This sample shows the code that is utilized to post the JSON information to the server utilizing AngularJS $http service based on AJAX POST protocol in MVC. It additionally shows the capacity of AngularJS dependency injection which is used to inject $http service to the controller as it is initiated.
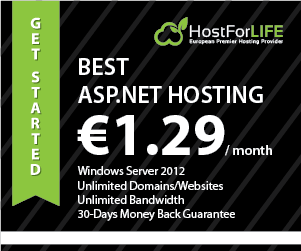
The $http administration is a center Angular administration that encourages communication with the remote HTTP servers via the browser's XMLHttpRequest object or via JSONP. The detailed article on $http service could be found on AngularJS $http page.
Pay consideration on Spring MVC controller strategy where @RequestBody is utilized. One needs to make a Domain item mapping to JSON. Additionally, simply making the space object won't do. One needs to include Jackson libraries in the classpath. Otherwise, 415 error would keep haunting.
$http Service & Related Code
Let’s write the following code to create JSON object:
var helloAjaxApp = angular.module("helloAjaxApp", []);
helloAjaxApp.controller("CompaniesCtrl", ['$scope', '$http', function($scope, $http) { $scope.companies = [
{ 'name':'Infosys Technologies',
'employees': 125000,
'headoffice': 'Bangalore'},
{ 'name':'Cognizant Technologies',
'employees': 100000,
'headoffice': 'London'},
{ 'name':'Peter',
'employees': 115000,
'headoffice': 'London'},
{ 'name':’IT Support',
'employees': 150000,
'headoffice': ' London '},
];
$scope.addRowAsyncAsJSON = function(){
$scope.companies.push({ 'name':$scope.name, 'employees': $scope.employees, 'headoffice':$scope.headoffice });
// Writing it to the server
//
var dataObj = {
name : $scope.name,
employees : $scope.employees,
headoffice : $scope.headoffice
};
var res = $http.post('/savecompany_json', dataObj);
res.success(function(data, status, headers, config) {
$scope.message = data;
});
res.error(function(data, status, headers, config) {
alert( "failure message: " + JSON.stringify({data: data}));
});
// Making the fields empty
//
$scope.name='';
$scope.employees='';
$scope.headoffice='';
};
}]);
Java Controller Code
saveCompany_JSON method which is called from $http service using POST method type.
@RequestBody Company company that represents the fact that request is parsed using Jackson library and passed as Company object.
@RequestMapping(value = "/angularjs-http-service-ajax-post-json-data-code-example", method = RequestMethod.GET)
public ModelAndView httpServicePostJSONDataExample( ModelMap model ) {
return new ModelAndView("httpservice_post_json");
} @RequestMapping(value = "/savecompany_json", method = RequestMethod.POST)
public @ResponseBody String saveCompany_JSON( @RequestBody Company company ) {
//
// Code processing the input parameters
//
return "JSON: The company name: " + company.getName() + ", Employees count: " + company.getEmployees() + ", Headoffice: " + company.getHeadoffice();
}
Java Code for Company Object (Domain object)
package com.vitalflux.core;
public class Company {
private String name;
private long employees;
private String headoffice;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Long getEmployees() {
return employees;
}
public void setEmployees(Long employees) {
this.employees = employees;
}
public String getHeadoffice() {
return headoffice;
}
public void setHeadoffice(String headoffice) {
this.headoffice = headoffice;
}
}
HostForLIFE.eu ASP.NET MVC 6 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
