Introduction: In this article, we will explore how to implement dynamic dropdown lists in an ASP.NET MVC application. We'll use jQuery AJAX to fetch data from the server and populate dependent dropdown lists based on user selections. The provided code includes examples of how to create cascading dropdowns for countries, states, and cities, allowing users to select specific locations for student data.
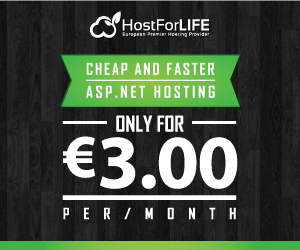
Prerequisites: To follow this tutorial, you should have a basic understanding of ASP.NET MVC, C#, jQuery, and Entity Framework.
Building the Dropdown Form
Building the Dropdown Form: In the "Index.chtml" file, we have designed a form to collect student data, including cascading dropdowns for countries, states, and cities.
<!-- Index.chtml -->
<!-- ... (existing code) ... -->
<div class="text-light text-center my-3 d-flex justify-content-center gap-3">
<div>
@if (ViewBag.Country != null)
{
@Html.DropDownListFor(x => x.Country, ViewBag.Country as SelectList, "Select Country", new { @class = "form-control" })
}
</div>
<div class="stateSection">
<span id="states-loading-progress" style="display: none;">Please wait for State..</span>
@Html.DropDownListFor(x => x.State, ViewBag.State as SelectList, "Select State", new { @class = "form-control" })
@Html.ValidationMessageFor(x => x.State)
</div>
<div class="citySection">
<span id="states-loading-progress" style="display: none;">Please wait for City..</span>
@Html.DropDownListFor(x => x.City, ViewBag.City as SelectList, "Select City", new { @class = "form-control" })
@Html.ValidationMessageFor(x => x.City)
</div>
</div>
<!-- ... (existing code) ... -->
Implementing AJAX for Dropdowns
Implementing AJAX for Dropdowns: In the JavaScript section, we've added AJAX functions to handle the country and state dropdowns dynamically.
/*------ Country Function -------*/
$("#Country").change(function () {
var stateID = $(this).val();
$('#State').empty();
$('#City').addClass('d-none');
console.log(stateID);
var statesProgress = $("#states-loading-progress");
statesProgress.show();
if (stateID > 0) {
$(".stateSection").addClass('active');
$.ajax({
type: "GET",
url: "/Home/GetStateById/" + stateID,
success: function (data) {
$("#State").html('');
var defaultOption = "<option value>Select State</option>";
$("#State").append(defaultOption);
$.each(data, function (id, option) {
var optionHtml = "<option value=" + option.StateID + ">" + option.StateName + "</option>";
$("#State").append(optionHtml);
});
statesProgress.hide();
},
error: function (xhr, ajaxOptions, thrownError) {
alert('State function failed');
statesProgress.hide();
}
});
} else {
$(".stateSection").removeClass('active');
$(".citySection").removeClass('active');
$.notify("Please select a Country", "error");
}
});
/*------ State Function -------*/
$("#State").change(function () {
$('#City').removeClass('d-none');
var cityID = $(this).val();
var statesProgress = $("#states-loading-progress");
statesProgress.show();
if (cityID > 0) {
$(".citySection").addClass('active');
$.ajax({
type: "GET",
url: "/Home/GetCityById/" + cityID,
success: function (data) {
$("#City").html('');
var defaultOption = "<option value>Select City</option>";
$("#City").append(defaultOption);
$.each(data, function (id, option) {
var optionHtml = "<option value=" + option.Id + ">" + option.CItyName + "</option>";
$("#City").append(optionHtml);
});
statesProgress.hide();
},
error: function (xhr, ajaxOptions, thrownError) {
alert('City function failed');
statesProgress.hide();
}
});
} else {
$(".citySection").removeClass('active');
$.notify("Please select a State", "error");
}
});
Handling Dropdown Requests in the Controller
Handling Dropdown Requests in the Controller: In the "HomeController," we've added action methods to retrieve country, state, and city data from the database and return them as JSON results for the AJAX requests.
// Home Controller Method
public class HomeController : Controller
{
public ActionResult Index()
{
List<CountryTable> CountryList = db.CountryTable.ToList();
ViewBag.Country = new SelectList(CountryList, "Id", "countryName");
List<StateTable> StateList = db.StateTable.ToList();
ViewBag.State = new SelectList(StateList, "StateID", "StateName");
List<CityTable> CityList = db.CityTable.ToList();
ViewBag.City = new SelectList(CityList, "Id", "CItyName");
return View();
}
public JsonResult GetStateById(int ID)
{
db.Configuration.ProxyCreationEnabled = false;
var stateList = db.StateTable.Where(x => x.CountryID == ID).ToList();
return Json(stateList, JsonRequestBehavior.AllowGet);
}
public JsonResult GetCityById(int ID)
{
db.Configuration.ProxyCreationEnabled = false;
var CityList = db.CityTable.Where(x => x.StateID == ID).ToList();
return Json(CityList, JsonRequestBehavior.AllowGet);
}
public ActionResult EditStudent(int ID)
{
var result = repo.EditFunction(ID);
// Set selected values for the dropdown lists
List <CountryTable> CountryList = db.CountryTable.ToList();
ViewBag.CountryList = new SelectList(CountryList, "Id", "countryName", result.Country);
List<StateTable> StateList = db.StateTable.ToList();
ViewBag.StateList = new SelectList(StateList, "StateID", "StateName", result.State);
List<CityTable> CityList = db.CityTable.ToList();
ViewBag.CityList = new SelectList(CityList, "Id", "CItyName", result.City);
return View(result);
}
}
}
Conclusion: In this article, we have learned how to create dynamic dropdown lists with cascading behavior in an ASP.NET MVC application using AJAX. The JavaScript AJAX functions communicate with the server to fetch data based on user selections, and the ASP.NET MVC controller methods handle the requests and return JSON results. By following this guide, you can enhance your web application with more interactive features and improve the user experience with dynamic dropdowns. Happy coding!