By convention, ASP.NET MVC 6 applications use HomeController to discuss with the controller that handles requests created to the root of the application. this can be designed by default with the subsequent route registration:
routes.MapRoute(
name: "Default",
url: "{controller}/{action}/{id}",
defaults: new { controller = "Home", action = "Index", id = UrlParameter.Optional }
);
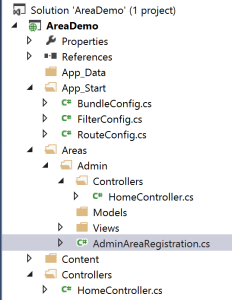
If you choose to partition your application using the ASP.NET MVC Areas feature, it’s commonplace that you simply would need calls to the root of every space to be handled employing a similar convention, via a HomeController. However, if you add a HomeController to a part (for instance, an Admin area), you'll find yourself faced with this exception:
Multiple types were found that match the controller named ‘Home’. This can happen if the route that services this request (‘{controller}/{action}/{id}’) does not specify namespaces to search for a controller that matches the request. If this is the case, register this route by calling an overload of the ‘MapRoute’ method that takes a ‘namespaces’ parameter.
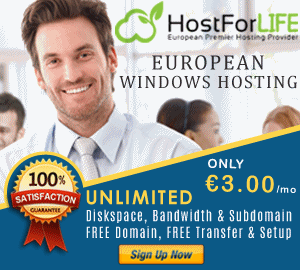
The request for ‘Home’ has found the following matching controllers:
AreaDemo.Areas.Admin.Controllers.HomeController
AreaDemo.Controllers.HomeController
Unfortunately by default you can not have duplicate controller names in ASPNET MVC Areas (or between a part and also the root of the application). fortunately, the fix for this can be pretty easy, and also the exception describes the step you wish to require. Once you’ve added a part, you may have 2 completely different places (by default) wherever routes are defined: one in your root application and one in your area registration. you may need to regulate each of them to specify a namespace parameter. the root registration will change to one thing like this:
routes.MapRoute(
name: "Default",
url: "{controller}/{action}/{id}",
defaults: new { controller = "Home", action = "Index", id = UrlParameter.Optional },
namespaces: new string[] {"AreaDemo.Controllers"}
);
Likewise, at intervals the AdminAreaRegistration.cs class, the default RegisterArea methodology feels like this:
public override void RegisterArea(AreaRegistrationContext context)
{
context.MapRoute(
"Admin_default",
"Admin/{controller}/{action}/{id}",
new { action = "Index", id = UrlParameter.Optional}
);
}
To adjust it to support a default HomeController and namespaces, it ought to be updated like so:
public override void RegisterArea(AreaRegistrationContext context)
{
context.MapRoute(
"Admin_default",
"Admin/{controller}/{action}/{id}",
new { controller="Home", action = "Index", id = UrlParameter.Optional},
new string[] {"AreaDemo.Areas.Admin.Controllers"}
);
}
With that changes in place, you must currently be ready to support the HomeController convention at intervals your MVC Areas, with duplicate controller names between areas and also the root of the applying.