
May 31, 2024 09:09 by
Peter
In ASP.NET MVC applications, partial views are often used to simplify and modularize the user experience. On the other hand, there are times when communication between partial views and their parent views might be challenging, particularly when transmitting values or data. This article explores effective ways to transfer values from partial views to their parent views in ASP.NET MVC, hence enhancing interactivity and user experience.
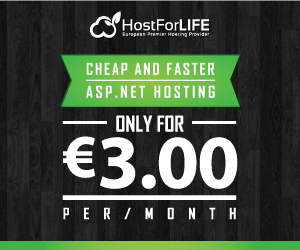
What is partial views?
Partial views, denoted by the _ prefix convention in ASP.NET MVC, are reusable components that allow developers to encapsulate specific pieces of HTML and Razor code. These partial views can be shown inside of parent views through modularization and code reuse, which promotes a more structured and manageable codebase.
Challenges in passing values
Passing values or data from a partial view back to its parent view is a typical requirement in web development. This can be challenging since partial views are divided and do not offer direct access to the components or features of the parent view. However, there are several approaches that can break through this barrier and allow parent views and partial views to communicate with each other without difficulty.
Example
Let's look at an example where there is a form input field in a partial view, and the value entered has to be sent back to the parent view for processing.
Partial view (_MyPartialView.cshtml)
<div>
<input type="text" id="inputValue" />
</div>
<script>
var value = document.getElementById('inputValue').value;
window.parent.updateMainView(value);
</script>
Parent view (Index.cshtml)
<h2>Main View</h2>
<div id="displayValue"></div>
<script>
function updateMainView(value) {
document.getElementById('displayValue').innerText = "Value from Partial View: " + value;
}
</script>
Conclusion
In ASP.NET MVC development, variables from partial views must be passed to parent views in order to improve user experience and interactivity. Using JavaScript messaging or direct function invocation, developers can establish seamless communication channels across views, enabling dynamic data interchange and interactive user interfaces. By learning these techniques, developers may produce more dependable and engaging ASP.NET MVC web applications.