UNDERSTANDING ASP.NET MVC
ASP.NET MVC is the implementation of the MVC architecture to the programming framework. ASP.NET physically is library consisting of some assembly that can be installed on top .NET Framework 3.5. ASP.NET MVC was created as an option for developers who like MVC architecture. ASP.NET MVC did not replace Web Forms architecture that has been known and used by developers ASP.NET. The main components of ASP.NET MVC still the same as the basic architecture of MVC, the Model, View, and Controller. Some of the additional component is a web server, Routing, and dispatchers. Web server always bridging the interaction between the user with a web program. We can use the IIS web server, or a personal web server as long as the program is still in the development phase. IIS versions that can be used vary from version 5 to version 7.
ASP.NET MVC 5 Provides five different kinds of Filters. They are :
- Authentication
- Authorization
- Action
- Result
- Exception
Filters are used to inject logic at the different levels of request processing. Let us understand where at the various stages of request processing different filters get applied. Authentication Authorization filter runs after the filter and before any other filter or action method. Action filter runs before and after any action method. Result filter runs before and after execution of any action result. Exception filter runs only if action methods, filters or action results throw an exception.
An action filter consists of codes that run either before or after an action runs. It can be used for tasks like logging, privileged based authorization, authentication, caching etc. Creating a custom action filter is very easy. It can be created in four simple steps:
- Create a class
- Inherit ActionFilterAttribute class
- Override the OnActionExecuting method to run logic before the action method
- Override the OnActionExecuted method to run logic after the action method
The purpose of the action filter is to find whether a logged in user belongs to a particular privileges or not. On the basis of the result, a user will access a particular action or navigate to the login action. To do this, I have created a class and extended the ActionFilterAttribute class.
using PrivilegeDemo.Services;
using System.Web;
using System.Web.Mvc;
using Microsoft.AspNet.Identity;
using System.Web.Routing;
namespace PrivilegeDemo.Filters
{
public class AuthorizationPrivilegeFilter : ActionFilterAttribute
{
public override void OnActionExecuting(ActionExecutingContext filterContext)
{
AuthorizationService _authorizeService = new AuthorizationService();
string userId = HttpContext.Current.User.Identity.GetUserId();
if (userId != null)
{
var result = _authorizeService.CanManageUser(userId);
if (!result)
{
filterContext.Result = new RedirectToRouteResult(
new RouteValueDictionary{{ "controller", "Account" },
{ "action", "Login" }
});
}
}
else
{
filterContext.Result = new RedirectToRouteResult(
new RouteValueDictionary{{ "controller", "Account" },
{ "action", "Login" }
});
}
base.OnActionExecuting(filterContext);
}
}
}
The OnActionExecuting method is overridden because we want the code to execute before the action method gets executed. Once the action filter is created, it can be used in three ways:
- As Global filter
- As Controller
- As Action
By adding a filter to the global filter in App_Start\FilterConfig it will be available globally to the entire application.
public class FilterConfig
{
public static void RegisterGlobalFilters(GlobalFilterCollection filters)
{
filters.Add(new HandleErrorAttribute());
filters.Add(new AuthorizationPrivilegeFilter());
}
}
By adding a filter to a particular Controller it will also be available to the all actions of that particular controller.
[AuthorizationPrivilegeFilter]
public class HomeController : Controller
{
Adding a filter to a particular action it will be available to the particular action.
[AuthorizationPrivilegeFilter]
public ActionResult About()
{
HostForLIFE.eu ASP.NET MVC 5 Hosting
HostForLIFE.eu revolutionized hosting with Plesk Control Panel, a Web-based interface that provides customers with 24x7 access to their server and site configuration tools. Plesk completes requests in seconds. It is included free with each hosting account. Renowned for its comprehensive functionality - beyond other hosting control panels - and ease of use, Plesk Control Panel is available only to HostForLIFE's customers. They offer a highly redundant, carrier-class architecture, designed around the needs of shared hosting customers.
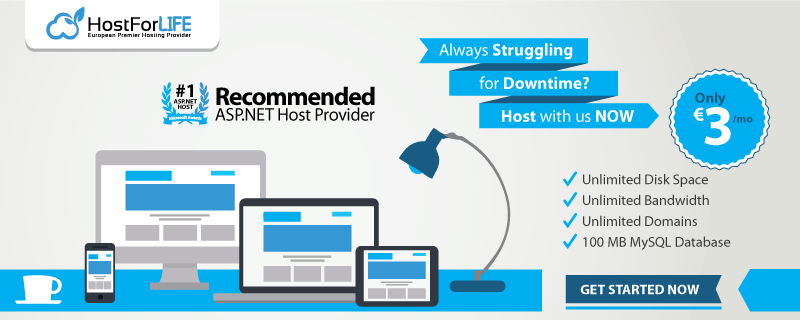