
December 19, 2014 06:19 by
Peter
Now, with this article I am going to create random 15 characters long authorization number in ASP.NET MVC 6. This can be used for several completely different purposes in your MVC application like unique barcodes, authorization codes etc. to make thinks more fascinating we are getting to force the method to place letter before and after random code. GenerateLetter method is responsible to provide us only letter. I used this code based on small tutorial.
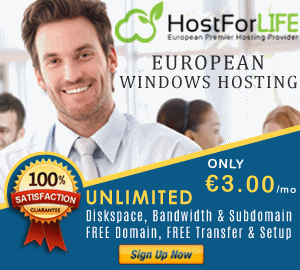
Controller
public char GenerateLetter()
{
Random randomNumber = new Random();
int number = randomNumber.Next(0, 26);
char letter = (char)('a' + number);
return letter;
}
public string GenerateAuthCode()
{
bool codeExists = false;
string code = GenerateLetter().ToString();
do
{
code += Guid.NewGuid().ToString("N").Substring(0, 13);
code += GenerateLetter().ToString();
YourDBContext dbContext = new YourDBContext();
var Exists = dbContext.products.FirstOrDefault(m => m.barcode == code);
codeExists = Exists == null? false : true;
}
while (codeExists);
return code;
}
I am calling GenerateLetter method twice before & after random 13 characters code is generated. I also want to make sure that code is always unique so I am calling Database to find if any product already have this barcode, if so my method will repeat whole process.