
November 18, 2014 08:56 by
Peter
While focusing on a project I encountered a wierd issue on my ASP.NET MVC 6. I produced an easy registration form which posted to my controller method, that obtained a view design like a parameter. Inside my method I had the need to update the values for this View Model prior to passing it to the user through a similar View. But, this really is exactly in which I encountered " strange " outcomes. Regardless of the correct data binding, correct code updating the design values, and also the correct design becoming passed straight into the view, front-end users still obtained the recent form values they initially posted.
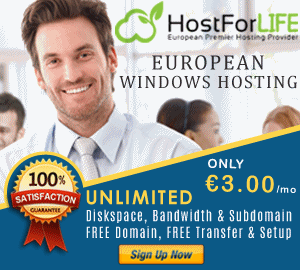
This was initially puzzling, because I can notice the model I'd been passing in was the right way up to date. Initially I assumed it was actually some type of caching issue and tried numerous choices, however to no avail. Eventually I found out the matter was coming coming from the Razor Helper I'd been utilizing - specifically the EditorFor method (though this relates to any Razor helper method).
Let us have a closer look. Below is an easy registration view :
@model posting.Controllers.Guest
<h2>Register</h2>
<p>Welcome...</p>
@using (Html.BeginForm())
{
<table>
<tr>
<td>
@Html.LabelFor(g => g.FirstName)
</td>
<td>
@Html.EditorFor(g => g.FirstName)
</td>
</tr>
<tr>
<td>
@Html.LabelFor(g => g.LastName)
</td>
<td>
@Html.EditorFor(g => g.LastName)
</td>
</tr>
<tr>
<td>
@Html.LabelFor(g => g.Highlight)
</td>
<td>
@Html.EditorFor(g => g.Highlight)
</td>
</tr>
</table>
<input type="submit" />
}
Now, I set up 2 simple controller methods to demonstrate that error. public ActionResult Register()
{
return View();
}
[HttpPost]
public ActionResult Register(Guest guest)
{
guest.FirstName = "Peter";
return View(guest);
}
The first register method is really a Get request that will returns a simple sign up form using a first name and last name. The 2nd method will be the Post version from the first methodology, which means the values our user enters directly into form are certain to get mapped to our Guest view model. For demonstration purposes, I'm changing all users' names to Peter. But, when you operate this and fill out the form, you may notice the name never comes again as Peter - it constantly comes again as no matter what the user entered. The explanation for right here is the EditorFor helper method. These helper ways retrieve their information from ModelState first, then due to model object which is passed in. ModelState is essentially metadata in regards to the current model assigned from the MVC Model Binder when a request is mapped into your controller. Therefore in case the ModelState presently has values mapped from the MVC Model Binder, Razor Helpers can use those. The answer to get this is easy.[HttpPost]
public ActionResult Register(Guest guest)
{
ModelState.Remove("FirstName");
guest.FirstName = "Peter";
return View(guest);
}
That is literally all we need to do - this'll eliminate the FirstName property value set from the default MVC Model Binder and permit our personal code to write down a whole new value. You may also clear out the complete ModelState directly, as noticed beneath :
[HttpPost]
public ActionResult Register(Guest guest)
{
ModelState.Remove("FirstName");
guest.FirstName = "Peter";
return View(guest);
}
This is a very simple issue however could be terribly frustrating in case you do not know what is happening.