
October 21, 2014 11:34 by
Peter
We can do Regular Expression or REGEX validation using Annotations in ASP.NET MVC 5. we can use "RegularExpression" attribute for validation. during this attribute we specify Regular Expression string. We can also specify our custom error messages for that we'd like to set "ErrorMessage" Property in "RegularExpression" attribute. Here is the example for this.
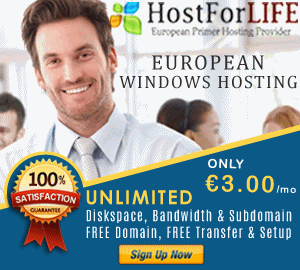
We take "Email" Address validation using REGEX. If user enter email address, which isn't in correct format at that time our validation message display on screen.
[RegularExpression(@"[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Za-z]{2,4}",
ErrorMessage="Email Address is not in proper format.")]
public string Email { get; set; }
At the view side , add this code:
@Html.EditorFor(model => model.Email)
@Html.ValidationMessageFor(model => model.Email,"", new { style="color:red"})
Here is Code for this. Model (Customer.cs):
public class Customer
{
public int CustomerID { get; set; }
[Required]
[StringLength(20,MinimumLength=2)]
public string FirstName { get; set; }
[StringLength(10)]
public string LastName { get; set; }
[RegularExpression(@"[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Za-z]{2,4}",
ErrorMessage="Email Address is not in proper format.")]
public string Email { get; set; }
}