With this article, I want to tell you about develop custom a HTML Helpers, thus that many of us could use inside the MVC 5 views, utilizing HTML Helpers class we will decrease the massive level of typing of HTML tags.
HTML Helpers
A HTML Helper is simply a method which returns a string. The string can represent any kinds of content that you need. As an example, you are able to use HTML Helpers to render standard HTML tags such as HTML <input> and <img> tags. You can also use HTML Helpers to render a lot of complicated content such as a tab strip or an HTML table of database data.
The listed are many of the standard HTML Helpers currently added in ASP. NET MVC :
Html.ActionLink()
Html.BeginForm()
Html.CheckBox()
Html.DropDownList()
Html.EndForm()
Html.Hidden()
Html.ListBox()
Html.Password()
Html.RadioButton()
Html.TextArea()
Html.TextBox()
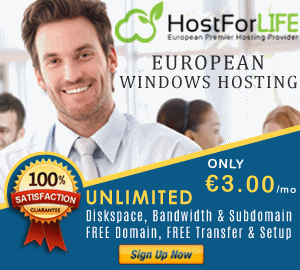
Develop a HTML Helpers with Static Methods
The easiest method to develop a new HTML Helper is to make a static method that returns a string. For example, that you decide to create some new HTML Helpers that render an HTML <Image> tag, HTML <ActionLink> tag, HTML <ActionLinkSortable> tag, HTML <DisplayAddress> tag and HTML < label> tag. And here is the example code that I used:
public static class HtmlHelpers
{
/// <summary>
/// Creates an Html helper for an Image
/// </summary>
/// <param name="helper"></param>
/// <param name="src"></param>
/// <param name="altText"></param>
/// <returns></returns>
public static MvcHtmlString Image(this HtmlHelper helper, string src, string altText)
{
var builder = new TagBuilder("img");
builder.MergeAttribute("src", src);
builder.MergeAttribute("alt", altText);
return MvcHtmlString.Create(builder.ToString(TagRenderMode.SelfClosing));
}
//create an action link that can display html
public static MvcHtmlString ActionLinkHtml(this AjaxHelper ajaxHelper, string linkText, string actionName,
string controllerName, object routeValues, AjaxOptions ajaxOptions, object htmlAttributes)
{
var repID = Guid.NewGuid().ToString();
var lnk = ajaxHelper.ActionLink(repID, actionName, controllerName, routeValues, ajaxOptions, htmlAttributes);
return MvcHtmlString.Create(lnk.ToString().Replace(repID, linkText));
}
//create an action link that can be clicked to sort and has a sorting icon (this is meant to be used to create column headers)
public static MvcHtmlString ActionLinkSortable(this HtmlHelper helper, string linkText, string actionName,
string sortField, string currentSort, object currentDesc)
{
bool desc = (currentDesc == null) ? false : Convert.ToBoolean(currentDesc);
//get link route values
var routeValues = new System.Web.Routing.RouteValueDictionary();
routeValues.Add("id", sortField);
routeValues.Add("desc", (currentSort == sortField) && !desc);
//build the tag
if (currentSort == sortField) linkText = string.Format("{0} <span class='badge'><span class='glyphicon glyphicon-sort-by-attributes{1}'></span></span>", linkText, (desc) ? "-alt" : "");
TagBuilder tagBuilder = new TagBuilder("a");
tagBuilder.InnerHtml = linkText;
//add url to the link
var urlHelper = new UrlHelper(helper.ViewContext.RequestContext);
var url = urlHelper.Action(actionName, routeValues);
tagBuilder.MergeAttribute("href", url);
//put it all together
return MvcHtmlString.Create(tagBuilder.ToString(TagRenderMode.Normal));
}
//custom html helper to output a nicely-formatted address element
public static MvcHtmlString DisplayAddressFor<TModel, TProperty>(this HtmlHelper<TModel> helper,
System.Linq.Expressions.Expression<Func<TModel, TProperty>> expression, bool isEditable = false, object htmlAttributes = null)
{
var valueGetter = expression.Compile();
var model = valueGetter(helper.ViewData.Model) as InGaugeService.Address;
var sb = new List<string>();
if (model != null)
{
if (!string.IsNullOrEmpty(model.AddressLine1)) sb.Add(model.AddressLine1);
if (!string.IsNullOrEmpty(model.AddressLine2)) sb.Add(model.AddressLine2);
if (!string.IsNullOrEmpty(model.AddressLine3)) sb.Add(model.AddressLine3);
if (!string.IsNullOrEmpty(model.City) || !string.IsNullOrEmpty(model.StateRegion) || !string.IsNullOrEmpty(model.PostalCode)) sb.Add(string.Format("{0}, {1} {2}", model.City, model.StateRegion, model.PostalCode));
if (model.IsoCountry != null) sb.Add(model.IsoCountry.CountryName);
if (model.Latitude != null || model.Longitude != null) sb.Add(string.Format("{0}, {1}", model.Latitude, model.Longitude));
}
var delimeter = (isEditable) ? Environment.NewLine : "<br />";
var addr = (isEditable) ? new TagBuilder("textarea") : new TagBuilder("address"); addr.MergeAttributes(new System.Web.Routing.RouteValueDictionary(htmlAttributes)); addr.InnerHtml = string.Join(delimeter, sb.ToArray());
return MvcHtmlString.Create(addr.ToString());
}
public static string Label(string target, string text)
{
return String.Format("<label for='{0}'>{1}</label>", target, text);
}
//Submit Button Helper
public static MvcHtmlString SubmitButton(this HtmlHelper helper, string buttonText)
{
string str = "<input type=\"submit\" value=\"" + buttonText + "\" />";
return new MvcHtmlString(str);
}
}