In this tutorial, I will show you how to use tag helper in ASP.NET 5 MVC 6. A Tag Helper is just another c-sharp class that inherits from the abstract Microsoft.AspNet.Razor.TagHelpers.TagHelper class. This abstract class contains two virtual methods for you: Process and ProcessAsync.
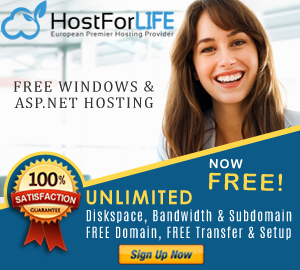
Adding Dependency
To be able to use MVC and his new feature called Tag Helper, we need to add some dependencies. Open the file and add 3 pieces project.json library
"dependencies": {
"Microsoft.AspNet.Server.IIS": "1.0.0-beta5",
"Microsoft.AspNet.Server.WebListener": "1.0.0-beta5",
"Microsoft.AspNet.Mvc": "6.0.0-beta5",
"Microsoft.AspNet.Mvc.TagHelpers": "6.0.0-beta5",
"Microsoft.AspNet.Tooling.Razor" : "1.0.0-beta5"
},
Library Microsoft.AspNet.Mvc "used to be able to use MVC and Microsoft.AspNet.Mvc.TagHelpers and Microsoft.AspNet.Tooling.Razor library in order to use the Tag Helper.
Creating Folders
Create a new folder in the root of some of the project for the benefit of our MVC. There are 4 folders created are Models, Views, Controllers and Repository. Repository Folder optional course here, only I use to put the class file repository.
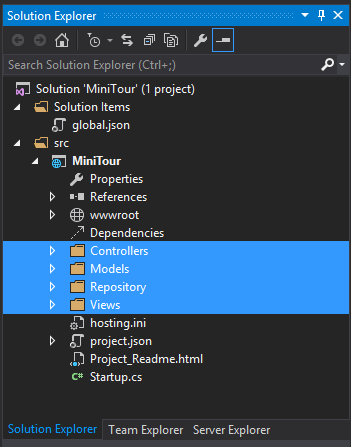
Startup configuration
We are ready to perform the configuration in Startup.cs so dependency has been added. For the purposes of configuration, MVC needs to be added to the DI Container and to the pipeline. Therefore, the configuration will occur in the second method.
To register MVC to DI Container is easy, see the following code
public void ConfigureServices(IServiceCollection services)
{
services.AddMvc();
}
As for the register to the pipeline and conduct the default configuration routing is below
public void Configure(IApplicationBuilder app)
{
app.UseMvc(route => {
route.MapRoute("Default", "{Controller=Home}/{Action=Index}/{Id:int?}");
});
}
Unlike earlier where we use an anonymous object in the third parameter to provide a default value in the template, now simply by using the = sign on the template that is the default value.
Else instead of using the fourth parameter to constraints in the form of regex we can directly use them in the template. In the example above there at {id: int?}. int id only intended to be integers.
The question mark (?) Means that the id is optional, there may be no.
MVC in Action
Until this stage, the configuration for MVC is ready and live Model, View and Controller as required. Example I had Controller as under
public class HomeController : Controller
{
private StudentRepository _studentRepository = new StudentRepository();
public IActionResult Index()
{
var students = _studentRepository.Get();
return View(students);
}
public IActionResult Get(int id)
{
var student = _studentRepository.Get(id);
return View(student);
}
}
Now let's make her view by utilizing the new features that the Tag Helper.
At the root folder View create a MVC View Page with name Import _ViewImports.cshtml.
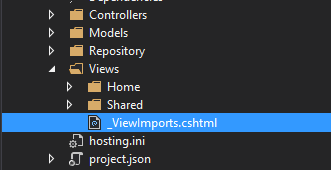
And enter the following line in it to be able to use the Tag Helper on all pages view.
@addTagHelper "*, Microsoft.AspNet.Mvc.TagHelpers"
I'll give you an example :
@{
ViewBag.Title = "Home Page";
Layout = "_Layout";
}
@using MiniTour.Models
@model List<Student>
<h3>Student List</h3>
<hr/>
<table>
<tr>
<th>First Name</th>
<th>LastName</th>
<th></th>
</tr>
@foreach (var s in Model)
{
<tr>
<td>@s.FirstName</td>
<td>@s.LastName</td>
<td>@Html.ActionLink("Detail","Get","Home",new { id=s.Id}) |
<a asp-controller="Home" asp-action="Get" asp-route-id="@s.Id">Detail</a></td>
</tr>
}
</table>
HostForLIFE.eu ASP.NET MVC 6 Hosting
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
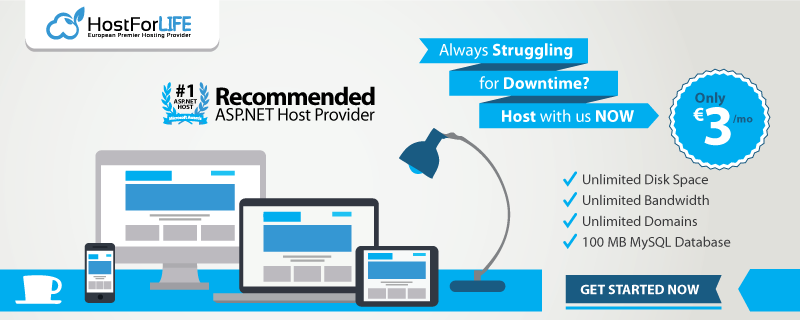