult DownloadFile()
{
return File("/Files/File Result.pdf", "text/plain", "File Result.pdf");
}
public FileContentResult DownloadContent()
{
var myfile = System.IO.File.ReadAllBytes("wwwroot/Files/FileContentResult.pdf");
return new FileContentResult(myfile, "application/pdf");
}
public FileStreamResult CreateFile()
{
var stream = new MemoryStream(Encoding.ASCII.GetBytes("Hello World"));
return new FileStreamResult(stream, new MediaTypeHeaderValue("text/plain"))
{
FileDownloadName = "test.txt"
};
}
public VirtualFileResult VirtualFileResult()
{
return new VirtualFileResult("/Files/PhysicalFileResult.pdf", "application/pdf");
}
public PhysicalFileResult PhysicalFileResult()
{
return new PhysicalFileResult(_environment.ContentRootPath + "/wwwroot/Files/PhysicalFileResult.pdf", "application/pdf");
}
}
}
Step 5
Open Index view which is in views folder under Home folder. Add the below code in Index view.
Index View
@{
ViewData["Title"] = "Home Page";
}
<h3 class="text-center text-uppercase">FileResult Action in core mvc</h3>
<ul class="list-group list-group-horizontal">
<li class="list-group-item"><a asp-action="DownloadFile" asp-controller="Home">File Result</a></li>
<li class="list-group-item"><a asp-action="DownloadContent" asp-controller="Home" target="_blank">File Content Result</a></li>
<li class="list-group-item"><a asp-action="CreateFile" asp-controller="Home">File Stream Result</a></li>
<li class="list-group-item"><a asp-action="VirtualFileResult" asp-controller="Home" target="_blank">Virtual File Result</a></li>
<li class="list-group-item"><a asp-action="PhysicalFileResult" asp-controller="Home" target="_blank">Physical File Result</a></li>
</ul>
Step 6
Build and run your Project ctrl+F5