
December 11, 2024 06:09 by
Peter
What is a Session?
Session is KEY-VALUE structure to store the user specific data. In Asp.Net Core .NET 8 session is not enable by default, we have to enable it. Session data persists across multiple HTTP requests.
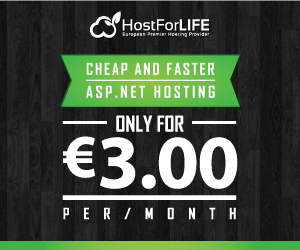
How many ways for Session storage?
In-Memory Cache: Server base storage. Storage capacity is depend upon server’s available memory. Best for single server base environment.
Distributed Cache: Session storage not on one server its store on multiple server. Distributed cache work with Redis, Sql Server etc. .
How to Enable Session in .Net 8 Asp.Net Core MVC Application?
builder.Services.AddSession();
Add another line just after app declaration line:
app.UseSession();
How to set or store the value in session?
Create or Setting Session value.
Syntax
HttpContext.Session.SetString(KEY,VALUE);
HttpContext.Session.SetInt22(KEY,VALUE);
Example
HttpContext.Session.SetString(“USERNAME”, UserName);
HttpContext.Session.SetString(“USERID”,Convert.ToString(UserID));
How to get or retrieve the value of session?
Getting Session value.
Syntax
HttpContext.Session.GetString(KEY);
HttpContext.Session.GetInt22(KEY);
Example
HttpContext.Session.GetString(“USERNAME”);
HttpContext.Session.GetString(“USERID”);
How to remove particular session key?
You can easily remove a specific session key.
Syntax
HttpContext.Session.Remove(KEY);
Example
HttpContext.Session.Remove(“UESERNAME”);
How to remove all session keys?
You can delete/remove/clear all sessions in one go.
HttpContext.Session.Clear();
Clear: It remove all the current session entries from the server.
OR
HttpContext.SignOutAsync();
SignOutAsync: It end the current user session and remove or clear the user’s authentication cookie from the server.
Walk-through on session implementation
In this article I had used Visual Studio 2022. After follow step by step you are able to implement SESSION in your Asp.Net Core project.
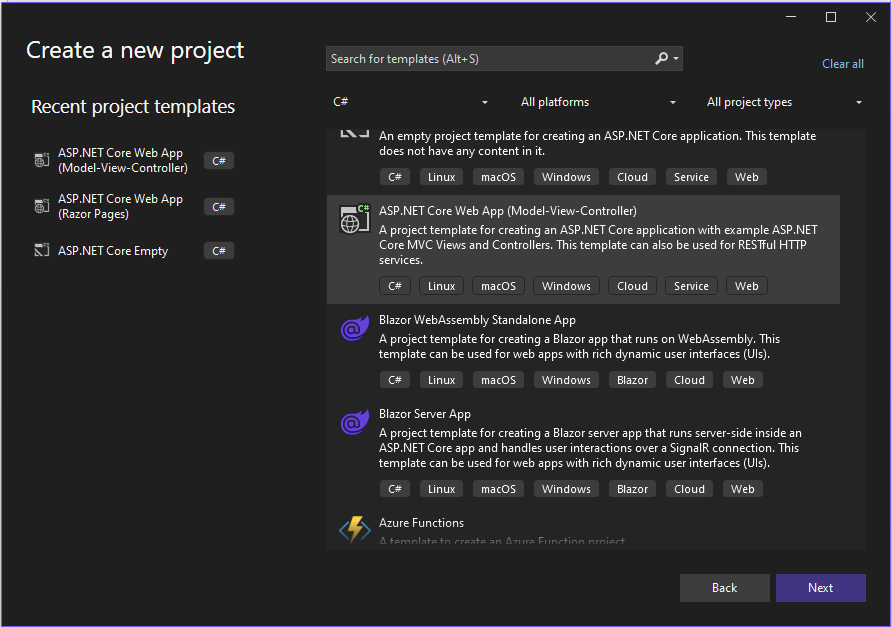
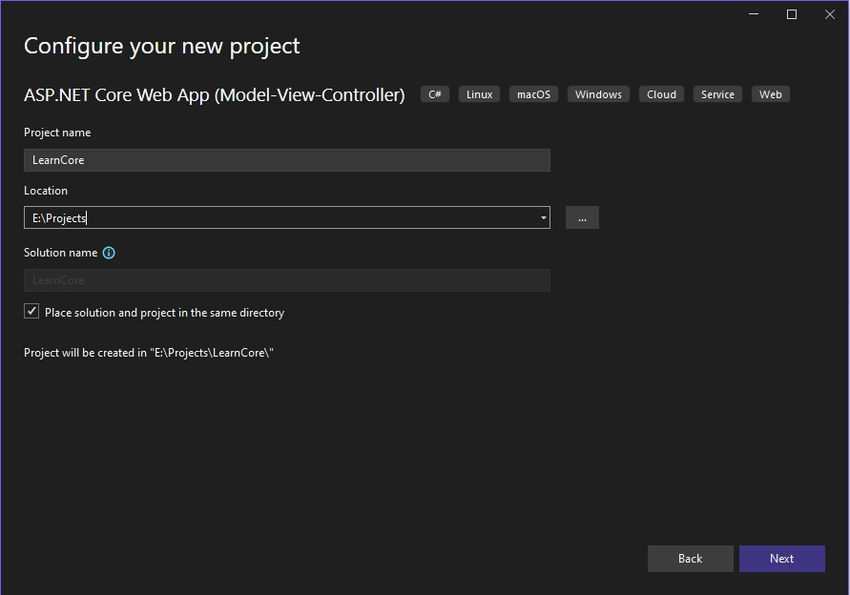
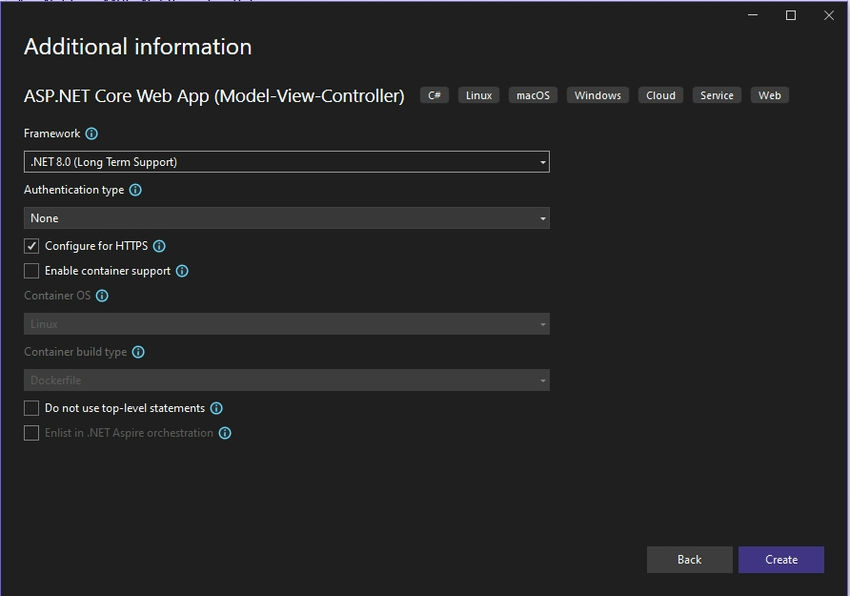
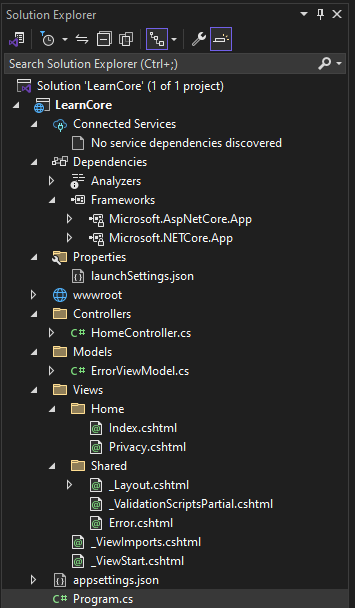
Above screenshot is a view of default project structure of Asp.Net Core .NET 8.
Now open program.cs file to add following line:
builder.Services.AddSession(); //
app.UseSession(); //after app declaration.
Update Program.cs file Code:
var builder = WebApplication.CreateBuilder(args);
// Add services to the container
builder.Services.AddControllersWithViews();
// Configure session service for the application
builder.Services.AddSession();
var app = builder.Build();
// Enable the Session Middleware
app.UseSession();
// Configure the HTTP request pipeline
if (!app.Environment.IsDevelopment())
{
app.UseExceptionHandler("/Home/Error");
// The default HSTS value is 30 days. You may want to change this for production scenarios
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseRouting();
app.UseAuthorization();
app.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
app.Run();
Open the HomeController.cs file from CONTROLLERS folder.
Update HomeController.cs file code:
using LearnCore.Models;
using Microsoft.AspNetCore.Mvc;
using System.Diagnostics;
namespace LearnCore.Controllers
{
public class HomeController : Controller
{
private readonly ILogger<HomeController> _logger;
public HomeController(ILogger<HomeController> logger)
{
_logger = logger;
}
public IActionResult Index()
{
// Create SESSION called USERNAME and store value "Peter Scott"
HttpContext.Session.SetString("USERNAME", "Peter Scott");
return View();
}
public IActionResult Privacy()
{
// Get value from SESSION
string UserValName = HttpContext.Session.GetString("USERNAME")?.ToString();
ViewBag.UserName = UserValName;
return View();
}
[ResponseCache(Duration = 0, Location = ResponseCacheLocation.None, NoStore = true)]
public IActionResult Error()
{
return View(new ErrorViewModel { RequestId = Activity.Current?.Id ?? HttpContext.TraceIdentifier });
}
}
}
Open the Privacy.cshtml file from VIEWS àHOME.
Update the Privacy.cshtml file code :
@{
ViewData["Title"] = "Privacy Policy";
}
<h1>@ViewData["Title"]</h1>
<p>Use this page to detail your site's privacy policy.</p>
<h2>
User Name: <i><u>@ViewBag.UserName</u></i>
</h2>
Now press F5 to run the project. Happy Coding!