In the RavenDB mailing list, How to combine the standard unit of work pattern of working with RavenDB in MVC applications with async. In particular, the problematic code was:
public class HomeController : Controller
{
public IAsyncDocumentSession Db { get; set; }
public async Task<ActionResult> Index()
{
var person = new Person {Name = "Khalid Abuhakmeh"};
await Db.StoreAsync(person);
return View(person);
}
protected override void OnActionExecuting(ActionExecutingContext filterContext)
{
Db = MvcApplication.DocumentStore.OpenAsyncSession();
base.OnActionExecuting(filterContext);
}
protected override void OnActionExecuted(ActionExecutedContext filterContext)
{
Db.SaveChangesAsync()
.ContinueWith(x => { });
base.OnActionExecuted(filterContext);
}
lic class Person
{
public string Id { get; set; }
public string Name { get; set; }
}
}
As you probably noticed, the problem Db.SaveChangesAsync(). We want to execute the save changes in an async manner, but we don’t want to do that in a way that would block the thread. The current code just assume the happy path, and any error would be ignored. That ain’t right. If we were using Web API, this would be trivially easy, but we aren’t. So let us see what can be done about it.
I created a new MVC 4 application and wrote the following code:
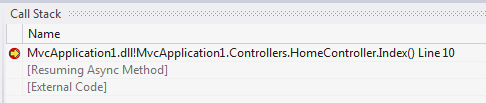
As you can see, I have a break point after the await, which means that when that break point is hit, I’ll be able to see what is responsible for handling async calls in MVC4. When the breakpoint was hit, I looked at the call stack, and saw:
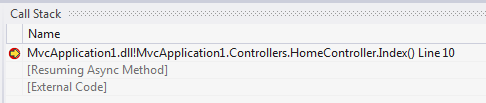
Not very useful, right? But we can fix that:
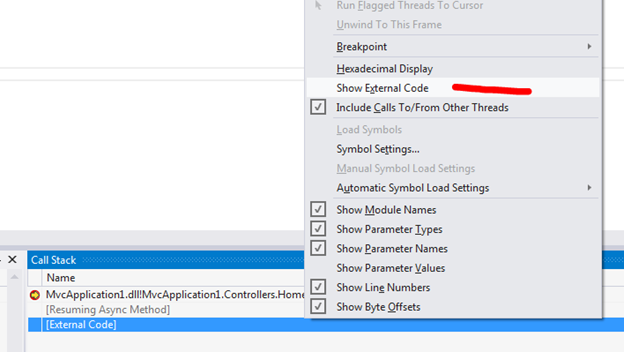
And now we get:
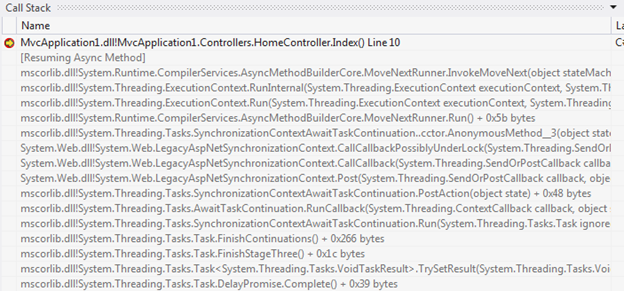
This is a whole bunch of stuff that doesn’t really help, I am afraid. But then I thought about putting the breakpoint before the await, which gave me:
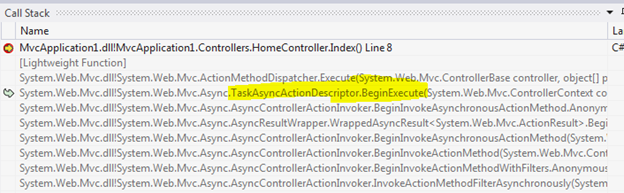
And this means that I can check the code here. I got the code and started digging. At first I thought that I couldn’t do it, but then I discovered that I could. See, all you have to do is to create you own async action invoker, like so:
public class UnitOfWorkAsyncActionInvoker : AsyncControllerActionInvoker
{
protected override IAsyncResult BeginInvokeActionMethod(
ControllerContext controllerContext,
ActionDescriptor actionDescriptor,
IDictionary<string, object> parameters, AsyncCallback callback,
object state)
{
return base.BeginInvokeActionMethod(controllerContext, actionDescriptor, parameters,
result => DoSomethingAsyncAfterTask().ContinueWith(task => callback(task)),
state);
}
public async Task DoSomethingAsyncAfterTask()
{
await Task.Delay(1000);
}
}
And then register it :
DependencyResolver.SetResolver(type =>
{
if (type == typeof (IAsyncActionInvoker))
return new UnitOfWorkAsyncActionInvoker();
return null;
}, type => Enumerable.Empty<object>());
Note: Except for doing a minimum of F5 in the debugger, I have neither tested nor verified this code. It appears to do what I want it to, and since I am only getting to this because a customer asked about this in the mailing list, that is about as much investigation time that I can dedicate to it.