
February 26, 2015 06:15 by
Peter
In this article I will explain you about How to use the table to apply Custom filter and pagination with angularJS in ASP.NET MVC 6. First step, you must write down the code below to your Index View.
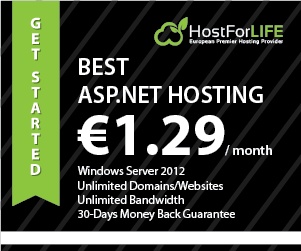
<div>
<div ng-controller="EmpList">
Select Number to show items Per Page <select ng-model="PerPageItems">
<option value="5">5</option>
<option value="10">10</option>
<option value="15">15</option>
<option value="20">20</option>
</select>
<div ng-show="filteredItems > 0">
<table class="table table-striped" id="tblEmp">
<tr>
<th>
First Name <a ng-click="orderByField='FirstName';">Sort</a>
</th>
<th>
Last Name <a ng-click="orderByField='LastName';">Sort</a>
</th>
<th>
Title <a ng-click="orderByField='Title';">Sort</a>
</th>
</tr>
<tr ng-repeat="items in empList | orderBy:orderByField | Pagestart:(currentPage-1)*PerPageItems | limitTo:PerPageItems ">
<td>
{{items.FirstName}}
</td>
<td>
{{items.LastName}}
</td>
<td>
{{items.Title}}
</td>
</tr>
</table>
</div>
<div ng-show="filteredItems > 0">
<pagination page="currentPage" total-items="filteredItems" ng-model="currentPage" items-per-page="PerPageItems"></pagination>
</div>
</div>
</div>
And now, write the below code to your JS file.
var myApp = angular.module('myApp1', ['ui.bootstrap']);
myApp.filter('Pagestart', function () { // custom filter
return function (input, start) {
if (input) {
start = +start;
return input.slice(start);
}
return [];
}
});
myApp.controller('EmpList', function ($scope, $http, $timeout) {
var EmployeesJson = $http.get("/Home/GetEmployees");
$scope.orderByField = 'FirstName';
EmployeesJson.success(function (data) {
$scope.empList = data;
$scope.filteredItems = $scope.empList.length;
$scope.totalItems = $scope.empList.length; // Total number of item in list.
$scope.PerPageItems = 5; // Intially Set 5 Items to display on page.
$scope.currentPage = 1; // Set the Intial currnet page
});
$scope.filter = function () {
$timeout(function () {
$scope.filteredItems = $scope.filtered.length;
}, 10);
};
});
Now, write the following JS Library to your View. And then, run the project. I hope this tutorial works for you!
HostForLIFE.eu ASP.NET MVC 6 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
