On this tutorial, I will show you how to create a simple API in ASP.NET MVC 5 that could return both collection of movies or one movie in Json format naturally, but depends on browser outcomes could be displayed in XML format. I targeted only on GET method during this tutorial.
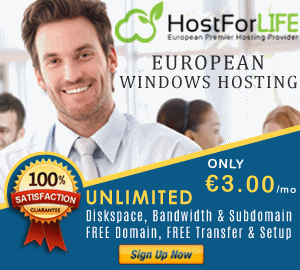
1. Let’s make a new ASP.MVC apps in Visual Studio.
2. In models create interface IMoviesRepository, class Movie and MoviesRepository.
public interface IMoviesRepository
{
IQueryable<Movie> GetAll();
Movie GetById(int id);
Movie Add(Movie movie);
bool Edit(Movie movie);
bool Remove(Guid? id);
}
public class Movie
{
public int movieId { get; set; }
public string name { get; set; }
public string releaseYear { get; set; }
}
public class MovieRepository : IMoviesRepository
{
private List<Movie> _listOfMovies = new List<Movie>();
public MovieRepository()
{
_listOfMovies.Add(new Movie {
movieId = 1,
name = "Keyboard massacre",
releaseYear = "1999" });
_listOfMovies.Add(new Movie {
movieId = 2,
name = "Keyboard massacre 2",
releaseYear = "2000" });
_listOfMovies.Add(new Movie {
movieId = 3,
name = "Keyboard massacre 3 ",
releaseYear = "2001" });
}
public IQueryable<Movie> GetAll()
{
return _listOfMovies.AsQueryable();
}
public Movie GetById(int id)
{
return _listOfMovies.Find(m => m.movieId == id);
}
public Movie Add(Movie movie)
{
throw new NotImplementedException();
}
public bool Edit(Movie movie)
{
throw new NotImplementedException();
}
public bool Remove(Guid? id)
{
throw new NotImplementedException();
}
}
Create interface first after which MovieRepository that could inherit IMoviesRepository and merely correct click on class name and apply Implement Interface.
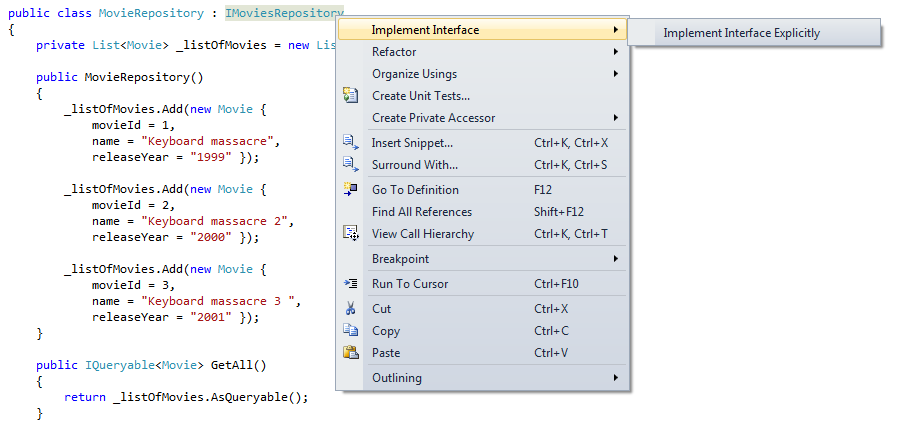
3. Then create new folder to your main project WebApiControllers and create Api Controller.Call itMoviesController.cs.
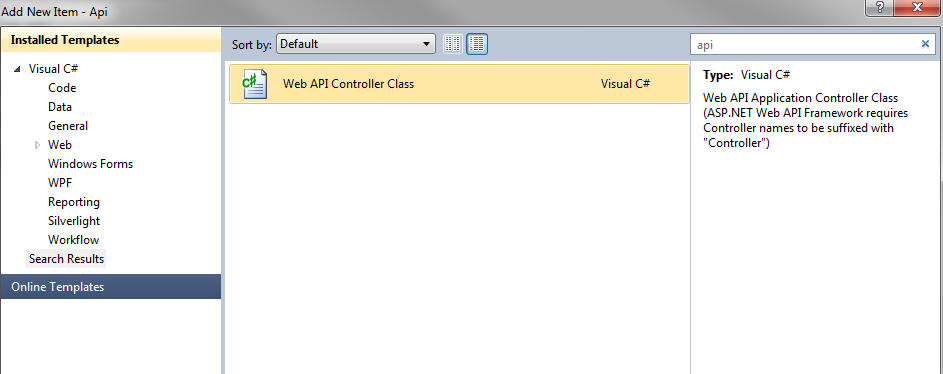
And here is the code for your controller class:
MovieRepository movieRepository;
public MoviesController()
{
this.movieRepository = new MovieRepository();
}
Calling default constructor can ensure that all repositories are set up. It's great practice to call all services that could be needed for controller - we're ensuring which our application is loose coupled. If you're acquainted with dependency injection this is very typical concept.
As I described I will be able to concentrate only on GET method, thus replace each GET methods along with custom code. You controller ought to such as this :
public class MoviesController : ApiController
{
MovieRepository movieRepository;
public MoviesController()
{
this.movieRepository = new MovieRepository();
}
// GET api/movies
public IQueryable<Movie> Get()
{
return movieRepository.GetAll();
}
// GET api/movies/5
public Movie Get(int id)
{
return movieRepository.GetById(id);
}
// POST api/movies
public void Post([FromBody]string value)
{
}
// PUT api/movies/5
public void Put(int id, [FromBody]string value)
{
}
// DELETE api/movies/5
public void Delete(int id)
{
}
}
4. Run your application now and call localhost:YourPortNumber/api/movies
You should get this results in Chrome:
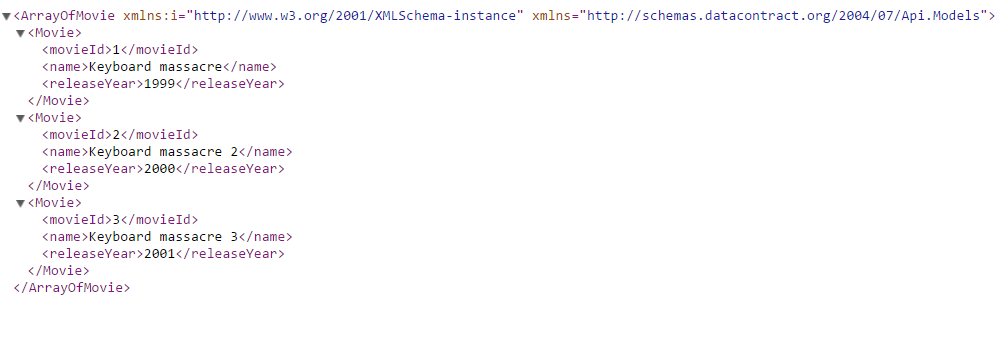
If you run it in IE you will be asked to save file. With opening it in notepad you will get this JSON results
[{"movieId":1,"name":"Keyboard massacre","releaseYear":"1999"},{"movieId":2,"name":"Keyboard massacre 2","releaseYear":"2000"},{"movieId":3,"name":"Keyboard massacre 3 ","releaseYear":"2001"}]
5. And this code below will improve GetById method. If movie can’t be found we want to return HttpResponseException.
// GET api/movies/5
public Movie Get(int id)
{
var movie = movieRepository.GetById(id);
if (movie == null)
{
throw new HttpResponseException(HttpStatusCode.NotFound);
}
return movie;
}