
August 27, 2024 07:47 by
Peter
Keeping resumes organized across several job vacancies is essential in the recruitment business. This blog post explores the effective mapping of resumes to various job listings within a.NET MVC application. We'll go over the structure of the database, the reasoning needed to link resumes to job IDs, and the procedures to make sure that resumes associated with closed jobs are removed from consideration for open positions.
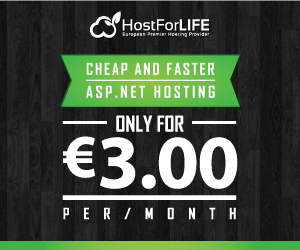
With the help of this guide, you will be able to optimize your job and resume management process in.NET MVC, regardless of whether you're creating a recruiting portal or improving existing HR administration system.
Here's how to approach your.NET MVC project to meet the requirements.
1. Database Design
- Job Table
- JobID (Primary Key)
- JobStatus (e.g., Active, Closed)
- Resume Table
- ResumeID (Primary Key)
- Other resume details.
- JobResumeMapping Table
- JobID (Foreign Key to Job Table)
- ResumeID (Foreign Key to Resume Table)
- MappingID (Primary Key for the table)
2. Mapping Resumes to Different Job IDs
You would create or update records in the JobResumeMapping table to map resumes to different jobs.
Example method to map a resume to a job.
public void MapResumeToJob(int jobId, int resumeId)
{
using (var context = new YourDbContext())
{
var mapping = new JobResumeMapping
{
JobID = jobId,
ResumeID = resumeId
};
context.JobResumeMappings.Add(mapping);
context.SaveChanges();
}
}
3. Preventing Resume from Showing in Other Jobs once the Job is closed
You should filter out resumes mapped to closed jobs.
Example of how to do it in a query.
var openJobsWithResumes = context.JobResumeMappings
.Where(jrm => jrm.Job.JobStatus == "Active")
.Include(jrm => jrm.Resume)
.ToList();
Alternatively, you can use a method to update the status of the job and remove the mapping.
public void CloseJob(int jobId)
{
using (var context = new YourDbContext())
{
var job = context.Jobs.Find(jobId);
if (job != null)
{
job.JobStatus = "Closed";
context.SaveChanges();
}
}
}
4. Ensure UI Logic
In your view, only show resumes linked to jobs that are not closed.
@foreach (var job in Model.Jobs)
{
if (job.JobStatus == "Active")
{
// Display resumes mapped to this job
}
}
5. Additional Considerations
To preserve referential integrity, you could also choose to use update operations or cascade deletes. The mappings must to be updated or eliminated in the event that a job is deleted.
6. Business Logic in the Controller
Make sure the logic for closing jobs and filtering resumes is handled by your controller methods.
Your demands for matching resumes to various jobs should be met by this configuration, which also makes sure that resumes linked to closed jobs are removed from consideration for open positions.