I have used Entity Framework to fetch the values and used Database First approach. I would also write an article on an Entity Framework but today, I would just show you how to bind the cascading dropdown list in this blog.
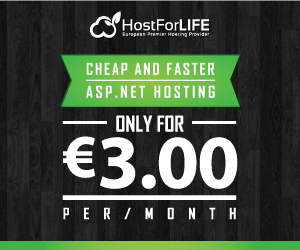
I have two dropdownlists. One is for state and the other for city. I would populate the city, which is based on the state selection. You can add as many dropdownlists, as you want. For simplicity, I am using only two dropdowns.
Create table scripts
tblState
CREATE TABLE [dbo].[tblState](
[stateid] [int] NOT NULL,
[statename] [nvarchar](50) NULL,
PRIMARY KEY CLUSTERED
(
[stateid] ASC
)WITH (PAD_INDEX = OFF, STATISTICS_NORECOMPUTE = OFF, IGNORE_DUP_KEY = OFF, ALLOW_ROW_LOCKS = ON, ALLOW_PAGE_LOCKS = ON) ON [PRIMARY]
) ON [PRIMARY]
GO
tblCity
CREATE TABLE [dbo].[tblCity](
[Cityid] [int] NOT NULL,
[CityName] [nvarchar](50) NULL,
[stateid] [int] NOT NULL
) ON [PRIMARY]
GO
ALTER TABLE [dbo].[tblCity] WITH CHECK ADD FOREIGN KEY([stateid])
REFERENCES [dbo].[tblState] ([stateid])
GO
I have tblState and tblCity, where I have stateid as Primary key in the tblState table and stateid as the Foreign key in tblCity table. You can insert states and cities from the database as you wish.
Now, with Entity Framework; create your EDMX file. In my case, I have named it ModelDemo.edmx.
Model
I have created a Model and named it Registration. This is not directly required but since I used a strongly typed View, so I have created Model. You can also add Model because if you write the httppost method to save the values to the database, then you would need the model.
public class Registration
{
[Required(ErrorMessage = "Enter State")]
public string State { get; set; }
[Required(ErrorMessage = "Enter City")]
public string City { get; set; }
}
Controller
Now, this is my Controller code.
[HttpGet]
public ActionResult Details()
{
bindState();
return View();
}
--------------------------------
public void bindState()
{
var state = objEF.tblStates.ToList();
List<SelectListItem> li = new List<SelectListItem>();
li.Add(new SelectListItem { Text = "--Select State--", Value = "0" });
foreach (var m in state)
{
li.Add(new SelectListItem { Text = m.statename, Value = m.stateid.ToString() });
ViewBag.state = li;
}
}
----------------------------------
public JsonResult getCity(int id)
{
var ddlCity = objEF.tblCities.Where(x => x.stateid == id).ToList();
List<SelectListItem> licities = new List<SelectListItem>();
licities.Add(new SelectListItem { Text = "--Select State--", Value = "0" });
if (ddlCity != null)
{
foreach (var x in ddlCity)
{
licities.Add(new SelectListItem { Text = x.CityName, Value = x.Cityid.ToString() });
}
}
return Json(new SelectList(licities, "Value", "Text", JsonRequestBehavior.AllowGet));
}
Now, if you see my Controller code, I have a httpget method Details(). I am then calling a non-action method bindState() to bind the state ddl. On selection of the state ddl, the getCity() method will be called to bind the city ddl. I have used jQuery to get the Id of the selected item and have passed it to the getCity method to get the cites for the selected state.
I have also used LINQ to get my desired select value w.r.t the Id passed.
View
Right click on action method Details(), followed by clicking Add View. I always prefer a strongly typed View and I have also created a strongly typed View in this case. The name of my View is Details.cshtml.
You need jQuery min.js. Just download it from the NuGet Package, if it’s not there.
<script src="~/Scripts/jquery-1.10.2.min.js"></script>
<script type="text/javascript">
$(document).ready(function () {
$("#State").change(function () {
$("#City").empty();
$.ajax({
type: 'POST',
url: '@Url.Action("getcity")',
dataType: 'json',
data: { id: $("#State").val() },
success: function (city) {
$.each(city, function (i, city) {
$("#City").append('<option value="'
+ city.Value + '">'
+ city.Text + '</option>');
});
},
error: function (ex) {
alert('Failed.' + ex);
}
});
return false;
})
});
</script>
I have added the jQuery-min.js. From the code given above, we get the Id of the state ddl.
<div class="form-group">
@Html.LabelFor(model => model.State, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.DropDownListFor(model => model.State, ViewBag.state as List<SelectListItem>, new { style = "width: 200px;" })
@Html.ValidationMessageFor(model => model.State, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.City, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.DropDownListFor(model => model.City, new SelectList(string.Empty, "Value", "Text"), "--Select City--", new { style = "width:200px" })
@Html.ValidationMessageFor(model => model.City, "", new { @class = "text-danger" })
</div>
I have used jQuery to get the ID of the state DDL on .change function and passed it to bind the city ddl.
Now, just run your Application and you should get the output given below and if you do not get it, then check that if you are getting any values in an Id in getCity() method. If not, then check for Id mis-match.
HostForLIFE.eu ASP.NET MVC 6 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
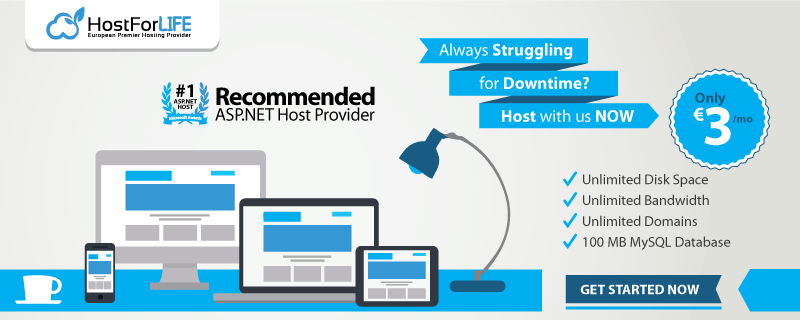