Generally in web application, users got to register to perform some operation. Therefore every user ought to have unique username. during this tutorial we are going to see a way to check Username availability Instantly using Ajax and jQuery. First, let’s create an empty ASP.NET 6 project and then Add model classes to model folder and write the following code:
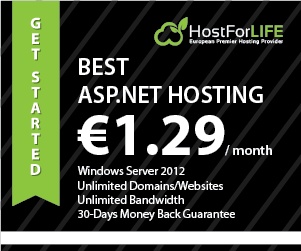
using System.ComponentModel.DataAnnotations;
namespace CheckUsernameDemo.Models
{
public class Employee
{
[Key]
public int Id { get; set; }
public string Username { get; set; }
public string Name { get; set; }
public string Title { get; set; }
}
}
Add another class
using System.Data.Entity;
namespace CheckUsernameDemo.Models
{
public class DemoContext:DbContext
{
public DbSet<Employee> Employees { get; set; }
}
}
Next step, Add a HomeController. Then an Index Action to It. Here is the code that I used:
using System.Linq;
using System.Web.Mvc;
using CheckUsernameDemo.Models;
namespace CheckUsernameDemo.Controllers
{
public class HomeController : Controller
{
DemoContext dbObj = new DemoContext();
public ActionResult Index()
{
return View();
}
}
}
Now, make a Index View and write the following code.
@model CheckUsernameDemo.Models.Employee
@{
ViewBag.Title = "Index";
}
<h2>Register Here</h2>
@using (Html.BeginForm()) {
@Html.AntiForgeryToken()
@Html.ValidationSummary()
<table>
<tr>
<td> @Html.LabelFor(m => m.Username)</td>
<td>
@Html.TextBoxFor(m => m.Username)
</td>
<td>
<label id="chkAvailable"></label>
</td>
</tr>
<tr>
<td> @Html.LabelFor(m => m.Name)</td>
<td>
@Html.TextBoxFor(m => m.Name)
</td>
<td></td>
</tr>
<tr>
<td> @Html.LabelFor(m => m.Title)</td>
<td>
@Html.TextBoxFor(m => m.Title)
</td>
<td></td>
</tr>
<tr>
<td colspan="3">
<input type="submit" value="Register" class="btnRegister"/>
</td>
</tr>
</table>
}
Now in order to examine Username accessibility Instantly we are going to Ajax call on modification Event of username Textbox. But before writing jquery code we are going to add a method in Home Controller.
public string CheckUsername(string userName)
{
string IsAvailable;
var getData = dbObj.Employees.FirstOrDefault(m => m.Username == userName.Trim());
if(getData == null)
{
IsAvailable = "Available";
}
else
{
IsAvailable = "Not Available";
}
return IsAvailable;
}
On the above code, we’ll find Employee using EntityFramework query. It will get Employee with that username then method will return Not Available otherwise Available. Now write jQuery code on change event on Username textbox.
<script type="text/javascript">
$(document).ready(function () {
$('#Username').on('change', function () {
var getName = $(this).val();
$.ajax({
type: 'POST',
contentType: 'application/json; charset=utf-8',
url: 'Home/CheckUsername',
data: "{ 'userName':' " + getName + "' }",
success: function (data) {
if (data == "Available") {
$('#chkAvailable').html('Username Is ' + data);
$('#chkAvailable').css('color', 'green');
} else {
$('#chkAvailable').html('Usernam Is ' + data);
$('#chkAvailable').css('color', 'red');
$('#Username').focus();
}
},
error: function (data) {
alert('Error in Getting Result');
}
});
});
})
</script>
With that code, we are passing the current Textbox value to Controller method to Check Username Availability. And here is the output:
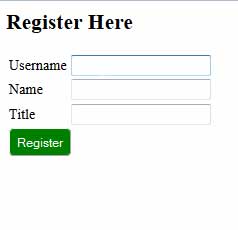
HostForLIFE.eu ASP.NET MVC 6 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
